In the domain of computer programming, assignments serve as essential tools for learning, evaluating technical skills, and assessing problem-solving capabilities. Whether you're starting your programming journey or facing complex tasks as an experienced coder, mastering the approach to advanced programming assignments is crucial for achieving success. This guide aims to provide a comprehensive framework that can be universally applied, offering a structured methodology and practical examples to effectively elucidate each step.
Programming assignments go beyond mere coding exercises; they are opportunities to cultivate critical thinking, refine problem-solving strategies, and deepen understanding of algorithms and data structures. For students, these assignments foster growth and proficiency by applying theoretical knowledge to practical scenarios. For professionals, they offer chances to refine skills, explore new techniques, and deliver solutions that meet stringent requirements.
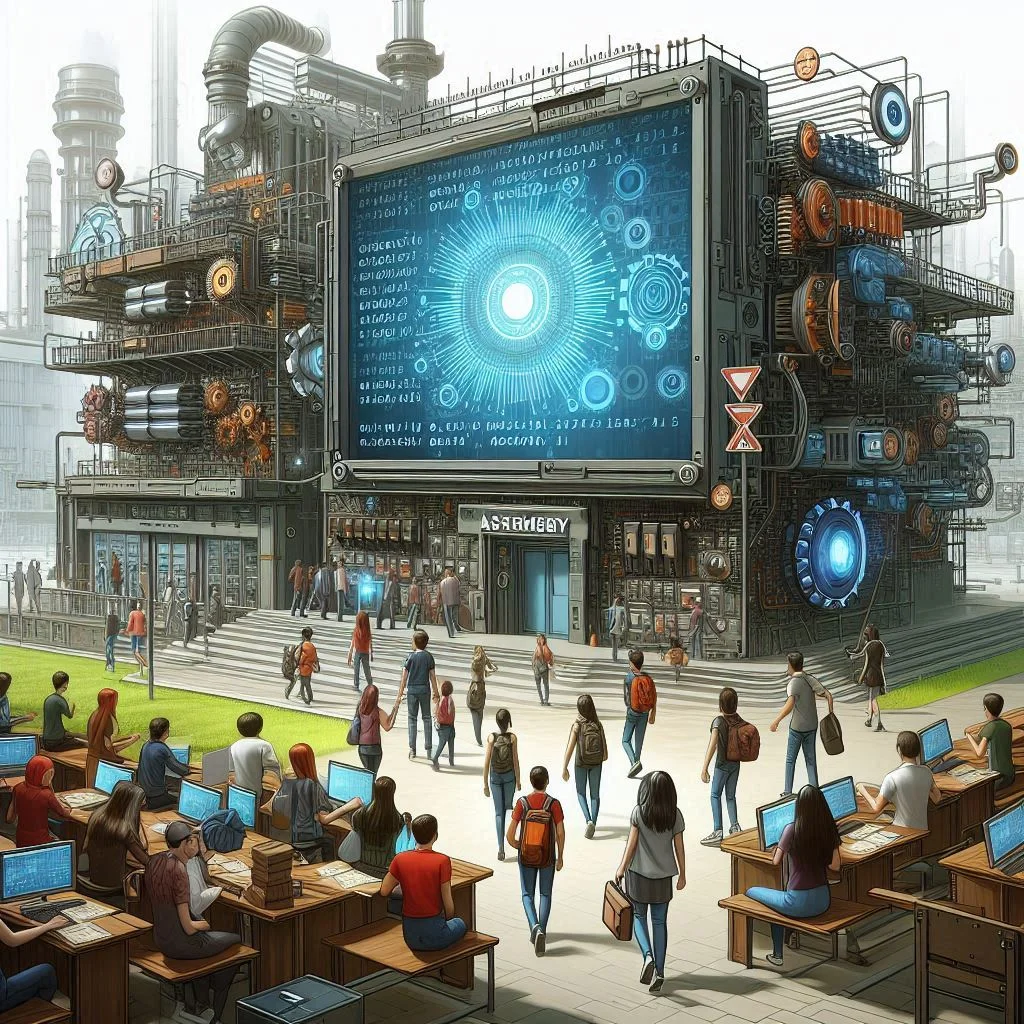
Successfully navigating these assignments requires not only technical prowess but also systematic planning and disciplined execution. By breaking down complex problems into manageable components, understanding specific requirements, and leveraging appropriate resources, programmers can approach tasks methodically. This systematic approach not only enhances efficiency but also ensures clarity in design and implementation, crucial for producing robust and maintainable code.
Skills such as problem dissection, effective research, and clear documentation transcend individual assignments; they are foundational to successful programming careers. This guide aims to equip readers with the tools and mind-set necessary to confidently tackle any programming challenge, including how to solve your assembly language assignments using C++ seamlessly and empowering them to excel in both academic and professional settings.
Understanding the Assignment Requirements
Before diving into any coding, it's vital to grasp the assignment requirements fully. This initial step sets the foundation for your entire approach. Take the time to read through the specifications carefully, noting key components such as required functions, input/output expectations, and any specific constraints or guidelines provided.
For instance, consider an assignment that involves implementing various assembly language functions, as described below:
- Void function, gnomeSort(): Sort numbers into ascending order using the gnome sort algorithm.
- Void function, basicStats(): Calculate statistical metrics (minimum, median, maximum, sum, average) for a list of numbers, calling listMedian() for median calculation.
- Value returning integer function, listMedian(): Compute and return the median of a sorted list of numbers.
- Value returning double function, corrCoefficient(): Calculate the correlation coefficient between two datasets.
- Function readB13Num(): Read an ASCII base-13 number from user input, convert it to an integer, and handle various error conditions.
Understanding these requirements upfront ensures you don't miss critical details and helps you plan your implementation strategy effectively.
