Programming assignments are a fundamental part of computer science education, designed to assess your ability to apply theoretical knowledge to practical problems. These assignments can vary in complexity, but with a structured approach, you can tackle them effectively. This blog will guide you through a detailed process to solve programming assignments, using a generic example involving arrays and basic operations.
We'll break down the task of creating a program that allows a user to input a set of numbers, store them in an array, and then find and display the largest and smallest numbers. By following this structured approach, you'll be able to approach similar programming assignments with greater clarity and efficiency.
Understanding the problem, designing a solution with pseudocode, and creating a flowchart are key steps in the process. Implementing these steps systematically will not only help you solve the current assignment but also prepare you for tackling more complex problems in the future. This guide aims to make the process of solving programming assignments straightforward and manageable.
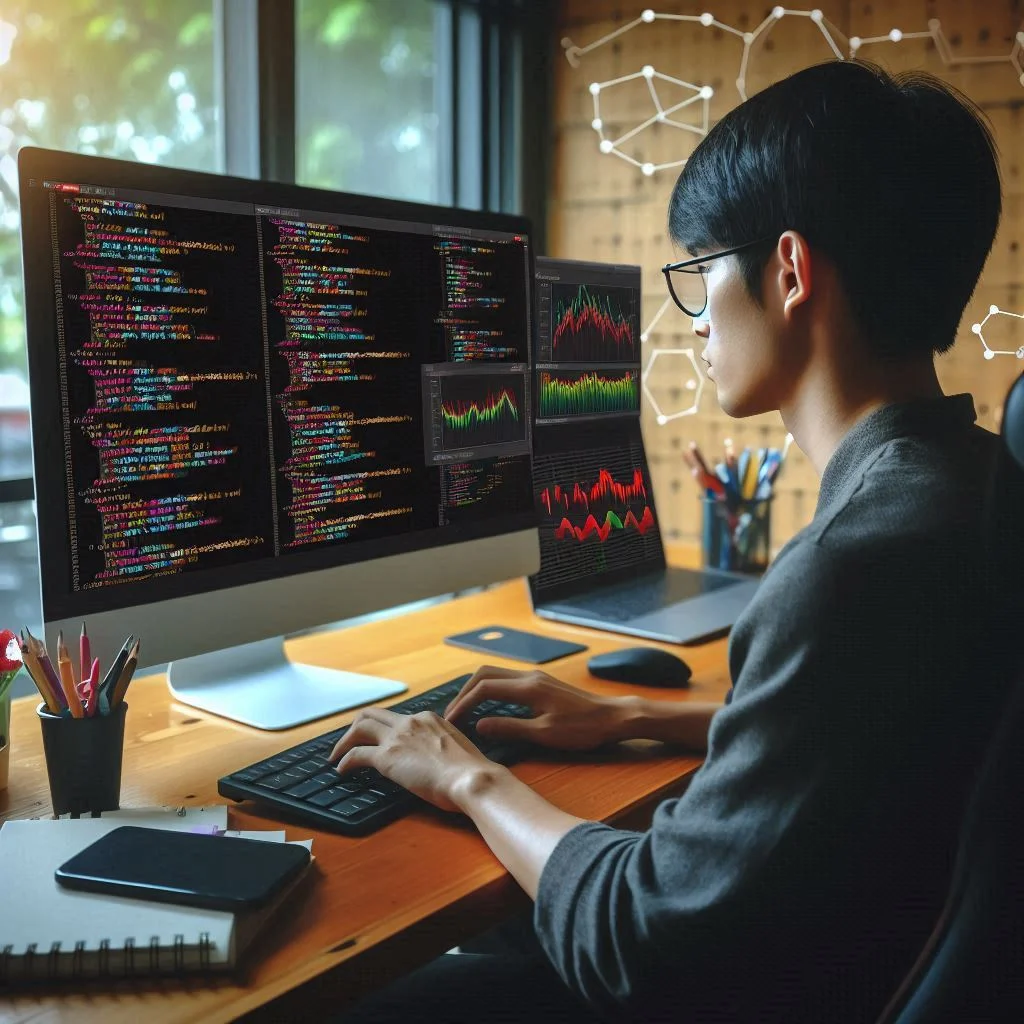
Understanding the Problem Statement
Before diving into any solution, it’s crucial to thoroughly understand the problem statement. Let’s consider a typical programming assignment: designing a program that allows a user to input 10 numbers, stores them in an array, and then displays all the numbers, along with the largest and smallest values.
Key Components to Address:
- Input: The program should prompt the user to enter 10 numbers.
- Storage: These numbers need to be stored in an array or list.
- Processing: The program should calculate the largest and smallest numbers from the stored array.
- Output: The program should display the list of numbers, the largest number, and the smallest number.
Understanding these components will guide you in breaking down the problem and designing a solution that addresses each aspect effectively.
