Programming assignments frequently confront students with intricate challenges that demand not just coding proficiency but also strategic foresight and adept problem-solving abilities. In this comprehensive guide, we delve into the nuanced landscape of handling complex programming tasks by examining a prototypical example: the Basic Maze and File Parsing assignment. Our goal is to furnish students with a meticulously structured approach that enhances their capacity to navigate and conquer such assignments with confidence and efficiency. By dissecting each facet of the assignment—from initial comprehension of requirements and project setup to the implementation of fundamental functionalities and management of input files—we equip learners with a definitive roadmap. This roadmap is designed to empower them to tackle similar programming challenges effectively and methodically, offering help with Java assignments. Throughout this guide, emphasis is placed not just on the technical aspects of coding, but also on the crucial skills of interpreting UML diagrams, adhering to Java coding conventions, and employing object-oriented methodologies seamlessly. By providing practical steps, insightful strategies, and troubleshooting tips, this guide serves as a valuable resource for students seeking to cultivate both their technical acumen and their proficiency in navigating the complexities inherent in programming assignments.
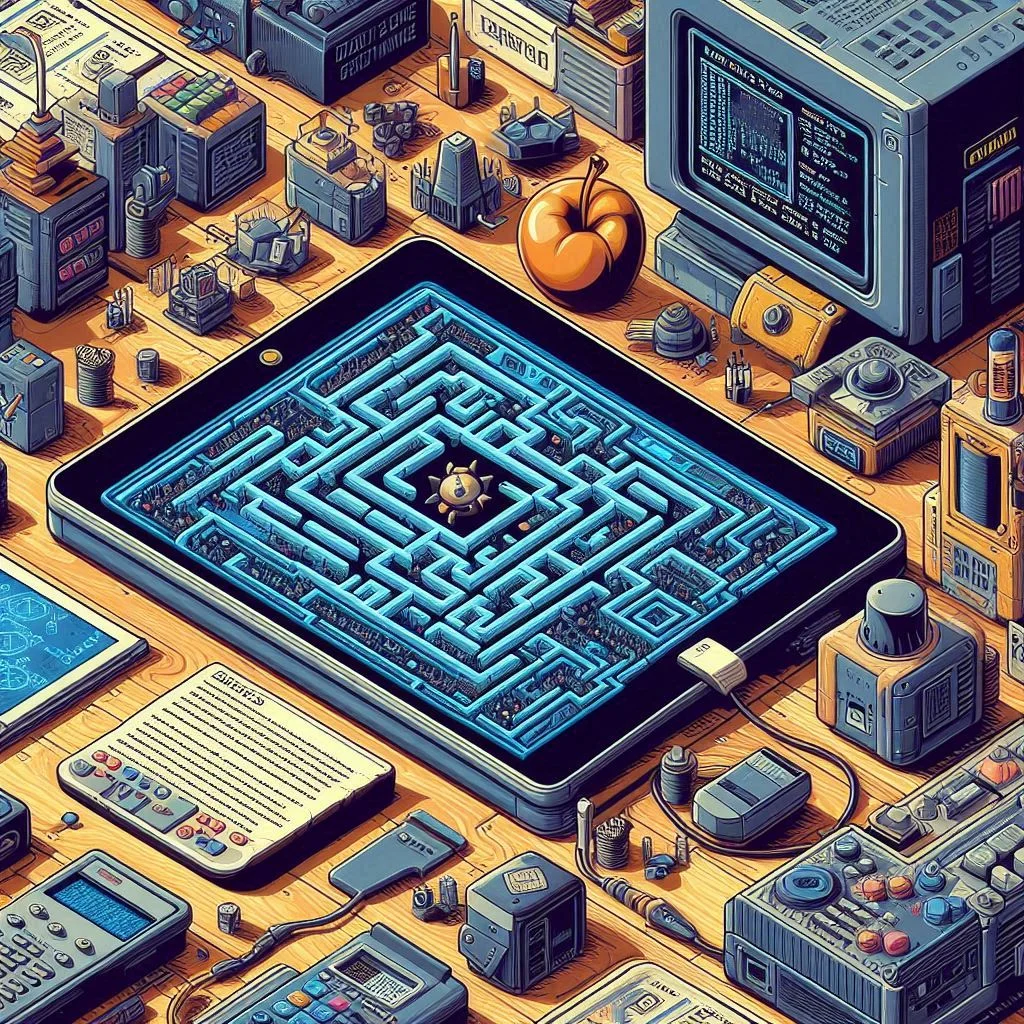
Understanding the Assignment
Before diving into the code, it's crucial to thoroughly grasp the assignment requirements. In our example, the goal is to create a maze using Java, starting from an empty structure and progressively adding rooms defined in input files. Let's break down the assignment into manageable stages and explore how to approach each one.