Break Down the Problem
Once you've understood the requirements, the next step is to break down the assignment into manageable parts or sub-tasks. This decomposition process is crucial for tackling complexity and maintaining clarity throughout your implementation.
Using our example assignment:
- Identify Functions: List out the functions you need to implement based on the requirements (gnomeSort, basicStats, listMedian, corrCoefficient, readB13Num).
- Understand Function Interdependencies: Recognize how functions might interact or depend on each other (e.g., basicStats depending on listMedian for median calculation).
- Define Inputs and Outputs: Clarify what inputs each function will require and what outputs they should produce.
- Consider Edge Cases: Anticipate special cases or edge scenarios (e.g., empty lists, maximum input lengths) that may require specific handling.
Breaking down the problem helps in organizing your thoughts and provides a clear roadmap for implementation.
Research and Gather Resources
Programming assignments often require familiarity with algorithms, data structures, and sometimes, specific programming languages or assembly instructions. Researching unfamiliar concepts or functions is essential to ensure accuracy and efficiency in your implementation.
For our assembly language assignment, consider these research points:
- Algorithm Understanding: Gain a deeper understanding of the gnome sort algorithm for efficient sorting.
- Statistical Calculations: Review statistical formulas for calculating minimum, maximum, sum, average, and median.
- Assembly Language Specifics: Brush up on assembly language syntax, memory management, and function calling conventions relevant to your platform (e.g., Ubuntu with yams).
Utilize resources such as official documentation, textbooks, online tutorials, and reputable programming forums to supplement your understanding.
Designing the Solution
With a clear understanding of the requirements and necessary background knowledge, proceed to design your solution. Effective design focuses on creating functions that are modular, well-structured, and meet the assignment specifications.
- Modular Function Design: Plan each function's scope, inputs, outputs, and internal logic.
- Use of Stack for Local Variables: Ensure proper use of stack memory for storing local variables (avoiding static variables as per assignment requirements).
- Integration with Main Program: Consider how your assembly file will integrate with the main program that calls these functions.
For instance, sketch out pseudo code or flowcharts to outline the logic flow of each function before diving into actual coding. This step helps in visualizing the implementation and catching potential issues early on.
Implementing Functions in Assembly
Now comes the hands-on part: implementing each function in assembly language. This step-by-step process involves translating your design into actual code while adhering strictly to the assignment guidelines and best practices.
gnomeSort() Function Implementation:
; Function: gnomeSort
; Description: Sorts an array of numbers in ascending order using gnome sort algorithm
; Inputs:
; - Array of numbers (passed via registers or stack)
; Outputs:
; - Sorted array (in place)
; Notes:
; - Uses stack for local variables, no static variables allowed
gnomeSort:
; Implementation details go here
; Use stack for local variables
; Implement gnome sort logic
; Return sorted array
basicStats() Function Implementation:
; Function: basicStats
; Description: Computes minimum, median, maximum, sum, and average for a list of numbers
; Inputs:
; - Array of numbers (passed via registers or stack)
; Outputs:
; - Minimum, median, maximum, sum, and average values (returned or via reference)
; Notes:
; - Calls listMedian() function for median calculation
; - Uses stack for local variables, no static variables allowed
basicStats:
; Implementation details go here
; Use stack for local variables
; Compute min, max, sum, average
; Call listMedian() for median calculation
; Return or set values
Continue this pattern for listMedian(), corrCoefficient(), and readB13Num() functions, ensuring each function is implemented correctly according to its specific requirements and using appropriate assembly language instructions.
Testing and Debugging
Once you've implemented your functions, it's crucial to test them rigorously to ensure they work as intended across various scenarios and inputs. Testing should cover:
- Normal Use Cases: Test with typical inputs to verify correct functionality (e.g., sorting numbers, calculating statistics).
- Edge Cases: Test with edge scenarios (e.g., empty lists, maximum input lengths) to ensure robustness.
- Error Handling: Validate error handling mechanisms (e.g., invalid inputs, out-of-range values) as specified in the assignment.
Use debuggers, print statements, or logging techniques to identify and resolve any issues or unexpected behaviors in your code.
Documenting Your Code
Clear and concise documentation is essential for understanding your codebase, especially in programming assignments where clarity and adherence to specifications are paramount. Document each function within your assembly file with:
- Function Purpose: Describe what each function does and its expected behavior.
- Parameters and Return Values: Specify input parameters and how results are returned (registers, stack, etc.).
- Special Considerations: Note any specific instructions or constraints (e.g., stack usage, no static variables).
Here's an example documentation format for basicStats() function:
; Function: basicStats
; Description: Computes minimum, median, maximum, sum, and average for a list of numbers
; Inputs:
; - Array of numbers (passed via registers or stack)
; Outputs:
; - Minimum, median, maximum, sum, and average values (returned or via reference)
; Notes:
; - Calls listMedian() function for median calculation
; - Uses stack for local variables, no static variables allowed
ign: justify; ">; - Uses stack for local variables, no static variables allowed
Ensure that your documentation is in line with your code and provides sufficient detail for someone else (or your future self) to understand and maintain the codebase.
Conclusion
Achieving mastery in complex programming assignments demands a methodical approach that includes comprehending requirements, meticulous planning, thorough research, effective design, precise implementation, rigorous testing, and comprehensive documentation. By adhering to the steps delineated in this guide and implementing them consistently, you will enhance your ability to confront programming challenges with confidence and proficiency. Each assignment serves as an opportunity not only to sharpen technical skills but also to foster critical thinking and problem-solving capabilities essential for success in programming careers. Embrace these principles, continue to explore new techniques, and maintain a commitment to excellence in both academic and professional endeavors. With dedication and practice, you can navigate the intricacies of programming assignments adeptly and achieve your goals effectively.