Step 1: Creating a Solution Algorithm
An algorithm is a step-by-step procedure to solve a problem. Writing a solution algorithm in pseudocode helps you conceptualize the logic before jumping into actual coding. Pseudocode is language-agnostic and focuses on the logic rather than the syntax of a specific programming language.
Example Pseudocode for the Assignment:
START
Initialize an empty array to store 10 numbers
For i from 1 to 10 do
Prompt the user to enter a number
Store the number in the array at index i
End For
Initialize variables for largest and smallest numbers
Set largest to the first element of the array
Set smallest to the first element of the array
For each number in the array do
If the number is greater than largest then
Update largest to this number
End If
If the number is less than smallest then
Update smallest to this number
End If
End For
Display all numbers in the array
Display the largest number
Display the smallest number
END
Explanation of Pseudocode Steps:
- Initialization: Start by initializing an empty array to store the user’s numbers.
- Input Loop: Use a loop to prompt the user for input 10 times, storing each number in the array.
- Set Initial Values: Initialize largest and smallest with the first element of the array.
- Find Extremes: Loop through the array to update largest and smallest based on comparisons.
- Output: Display all numbers, the largest number, and the smallest number.
Step 2: Designing a Flowchart
A flowchart visually represents the logical flow of your program. It uses symbols to denote different types of actions and decision points. Creating a flowchart helps you visualize the program’s control flow and identify potential issues before coding.
Using RAPTOR to Create a Flowchart:
- Start Symbol: Begin with a Start symbol to indicate the start of the program.
- Input/Output Symbols: Use input/output symbols to prompt for user input and display results.
- Process Symbols: Represent operations like storing numbers and finding the largest and smallest numbers with process symbols.
- Decision Symbols: Use decision symbols to handle conditional checks, such as comparing numbers to update the largest and smallest values.
- End Symbol: Conclude the flowchart with an End symbol.
Steps to Create a Flowchart in RAPTOR:
1. Open RAPTOR: Launch RAPTOR and create a new flowchart.
2. Add Symbols:
- Drag and drop the Start symbol onto the workspace.
- Add input symbols for collecting numbers from the user.
- Use process symbols to store numbers in an array and perform calculations.
- Add decision symbols to compare numbers and determine the largest and smallest values.
- Place output symbols to display the results.
- End with the End symbol.
3. Connect Symbols: Use arrows to connect the symbols, representing the flow of execution.
4. Test and Refine: Test your flowchart to ensure it accurately represents the program logic. Refine as needed.
5. Snapshot: Capture a snapshot of your RAPTOR flowchart to include in your documentation.
Step 3: Implementing the Solution
Once you have your pseudocode and flowchart, it’s time to translate these into actual code. Here’s how to implement the solution using Python, a popular programming language known for its simplicity and readability.
Example Python Code:
# Initialize an empty list to store numbers
numbers = []
# Input 10 numbers from the user
for i in range(10):
number = float(input("Enter a number: "))
numbers.append(number)
# Find the largest and smallest numbers
largest = numbers[0]
smallest = numbers[0]
for num in numbers:
if num > largest:
largest = num
if num < smallest:
smallest = num
# Display the numbers, largest, and smallest
print("Numbers entered:", numbers)
print("Largest number:", largest)
print("Smallest number:", smallest)
Explanation of Code:
- Initialization: Create an empty list numbers to store user inputs.
- Input Loop: Use a for loop to collect 10 numbers from the user and append them to the numbers list.
- Find Extremes: Initialize largest and smallest with the first number in the list. Then, use a loop to compare each number in the list to update largest and smallest.
- Display Results: Print the list of numbers, the largest number, and the smallest number.
Step 4: Documenting Your Work
1. Word Document:
- Problem Statement: Include a clear description of the problem, outlining the requirements and objectives.
- Flowchart: Insert a snapshot of your RAPTOR flowchart.
- Algorithm: Provide the pseudocode you created.
- Output: Include a snapshot of the Master Console output from RAPTOR showing the results.
2. RAPTOR File:
- Save and submit the RAPTOR file containing your flowchart. This file will help others verify that your flowchart accurately represents the program logic.
Tips for Success:
- Understand the Requirements: Carefully read and analyze the problem statement before starting. Identify the key components and objectives.
- Plan Your Solution: Use pseudocode and flowcharts to plan your approach. This will help you avoid mistakes and ensure you cover all aspects of the problem.
- Implement Incrementally: Write and test your code in small sections. This makes it easier to identify and fix issues.
- Test Thoroughly: Test your program with various inputs to ensure it handles all possible scenarios correctly.
- Document Clearly: Provide clear and concise documentation. This helps others understand your solution and verify its correctness.
- Seek Help When Needed: If you encounter difficulties, don’t hesitate to seek help from peers, instructors, or online resources. Collaboration and additional resources can provide valuable insights.
Conclusion
Approaching programming assignments with a structured method will make the process more manageable and less intimidating. By following these steps—understanding the problem, creating a pseudocode algorithm, designing a flowchart, implementing the solution, and documenting your work—you’ll develop the skills to tackle any programming challenge with confidence.