Programming assignments often require students to design algorithms to solve specific problems. Whether you're a beginner or seeking to improve your skills, understanding how to break down problems and create effective solutions is crucial. Flowcharts are an excellent tool for visualizing and organizing the steps involved in solving a problem. They help to clarify the sequence of operations and can make the design of your algorithm more straightforward.
In this guide, we will walk you through the process of using flowcharts to solve a typical algorithm assignment. We’ll start by breaking down a problem into manageable parts and illustrate how to represent these parts visually with a flowchart. This approach will not only enhance your ability to design algorithms but also improve your problem-solving skills.
By following a step-by-step method, you can better understand the flow of data and operations required to complete a task. Flowcharts provide a clear roadmap for implementing algorithms, helping you to organize your thoughts and identify potential issues before coding begins. This ensures a more structured approach to problem-solving and can significantly improve the quality of your programming assignments.
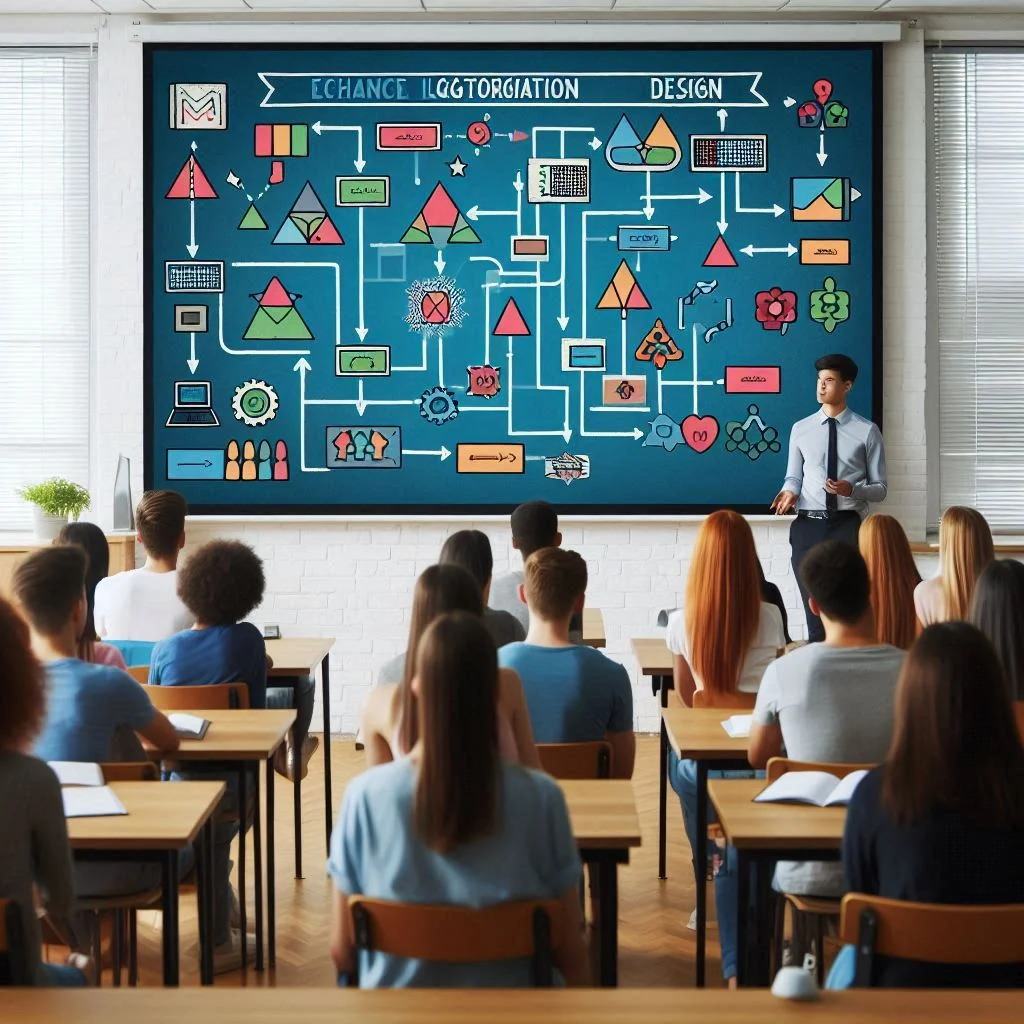
Problem Statement
Let’s start with a simple problem statement to illustrate our process:
Problem: You need to create an algorithm that performs the following operations:
- Receive an integer input from the user.
- Add 5 to the integer.
- Double the result.
- Subtract 7 from the doubled value.
- Display the final result.
This problem requires a series of arithmetic operations and basic user input/output handling. By dissecting this problem, you’ll learn how to approach a variety of algorithmic assignments.
Step 1: Defining the Problem
To effectively solve any problem, it's crucial to define its components clearly. For this problem, we need to break it down into three main elements:
- Input: The integer value that the user will provide.