Stage 1: Setting Up the Project
The first step is to obtain and set up the project files. Typically, assignments are distributed as compressed folders containing necessary source code, libraries, and documentation.
- Download and Extraction: Begin your assignment journey by accessing the designated files from your course platform, such as Blackboard or Moodle. Once downloaded, proceed to extract the contents to a suitable directory on your local computer. Organizing these files systematically ensures you have easy access and clarity on where your project materials reside.
- Importing into IDE: Utilize a robust Integrated Development Environment (IDE) like IntelliJ IDEA or an equivalent software tool. Import the extracted project folder into your IDE as a new project. This step is pivotal as it sets the foundation for your coding environment. Note that the process may vary slightly depending on the IDE you're using. Consult platform-specific guides or documentation provided by your course instructor for detailed instructions tailored to your setup.
- Initial Compilation: Once your project is imported, take the crucial step of compiling the code. Compile the entire project within your IDE to verify that your development environment is correctly configured. A successful compilation indicates that basic dependencies and configurations are correctly in place, laying a solid groundwork for subsequent development tasks.
- Troubleshooting Tips: During the setup phase, encountering errors is not uncommon. If you face challenges such as unresolved dependencies, project structure discrepancies, or IDE-specific configuration issues, refer to the assignment's documentation for troubleshooting guidance. These resources often contain valuable insights and solutions to common issues encountered by students. Addressing these challenges promptly ensures a smooth transition into the development phase, minimizing potential disruptions to your workflow.
Stage 2: Implementing Basic Functionality
With the project set up, the next stage involves implementing basic functionality as outlined in the assignment.
- Understanding UML Diagrams: Begin by immersing yourself in the provided UML (Unified Modeling Language) diagrams. These visual representations offer a blueprint of the program's structure, detailing classes, methods, and their relationships. Take time to analyze and interpret these diagrams thoroughly. By identifying key components and their interactions, you gain insights into how various parts of the program integrate and function together. This foundational understanding is essential for effectively translating the UML design into tangible Java code during the implementation phase of your assignment.
- Creating an Empty Maze: Transitioning from conceptualization to implementation, the next step involves crafting an initial framework for your maze structure. Modify existing methods or introduce new ones to establish an empty maze. This pivotal phase typically entails initializing maze objects and establishing foundational interfaces that will support future functionalities. By setting up this basic infrastructure, you lay the groundwork for dynamically expanding and refining the maze structure as you progress through subsequent stages of development.
- Adding Rooms to the Maze: With the foundational maze structure in place, proceed to enrich your program by integrating rooms within the maze environment. Extend your codebase to accommodate the inclusion of distinct rooms, each characterized by specific attributes such as walls, doors, and other architectural elements. Adhere closely to the guidelines set forth in the UML design, ensuring that each room is meticulously defined and integrated into the broader maze framework. This iterative process not only enhances the complexity and realism of your maze but also reinforces your understanding of translating design concepts into functional Java implementations.
