Programming assignments involving the creation of custom data structures can be both challenging and rewarding. They require not only a deep understanding of fundamental programming concepts but also the ability to design efficient, robust solutions. Whether you are tasked with implementing a new type of collection class or developing a novel data structure, approaching these assignments systematically can make a significant difference.
In this blog, we'll explore effective strategies for tackling such complex assignments, using a sample problem as a guide. For students who find themselves struggling with these tasks, Java assignment help can provide valuable insights and support. When faced with the intricacies of designing a custom collection class, leveraging such resources can enhance your understanding and execution.
Creating a custom collection class with dynamic arrays involves several key steps. You'll need to design a data structure that combines the best aspects of linked lists and array lists, ensuring efficient memory usage and fast access times. Programming assignment challenges like this often require careful planning and a methodical approach to achieve optimal results.
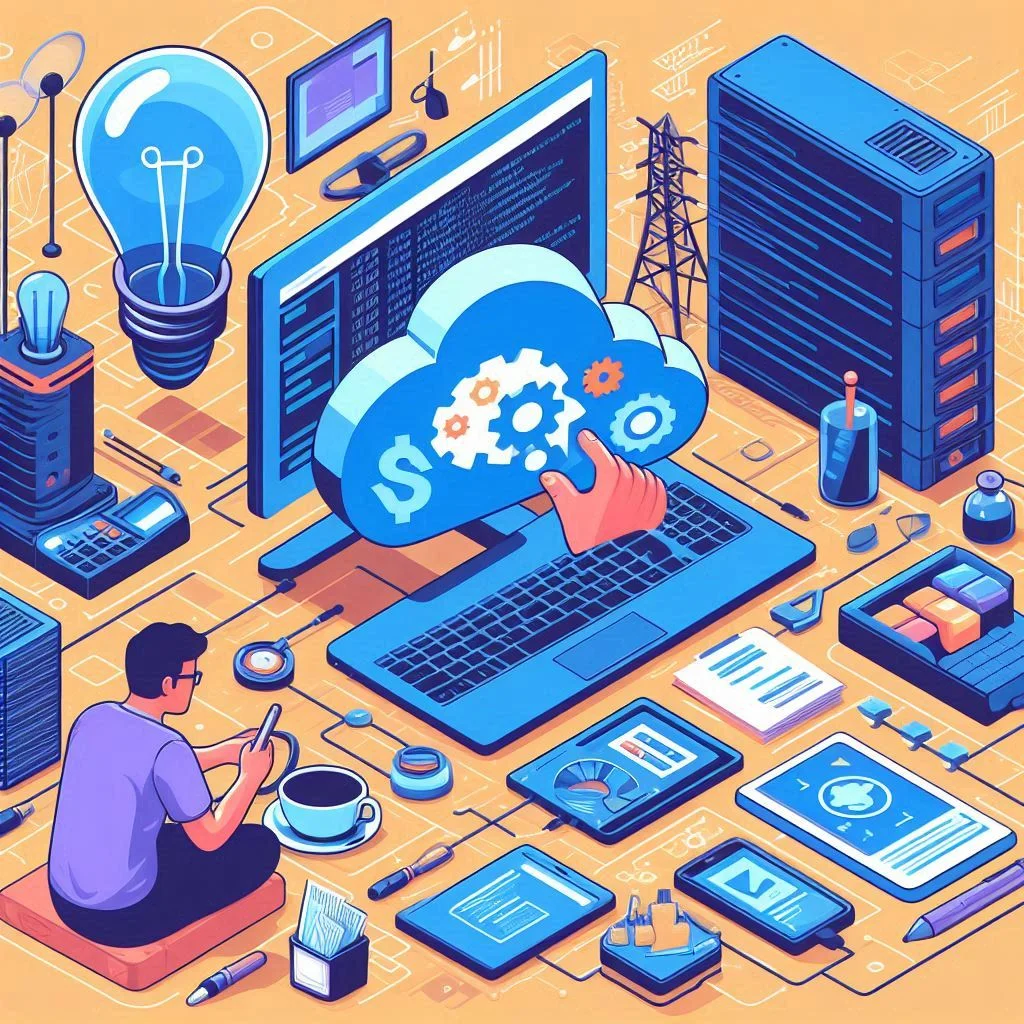
Incorporating Java assignment help into your study routine can aid in mastering these complex concepts. Professional guidance can streamline the learning process, helping you to better grasp the principles and apply them effectively in your assignments. By understanding the core elements and receiving targeted assistance, you can tackle custom data structures with greater confidence and efficiency.
Understanding the Problem
Before jumping into code, it's crucial to have a clear grasp of the problem at hand. Let's consider a typical assignment that involves creating a custom collection class—a hybrid data structure that combines aspects of linked lists and array lists. The goal is to design a class that manages a collection of items efficiently while balancing the strengths and weaknesses of both linked lists and array lists.
