In this comprehensive guide, we'll explore the step-by-step process of implementing a Covid Data Tracker using hash tables to solve your C++ Assignment. This project serves not only to fulfill specific assignment requirements but also to develop crucial skills in utilizing the STL (Standard Template Library), managing file operations, implementing hash tables, and efficiently handling data structures.
Implementing a Covid Data Tracker involves designing a robust CovidDB class that supports essential operations like adding, retrieving, and removing data entries. This class encapsulates each data entry using the DataEntry class, which stores details such as the date, country name, cumulative cases (c_cases), and cumulative deaths (c_deaths). By structuring our implementation around these classes, we ensure clarity and maintainability in our codebase.
Central to our implementation is the hash table itself, which utilizes the STL's vector and list containers. We employ separate chaining to handle collisions, ensuring efficient storage and retrieval of data entries. A well-defined hash function maps country names to indices within the table, facilitating quick access and manipulation of data.
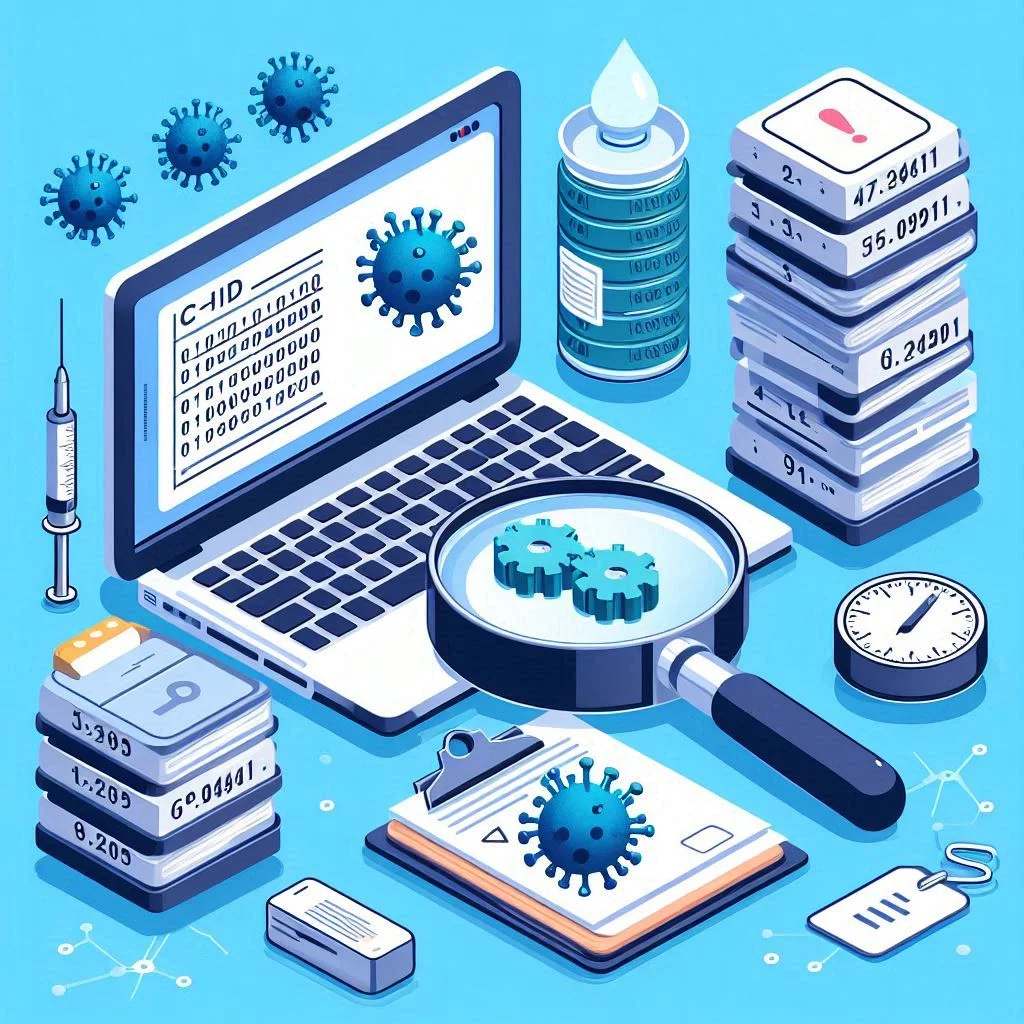
File operations play a critical role in this project, particularly in loading initial data from a CSV file (WHO-COVID-Data.csv). This file contains detailed Covid-19 statistics, which are parsed and inserted into the hash table during initialization. The implementation includes functions to read, parse, and validate data from this file, ensuring accurate representation within our data structure.
Creating a user-friendly interface is essential for practical application. Our guide includes a console-based menu system that allows users to interact seamlessly with the Covid Data Tracker. Options such as creating the initial hash table, adding new data entries, retrieving specific entries, removing entries, and displaying the current state of the hash table are implemented to provide comprehensive functionality.
Finally, thorough testing and adherence to assignment guidelines are emphasized. By rigorously testing each functionality—ensuring correct hash table operations, file loading accuracy, and interface responsiveness—students solidify their understanding and proficiency in C++ programming concepts relevant to real-world data management scenarios.