- Processing: The arithmetic operations to be performed on the input.
- Output: The final result that will be displayed to the user.
Defining Diagram:
Here’s a simple diagram to illustrate the relationship between these components:
Input | Processing | Output |
---|---|---|
integer | Promptfor integer | final_number |
Getinteger | ||
Calculatefinal_number | ||
Displayfinal_number |
This diagram helps to visualize the flow of data and operations from input to output, providing a clear roadmap for implementing the algorithm.
Step 2: Creating a Flowchart
A flowchart is a visual representation of the algorithm, showing the sequence of steps and decision points. To create a flowchart for our problem, follow these steps:
- Start: Begin the process.
- Input: Prompt the user to enter an integer.
- Process:
- Read the integer input.
- Add 5 to the integer.
- Double the result.
- Subtract 7 from the doubled value.
- Output: Display the final result.
- End: Complete the process.
Flowchart Example:
In this flowchart:
- Start initiates the process.
- Input involves asking the user to enter a number.
- Processing steps include arithmetic operations.
- Output displays the result.
- End signifies the completion of the process.
Step 3: Writing the Solution Algorithm
An algorithm is a step-by-step procedure to solve the problem. For our example, the algorithm can be written as follows:
Calculate_final_number
- Prompt for integer
- Get integer
- New_number = (integer + 5) * 2
- Final_number = new_number - 7
- Display 'The final number is ', final_number
END
Explanation of the Steps:
- Prompt for integer: Ask the user to enter an integer value.
- Get integer: Read the integer value entered by the user.
- Calculate new_number: Add 5 to the integer and then double the result. This step uses the formula: new_number = (integer + 5) * 2.
- Calculate final_number: Subtract 7 from the new_number to get the final result: final_number = new_number - 7.
- Display result: Show the final number to the user with a descriptive message.
This algorithm provides a clear and concise method for solving the problem. It ensures that each step is logically sequenced and easy to follow.
Step 4: Performing Desk Checking
Desk checking involves manually verifying the algorithm with sample inputs to ensure its correctness. This step is crucial for identifying errors and ensuring that the algorithm performs as expected.
Input Data:
First Data Set:
- Integer = 10
•Second Data Set:
- Integer = 50
Expected Results:
- For Integer = 10:
- o final_number should be 23.
- For Integer = 50:
- o final_number should be 103.
Desk Check Table:
Statement Number | First Pass | Second Pass |
---|---|---|
1, 2 | 10 | 50 |
3 | 30 | 110 |
4 | 23 | 103 |
5 | Display | Display |
Detailed Desk Check:
First Pass (integer = 10):
- Input integer: 10
- Calculate new_number = (10 + 5) * 2 = 30
- Calculate final_number = 30 - 7 = 23
- Display result: "The final number is 23"
Second Pass (integer = 50):
- Input integer: 50
- Calculate new_number = (50 + 5) * 2 = 110
- Calculate final_number = 110 - 7 = 103
- Display result: "The final number is 103"
Desk checking helps to ensure that the algorithm produces the correct results for different input values. By following these steps, you can validate that your solution is accurate and reliable.
Tips for Solving Similar Assignments
To effectively tackle similar programming assignments, follow these tips:
- Understand the Problem: Carefully read the problem statement and make sure you understand what is required. Break the problem into smaller parts if needed.
- Break Down the Problem: Divide the problem into input, processing, and output components. This will help you organize your approach and ensure that all aspects of the problem are addressed.
- Create Visual Aids: Use flowcharts to visualize the steps of your algorithm. Flowcharts help to clarify the sequence of operations and make it easier to identify any logical errors.
- Write a Clear Algorithm: Ensure that each step of your algorithm is clear and logical. Avoid ambiguous instructions and make sure that the algorithm can be easily followed.
- Perform Desk Checking: Validate your algorithm with sample inputs to ensure that it produces the correct results. Desk checking helps to identify any errors and ensures that your solution is accurate.
- Practice Regularly: The more you practice solving programming problems, the more proficient you will become. Work on a variety of problems to build your skills and gain confidence.
- Seek Feedback: If possible, review your algorithm with peers or mentors. Feedback can provide valuable insights and help you improve your problem-solving skills.
By following these steps and tips, you’ll be well-equipped to handle a range of algorithmic programming assignments. Remember, the key to success is a clear understanding of the problem, a well-structured approach, and thorough validation of your solution.
Conclusion
Algorithmic programming assignments are an excellent way to develop problem-solving skills and enhance your understanding of computational logic. By following the steps outlined in this guide—defining the problem, creating flowcharts, writing algorithms, and performing desk checking—you can effectively tackle various types of assignments and build a solid foundation for more complex programming challenges.
Remember, practice is essential to mastering algorithmic problem-solving. Keep working on different problems, apply the principles you’ve learned, and don’t hesitate to seek help when needed. With persistence and practice, you’ll become proficient at solving programming assignments and excel in your programming endeavors.