- Object-Oriented Techniques: Embrace the principles of object-oriented programming (OOP) as you refine and expand your codebase. Apply concepts such as encapsulation, inheritance, and polymorphism to architect a modular and scalable solution for your maze assignment. Define distinct classes for rooms, maze structures, and any supplementary components mandated by the assignment's requirements. By leveraging OOP methodologies, you streamline code maintenance, promote code reusability, and foster a structured approach to software design. This systematic application of OOP principles not only enhances the clarity and maintainability of your code but also reinforces your proficiency in employing industry-standard practices within real-world programming scenarios.
Stage 3: Working with Input Files
The assignment progresses to reading maze structures from input files, such as small.maze and large.maze.
- Understanding Input Formats: Begin by thoroughly examining the structure and content of the maze input files provided for your assignment. These files typically detail how rooms are configured; specifying crucial elements such as wall placements, door connections linking rooms, and unique identifiers for each room. Familiarizing yourself with these specifics lays a solid foundation for accurate interpretation and utilization of the input data in your program.
- Implementing File Parsing: Proceed by developing robust parsing mechanisms designed to extract and interpret maze configurations from the designated input files. Start your implementation journey with smaller files like 'small.maze' to validate and refine your parsing logic. This iterative approach ensures that your code effectively handles varying complexities and scales seamlessly when processing larger files such as 'large.maze.
- Error Handling: Prioritize the implementation of comprehensive error-checking protocols within your parsing logic. Establish mechanisms to meticulously validate the format and integrity of input files, preemptively identifying and addressing discrepancies or unexpected data entries. By integrating robust error-handling routines, you enhance the resilience and reliability of your program, facilitating smoother debugging processes and mitigating potential runtime issues.
- Integration and Testing: Seamlessly integrate your meticulously crafted file parsing functionality into the core program workflow. Conduct rigorous testing scenarios using diverse sets of input files to rigorously assess the program's capability in generating accurate maze structures and validating input configurations. Through systematic testing practices, ensure that your program consistently delivers expected outcomes across varying input scenarios, thereby validating its functionality and adherence to assignment requirements.
Compiling and Testing
Throughout the implementation stages, frequent compilation and testing are essential to verify the correctness and functionality of your code.
- Compiling Modified Programs: Ensure to regularly compile your modified programs to detect and rectify any syntax errors, logical inconsistencies, or potential runtime issues. Timely resolution of compilation errors is essential to uphold the integrity and functionality of your codebase, maintaining a stable development environment conducive to further refinement and enhancement.
- Testing Functionality: Execute thorough testing procedures to validate the comprehensive functionality of your program. Assess the accuracy of maze generation algorithms, verify the precision of file parsing mechanisms, and evaluate overall program performance across diverse input scenarios. Leveraging sample input files provided in the assignment facilitates simulation of various usage scenarios and edge cases, ensuring robust validation of your program's capabilities.
- Debugging Strategies: Harness the full potential of debugging tools integrated within your IDE to effectively troubleshoot and resolve programmatic issues. Utilize these tools to meticulously trace the execution flow of your code, inspect variable states in real-time, and pinpoint the root causes of logical errors or unexpected behaviors. Proficiency in debugging is instrumental in navigating and overcoming complex programming challenges, facilitating efficient problem-solving and code optimization processes.
Conclusion
Successfully navigating complex programming assignments requires a systematic approach encompassing understanding requirements, setting up the development environment, implementing core functionalities, and rigorous testing. By following the steps outlined in this guide, students can enhance their problem-solving skills and achieve success in similar programming tasks.
Remember, while assignments may vary in complexity and specific requirements, the fundamental principles discussed here—understanding UML diagrams, implementing Java code, and leveraging object-oriented techniques—are universally applicable. Embrace challenges, seek assistance when needed, and approach each assignment with confidence in your growing programming expertise.
By mastering these steps, you'll not only complete your assignments effectively but also lay a solid foundation for tackling more advanced programming challenges in your academic and professional journey.