Designing and implementing a Point of Sale (POS) system using SQL/PLSQL can be a challenging task, particularly for those tackling complex SQL/PLSQL assignments. However, breaking down the process into manageable steps makes it achievable. To start, it’s crucial to design a comprehensive database schema that forms the foundation of the system, addressing entities such as employees, customers, invoices, and items. Next, implementing procedures and packages will help encapsulate the necessary business logic, making operations like adding new customers or items more efficient. Effective transaction handling ensures data consistency, while robust database security protects against unauthorized access and modifications. Finally, generating reports is essential for data analysis and documentation, providing valuable insights into the system’s performance. For those seeking additional support, SQL/PLSQL assignment help can offer guidance and expertise, simplifying the development of a well-designed and functional POS system. By leveraging programming assignment assistance, you can ensure that every aspect of your system is thoroughly addressed, from schema design to security, resulting in a reliable and effective solution.
Designing the Database Schema
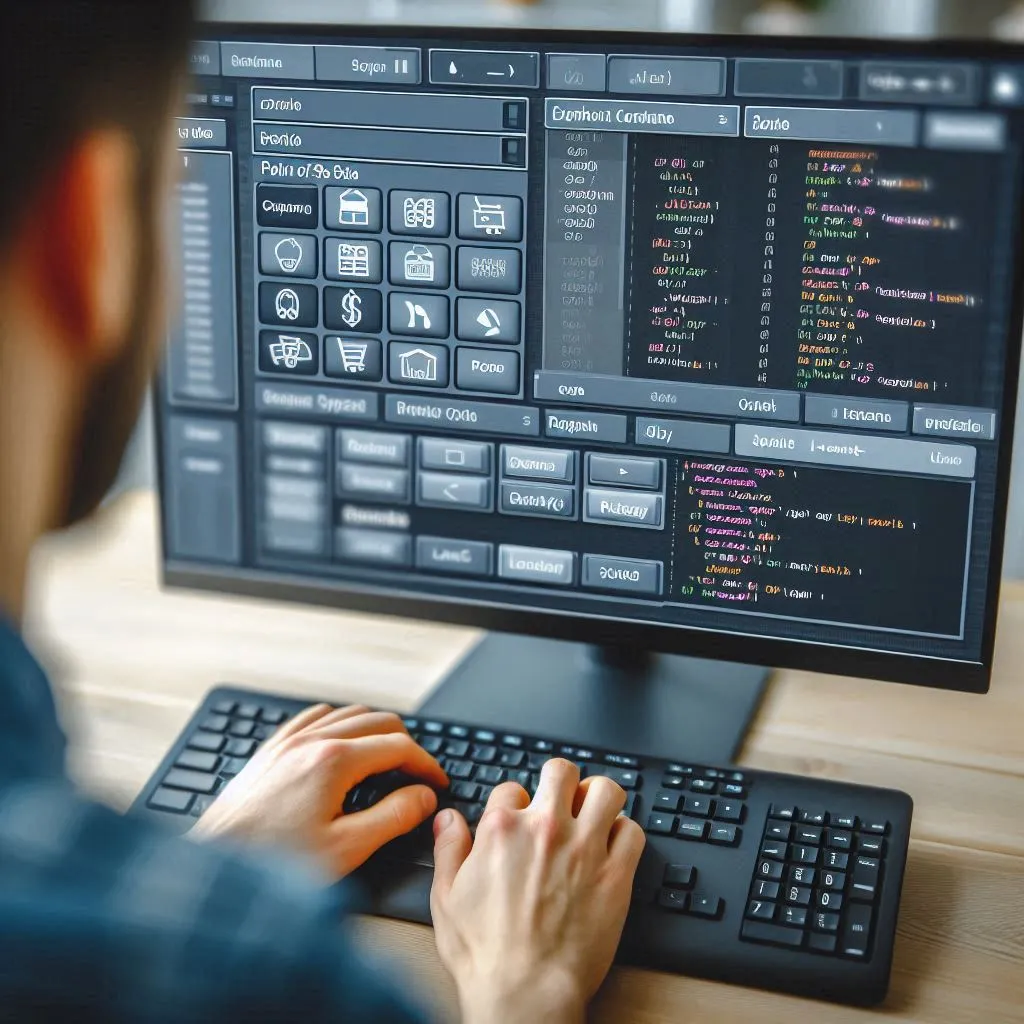
The database schema forms the foundation of any SQL/PLSQL system. Proper design is crucial as it affects the efficiency, integrity, and scalability of the system.
Identify Entities and Relationships
1. Identify Entities: Start by listing all the entities required for your system. For a POS system, entities might include Employee, Customer, Invoice, Item, Category, and Branch.
