MIPS assembly programming can be a challenging yet rewarding experience, especially when faced with assignments involving interactive applications or games. This guide provides a detailed approach to help you solve similar assignments effectively. While our example focuses on developing a basic game, the principles and strategies discussed here can be applied to various MIPS programming tasks.
When tackling a MIPS assembly game project, understanding the constraints and permitted syscalls is crucial. For instance, if your assignment restricts the use of certain syscalls, you will need to creatively manage tasks like input handling and display updates manually. This requires a solid grasp of Assembly Assignment Help techniques and careful planning.
Programming assignment help often involves structuring your solution to fit within the given limitations. By breaking down the problem into manageable components—such as designing the game board, handling user input, and implementing game logic—you can systematically address each part of the assignment. Remember, thorough testing and debugging are key to ensuring that your game functions as expected.
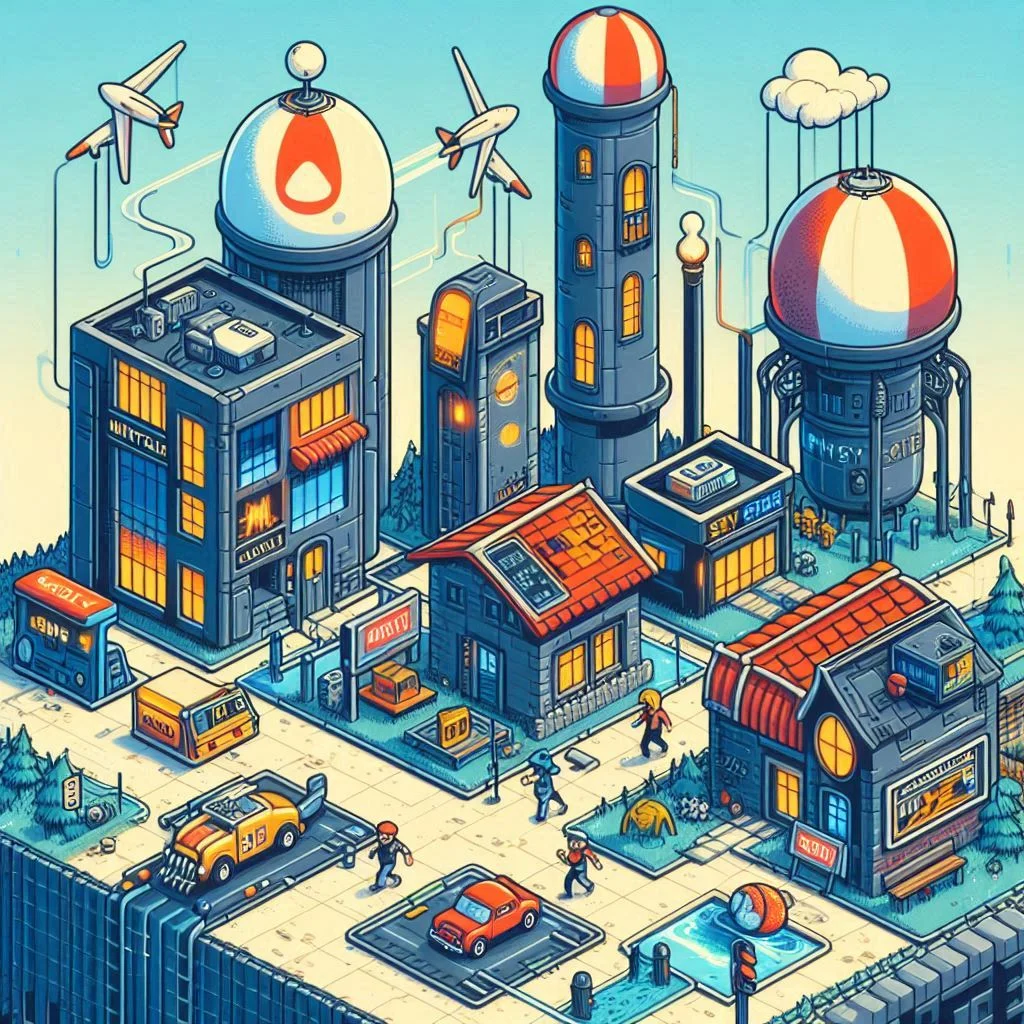
Incorporating these strategies will not only help you with this specific assignment but also enhance your overall skills in MIPS assembly programming. Whether you’re working on a basic game or a more complex project, applying these principles will lead to a more effective and successful implementation.
Understanding the Assignment
Before diving into the code, it's essential to fully understand the assignment's requirements and constraints. For instance, in our example assignment, you are tasked with writing a MIPS program for a basic game, subject to specific syscall limitations and using the MARS simulator for testing. Here’s how to break it down:
1. Analyze Key Components
Game Environment:
- Layout: You need to create a walled environment, represented as a 2D grid. The player (denoted by 'P') moves around this environment, collecting rewards ('R') and avoiding walls ('#').
- Interactions: The player should interact with the game using the keyboard (WASD keys). The game should also track and display the player’s score.