Key Considerations
- Linked Lists: These are dynamic data structures that consist of nodes, where each node contains a reference to the next node. They offer efficient insertion and deletion operations but can be slow due to the time required to traverse the list.
- Array Lists: Array lists provide fast access to elements via indices but can incur significant overhead when resizing the underlying array, especially if it needs to be frequently expanded.
- Hybrid Approach: Combining linked lists and arrays aims to leverage the advantages of both structures. However, this approach introduces its own set of challenges, such as managing the linked list of arrays and handling resizing efficiently.
By understanding these trade-offs, you can begin designing a data structure that optimally balances these factors.
Designing the Data Structure
Choosing the Right Components
- Small Arrays: Decide on the size of the small arrays that will be used in your custom collection class. For instance, you might choose a default size of 5 for simplicity but also allow users to specify a different size if needed.
- Linked List Nodes: Implement the linked list nodes to hold the small arrays and reference the next node. Each node should be able to manage a small array of items and provide methods to access and modify these items.
Core Features Implementation
- Constructors: Provide multiple constructors to initialize the collection class with different configurations. For example, you might offer a default constructor that initializes the collection with a small array of a default size, and another constructor that allows specifying both the size of the small arrays and the initial number of small arrays.
- Add and Remove Operations: Implement methods to add and remove elements. Consider edge cases such as inserting an element at the end, in the middle, or removing an element. Ensure that the implementation handles scenarios where small arrays are full or empty.
- Tracking the Last Item: Maintain a reference to the last item in the list to optimize append operations. This involves keeping track of the end of the current small array and handling cases where elements are added to a new small array.
Handling Edge Cases
- Empty Arrays: Ensure that empty small arrays are removed from the list when they no longer contain any items. This helps prevent wasted memory and keeps the list clean.
- Dynamic Resizing: If the collection grows beyond the capacity of the current small arrays, manage resizing efficiently. This might involve creating new small arrays and updating the linked list to include these new arrays.
Implementing and Testing
Implementing Iterators
1. Iterator Class: Implement an iterator class that adheres to the Iterator interface. This class should allow traversal of the custom collection class, enabling users to iterate over the elements.
public class ListArrayIterator implements Iterator {
private Node currentNode;
private int currentIndex;
private T[] currentArray;
public ListArrayIterator(Node head) {
this.currentNode = head;
this.currentIndex = 0;
this.currentArray = head.array;
}
@Override
public boolean hasNext() {
while (currentNode != null && currentIndex >= currentArray.length) {
currentNode = currentNode.next;
if (currentNode != null) {
currentArray = currentNode.array;
currentIndex = 0;
}
}
return currentNode != null && currentIndex < currentArray.length;
}
@Override
public T next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
return currentArray[currentIndex++];
}
}
Unit Testing
- Basic Functionality: Test fundamental operations such as adding, removing, and accessing elements. Verify that these operations work as expected in simple scenarios.
- Edge Cases: Test extreme scenarios, such as adding a large number of items in succession or removing all items. Ensure that the implementation handles these cases correctly and efficiently.
- Error Handling: Check how the implementation deals with errors, such as attempting to remove an item that doesn’t exist or accessing an element by an invalid index. Ensure that appropriate exceptions are thrown and handled.
Code Example
Below is a simplified example of the core structure of the custom collection class:
public class ListArray {
private static final int DEFAULT_ARRAY_SIZE = 5;
private Node head;
private Node tail;
private int size;
private int arraySize;
public ListArray() {
this(DEFAULT_ARRAY_SIZE);
}
public ListArray(int arraySize) {
this.arraySize = arraySize;
this.head = new Node<>((T[]) new Object[arraySize]);
this.tail = head;
this.size = 0;
}
public void add(T item) {
// Implementation here
}
public void add(int index, T item) {
// Implementation here
}
public void remove(int index) {
// Implementation here
}
public Iterator iterator() {
return new ListArrayIterator<>(head);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
Node currentNode = head;
while (currentNode != null) {
sb.append(Arrays.toString(currentNode.array)).append(" -> ");
currentNode = currentNode.next;
}
return sb.append("null").toString();
}
private static class Node {
private T[] array;
private Node next;
public Node(T[] array) {
this.array = array;
this.next = null;
}
}
}
Optimization Strategies
- Time Efficiency: Strive for time-efficient operations. For instance, maintaining a reference to the last item can optimize append operations and reduce the need for frequent traversal.
- Memory Management: Monitor and manage memory usage effectively. Ensure that your implementation does not waste memory by removing empty small arrays and optimizing the size of the arrays.
Conclusion
Tackling complex programming assignments involving custom data structures requires a structured approach. By thoroughly understanding the problem, designing an efficient data structure, implementing core functionalities, and rigorously testing your solution, you can handle these challenges with confidence. Focus on balancing efficiency and functionality to create robust, effective solutions.