2. Define Relationships: Determine how these entities interact. For example:
- An Invoice is linked to an Employee, a Customer, and a Branch.
- An Item belongs to a Category and is included in an Invoice through InvoiceDetail.
Design Tables
1. Create Tables: Define tables for each entity with appropriate attributes and data types. For example:
CREATE TABLE Employee (
employeeNo NUMBER PRIMARY KEY,
employeeName VARCHAR2(50),
job VARCHAR2(30)
);
CREATE TABLE Customer (
customerNo NUMBER PRIMARY KEY,
customerName VARCHAR2(50),
gender CHAR(1)
);
CREATE TABLE Invoice (
invoiceNo NUMBER PRIMARY KEY,
employeeNo NUMBER,
customerNo NUMBER,
branchNo NUMBER,
totalPrice NUMBER,
invoiceDate DATE,
FOREIGN KEY (employeeNo) REFERENCES Employee(employeeNo),
FOREIGN KEY (customerNo) REFERENCES Customer(customerNo),
FOREIGN KEY (branchNo) REFERENCES Branch(branchNo)
);
CREATE TABLE Item (
itemNo NUMBER PRIMARY KEY,
itemName VARCHAR2(50),
price NUMBER,
totalQuantity NUMBER,
catNo NUMBER,
FOREIGN KEY (catNo) REFERENCES Category(catNo)
);
CREATE TABLE Category (
catNo NUMBER PRIMARY KEY,
categoryName VARCHAR2(50)
);
CREATE TABLE Branch (
branchNo NUMBER PRIMARY KEY,
branchName VARCHAR2(50)
);
CREATE TABLE InvoiceDetail (
invoiceNo NUMBER,
itemNo NUMBER,
paidPrice NUMBER,
quantity NUMBER,
PRIMARY KEY (invoiceNo, itemNo),
FOREIGN KEY (invoiceNo) REFERENCES Invoice(invoiceNo),
FOREIGN KEY (itemNo) REFERENCES Item(itemNo)
);
2. Define Constraints: Ensure data integrity by applying constraints. For example, use foreign keys to enforce relationships between tables:
ALTER TABLE InvoiceDetail
ADD CONSTRAINT fk_invoice FOREIGN KEY (invoiceNo)
REFERENCES Invoice(invoiceNo);
ALTER TABLE InvoiceDetail
ADD CONSTRAINT fk_item FOREIGN KEY (itemNo)
REFERENCES Item(itemNo);
Create the Database User and Grant Privileges
1. Create User:
GRANT CONNECT, RESOURCE TO pointOfSal;
GRANT ALL PRIVILEGES TO pointOfSal;
2. Grant Privileges:
GRANT CONNECT, RESOURCE TO pointOfSal;
GRANT ALL PRIVILEGES TO pointOfSal;
Implementing Procedures and Packages
Procedures and packages encapsulate logic and facilitate operations on the database. They are crucial for maintaining the system’s functionality.
Create Database Packages
1. Define Procedures and Functions:
- Add Customer Procedure:
CREATE OR REPLACE PACKAGE pointOfSal AS
PROCEDURE addCustomer(p_name VARCHAR2);
FUNCTION getCategoryNo(p_name VARCHAR2) RETURN NUMBER;
-- Other procedures and functions
END pointOfSal;
2. Implement Procedures:
- Add Customer Procedure Implementation:
CREATE OR REPLACE PROCEDURE pointOfSal.addCustomer(p_name VARCHAR2) AS
v_count NUMBER;
BEGIN
SELECT COUNT(*) INTO v_count
FROM Customer
WHERE customerName = p_name;
IF v_count = 0 THEN
INSERT INTO Customer (customerName)
VALUES (p_name);
DBMS_OUTPUT.PUT_LINE('Customer added successfully.');
ELSE
DBMS_OUTPUT.PUT_LINE('Customer already exists.');
END IF;
END addCustomer;
- Add Item Procedure Implementation:
CREATE OR REPLACE PROCEDURE pointOfSal.addItem(p_itemName VARCHAR2, p_price NUMBER, p_quantity NUMBER, p_categoryName VARCHAR2) AS
v_catNo NUMBER;
v_itemNo NUMBER;
BEGIN
v_catNo := getCategoryNo(p_categoryName);
IF v_catNo = -1 THEN
DBMS_OUTPUT.PUT_LINE('Category does not exist.');
RETURN;
END IF;
SELECT itemNo INTO v_itemNo
FROM Item
WHERE itemName = p_itemName;
IF v_itemNo IS NULL THEN
INSERT INTO Item (itemName, price, totalQuantity, catNo)
VALUES (p_itemName, p_price, p_quantity, v_catNo);
DBMS_OUTPUT.PUT_LINE('Item added successfully.');
ELSE
DBMS_OUTPUT.PUT_LINE('Item already exists.');
END IF;
END addItem;