Game Rules:
- Movement and Scoring: The player earns points for collecting rewards and should avoid walls. The game ends when the player either collects 100 points or collides with a wall.
- End Conditions: On game termination, display a “GAME OVER” message along with the final score.
Syscalls:
- Permitted Syscalls: The assignment specifies which syscalls are allowed (memory allocation, print string, print integer, exit, set PRNG seed, generate random integer, and generate random integer in range). Other syscalls are not permitted.
2. Determine Your Constraints
Knowing the constraints helps you plan effectively. For this assignment:
- Syscall Limitations: You are restricted to a subset of syscalls. This means you must manually handle tasks usually covered by other syscalls, such as input and display management.
- Testing Environment: You must test your program on the MARS simulator, ensuring it functions as expected in the provided environment.
Planning Your Solution
A well-thought-out plan is crucial for successful implementation. Here’s how to approach planning:
1. Design Data Structures
Game Board Representation:
- 2D Array: Use a 2D array to represent the game board. Each cell can hold information about walls, rewards, or the player’s position.
Player and Rewards:
- Player Position: Track the player’s position on the board.
- Rewards: Store information about reward locations and update them as they are collected.
2. Develop a Flowchart
A flowchart helps visualize the game logic:
- Initialization: Set up the game environment, including the placement of walls, player, and rewards.
- Game Loop: Implement the main loop that handles user inputs, updates the game state, and checks for end conditions.
- Termination: Manage game termination by displaying the final score and a “GAME OVER” message.
Implementing the Game
With a solid plan in place, you can start coding. Follow these steps for effective implementation:
1. Set Up the Environment
Initialization Code:
- Memory Allocation: Use the sbrk syscall to allocate memory for your game board and other data structures.
- Display Setup: Initialize the display using memory-mapped I/O (MMIO) available in MARS. Set up the game board to be displayed.
Random Number Generation:
- Seed the PRNG: Use the set PRNG seed syscall to initialize the random number generator.
- Generate Random Locations: Use generate random integer or generate random integer in range to place rewards randomly.
2. Handle User Input
Keyboard Input:
- WASD Keys: Implement logic to read keyboard inputs and move the player accordingly. Use print integer to display the score and print string for debugging.
- Movement Logic: Ensure that movement is restricted by walls and update the player’s position based on input.
Collision Detection:
- Wall Collision: Check if the player’s new position after a move collides with a wall.
- Reward Collection: Verify if the player’s new position contains a reward and update the score accordingly.
3. Update Game State
Score Management:
- Update Score: Each time a reward is collected, increase the score by the designated amount (e.g., 5 points).
- Display Score: Continuously update the score display on the game board.
Reward Management:
- Reposition Rewards: After a reward is collected, generate a new random location for the next reward.
4. Implement End Game Conditions
Collision Handling:
- Wall Collision: End the game if the player collides with a wall. Display the final score and a “GAME OVER” message.
Score Threshold:
- 100 Points: End the game if the player reaches the score threshold (e.g., 100 points). Display the final score and a “GAME OVER” message.
Testing and Debugging
Testing and debugging are crucial steps to ensure your game works as intended:
1. Testing on MARS Simulator
Functionality Check:
- Test All Features: Verify that all game features, such as movement, score tracking, and reward collection, work as expected.
- Edge Cases: Test edge cases, such as collisions with walls and reaching the score limit, to ensure the game ends correctly.
Performance Evaluation:
- Check Performance: Ensure that the game runs smoothly and responds promptly to user inputs.
2. Debugging
Using Permitted Syscalls:
- Debug Output: Use the print string and print integer syscalls for debugging purposes to display information about the game state and track down issues.
Iterative Testing:
- Fix Issues: Address any bugs or issues found during testing. Iterate on your code to improve functionality and fix problems.
Writing the Report
A well-documented report is essential for explaining your approach and demonstrating your understanding:
1. Document Your Approach
Game Design:
- Structure: Explain how you structured your game and the rationale behind your design decisions.
Implementation Details:
- Syscall Usage: Describe how you used the permitted syscalls and managed tasks like input handling and display.
Testing Process:
- Testing Steps: Outline the steps you took to test and debug your game. Include any issues encountered and how they were resolved.
2. Include Code Snippets
Code Examples:
- Key Sections: Provide relevant code snippets to illustrate key parts of your implementation, such as the game loop, input handling, and score management.
Submission
Proper submission ensures that your work is evaluated correctly:
1. Prepare Your Files
Organize Files:
- Code and Report: Ensure all required files, including the MIPS code and report, are organized and prepared for submission.
2. Verify Compliance
Adhere to Guidelines:
- Assignment Constraints: Double-check that your code meets the assignment’s constraints and guidelines, including syscall limitations and testing requirements.
Conclusion
By following this guide, you can systematically tackle MIPS assembly programming assignments and similar tasks. Whether you’re developing a game or working on another application, a structured approach will help you manage complexity and produce a robust solution. Remember to test thoroughly, document your work clearly, and seek help if needed. Good luck with your assignments!