In conclusion, mastering the implementation of a Covid Data Tracker using hash tables not only prepares students for academic assignments but also equips them with foundational skills applicable across various software development domains. This guide serves as a fundamental resource for learning and applying hash table concepts in programming assignments and projects.
Understanding the Assignment Requirements
The assignment requires us to design and implement a CovidDB class that supports operations for managing Covid-19 data entries using hash tables. Here’s a breakdown of the required functionalities:
- add(DataEntry entry): Insert a new data entry into the hash table.
- get(string country): Retrieve a data entry for a specific country.
- remove(string country): Remove a data entry for a specific country.
Designing the Data Structures
The foundation of our implementation starts with designing the DataEntry class. This class will encapsulate the essential information for each data entry, including date, country, cumulative cases (c_cases), and cumulative deaths (c_deaths):
#include < string >
using namespace std;
class DataEntry {
private:
string date;
string country;
int c_cases;
int c_deaths;
public:
DataEntry(string date, string country, int cases, int deaths) {
this->date = date;
this->country = country;
this->c_cases = cases;
this->c_deaths = deaths;
}
string getCountry() {
return country;
}
// Getters and setters for other attributes
};
Implementing the Hash Table
To efficiently store and retrieve data entries, we'll implement a hash table using STL’s vector and list for separate chaining. Here’s how you can set up the CovidDB class:
#include < vector >
#include < list >
#include < iostream >
#include < fstream >
class CovidDB {
private:
vector> table; // Hash table using vector of lists
// Hash function to map country names to indices in the table
int hashFunction(string country) {
// Implement your hash function here
// Example: Simple ASCII sum hash
int hash = 0;
for (char c : country) {
hash += c;
}
return hash % table.size(); // Modulo to fit within table size
}
public:
CovidDB(int size) {
table.resize(size); // Initialize hash table with given size
}
bool add(DataEntry entry) {
int index = hashFunction(entry.getCountry());
table[index].push_back(entry);
return true; // Assume always successful for simplicity
}
DataEntry get(string country) {
int index = hashFunction(country);
for (auto& entry : table[index]) {
if (entry.getCountry() == country) {
return entry;
}
}
// Return a default entry or handle not found scenario
return DataEntry("", "", 0, 0);
}
void remove(string country) {
int index = hashFunction(country);
for (auto it = table[index].begin(); it != table[index].end(); ++it) {
if (it->getCountry() == country) {
table[index].erase(it);
return; // Assuming only one entry per country for simplicity
}
}
}
};
Handling File Operations
In the assignment, you'll need to load initial data from a CSV file (WHO-COVID-Data.csv). Let’s implement a function to read and parse this file to populate our hash table:
void loadFromFile(CovidDB& db, string filename) {
ifstream file(filename);
string line;
while (getline(file, line)) {
// Parse each line and create DataEntry objects
// Example parsing logic: Split by commas and populate DataEntry
// Add entries to the hash table using db.add(entry)
}
file.close();
}
Implementing Operations
To fulfill the assignment requirements, implement functions to add, retrieve, and remove data entries from the hash table. Ensure these operations handle collisions using separate chaining and manage data efficiently:
// Implementing add(), get(), and remove() within CovidDB class
// Refer back to CovidDB class implementation in section 3 for details
Creating a User Interface
Provide a user-friendly interface for interacting with the hash table. This includes options to create the initial hash table from file data, add new entries, retrieve entries, remove entries, and display the current state of the hash table:
void showMenu() {
cout << "Covid Tracker\n";
cout << "1. Create the initial hash table\n";
cout << "2. Add a new data entry\n";
cout << "3. Get a data entry\n";
cout << "4. Remove a data entry\n";
cout << "5. Display hash table\n";
cout << "0. Quit the system\n";
}
int main() {
CovidDB db(17); // Initialize hash table with size 17
int choice;
do {
showMenu();
cout << "Please choose the operation you want: ";
cin >> choice;
switch (choice) {
case 1:
loadFromFile(db, "WHO-COVID-Data.csv");
break;
case 2:
// Implement add functionality
break;
case 3:
// Implement get functionality
break;
case 4:
// Implement remove functionality
break;
case 5:
// Display current hash table
break;
case 0:
cout << "Exiting...\n";
break;
default:
cout << "Invalid choice. Please try again.\n";
}
} while (choice != 0);
return 0;
}
Testing and Submission
To ensure your implementation meets the assignment requirements, thoroughly test each functionality. Verify the hash table operations, file loading, and user interface interactions. Follow the assignment guidelines for submission, ensuring your code compiles without errors and functions as expected.
Conclusion
By following this comprehensive guide, you've acquired the knowledge and skills necessary to successfully implement a Covid Data Tracker using hash tables in C++. This project serves as more than just an academic exercise; it enhances your proficiency in essential areas of programming such as data structure management, file operations, and user interface design.
Implementing the Covid Data Tracker has equipped you with a solid understanding of hash table implementations, including handling collisions with separate chaining and optimizing data retrieval efficiency. You've learned how to integrate STL containers like vectors and lists effectively to manage large datasets and perform operations like adding, retrieving, and removing data entries.