Utilize Cursors and Exception Handling
1. Using Cursors:
- Example of Cursor for Adding Employee:
CREATE OR REPLACE PROCEDURE pointOfSal.addEmployee(p_name VARCHAR2, p_job VARCHAR2) AS
CURSOR c_employee IS
SELECT employeeNo FROM Employee WHERE employeeName = p_name;
v_count NUMBER;
BEGIN
OPEN c_employee;
FETCH c_employee INTO v_count;
CLOSE c_employee;
IF v_count IS NULL THEN
INSERT INTO Employee (employeeName, job)
VALUES (p_name, p_job);
DBMS_OUTPUT.PUT_LINE('Employee added successfully.');
ELSE
DBMS_OUTPUT.PUT_LINE('Employee already exists.');
END IF;
END addEmployee;
2. Exception Handling:
- Handling No Data Found Exception:
BEGIN
-- Code that might raise NO_DATA_FOUND exception
EXCEPTION
WHEN NO_DATA_FOUND THEN
DBMS_OUTPUT.PUT_LINE('No data found.');
END;
Creating Views and Handling Transactions
Views simplify data retrieval and improve security by controlling access to the underlying tables. Transaction management ensures data consistency and integrity.
a. Define Views
1. Create Views:
- Invoice Master View:
CREATE VIEW invoiceMaster AS
SELECT i.invoiceNo, i.invoiceDate, i.totalPrice, e.employeeName, c.customerName, b.branchName
FROM Invoice i
JOIN Employee e ON i.employeeNo = e.employeeNo
JOIN Customer c ON i.customerNo = c.customerNo
JOIN Branch b ON i.branchNo = b.branchNo;
- Invoice Master Detail View:
CREATE VIEW invoiceMasterDetail AS
SELECT i.invoiceNo, it.itemName, id.quantity, id.paidPrice, (id.quantity * id.paidPrice) AS totalPrice, it.itemNo
FROM InvoiceDetail id
JOIN Item it ON id.itemNo = it.itemNo;
Implement Transaction Management
1. Manage Transactions:
- Transaction Example:
BEGIN
-- Procedure code
COMMIT;
EXCEPTION
WHEN OTHERS THEN
ROLLBACK;
RAISE;
END;
- Using Savepoints:
BEGIN
SAVEPOINT sp1;
-- Code for first part of transaction
SAVEPOINT sp2;
-- Code for second part of transaction
COMMIT;
EXCEPTION
WHEN OTHERS THEN
ROLLBACK TO sp1;
DBMS_OUTPUT.PUT_LINE('Transaction failed and rolled back.');
END;
Securing the Database and Generating Reports
Database security ensures that your data is protected from unauthorized access and modifications. Generating reports helps in data analysis and documentation.
Apply Security Measures
1. Implement Security Triggers:
- Restrict Modifications on Weekends:
CREATE OR REPLACE TRIGGER no_dml_on_weekends
BEFORE INSERT OR UPDATE OR DELETE ON Invoice
FOR EACH ROW
BEGIN
IF TO_CHAR(SYSDATE, 'DY', 'NLS_DATE_LANGUAGE = ENGLISH') IN ('FRI', 'SAT') THEN
RAISE_APPLICATION_ERROR(-20001, 'Modifications are not allowed on Fridays and Saturdays.');
END IF;
END;
Generate Reports
1. Export Data to XML:
- Generate XML for Invoices:
SET LONG 1000000;
SET PAGESIZE 0;
SET LINESIZE 1000;
SPOOL invoices.xml;
SELECT dbms_xmlgen.getxml('SELECT * FROM invoiceMaster') FROM dual;
SPOOL OFF;
2. Optional XML for Detailed Report:
- Generate XML for Detailed Report:
SET LONG 1000000;
SET PAGESIZE 0;
SET LINESIZE 1000;
SPOOL detailed_invoices.xml;
SELECT dbms_xmlgen.getxml('SELECT * FROM invoiceMasterDetail') FROM dual;
SPOOL OFF;
Conclusion
Tackling complex SQL/PLSQL assignments involves a structured approach: designing a comprehensive database schema, implementing procedures and packages, managing transactions effectively, and ensuring database security. By following this guide, you can handle assignments such as developing a POS system with confidence, ensuring that your solution is well-designed, functional, and secure. Whether you are a student or a professional, mastering these techniques will enhance your SQL/PLSQL skills and prepare you for real-world challenges.