Combinatorial puzzles present a fascinating challenge, requiring a blend of logical thinking, mathematical reasoning, and programming skills. In this guide, we’ll explore a structured approach to solving combinatorial puzzles, with a specific focus on summation puzzles using recursion. Our goal is to provide you with a methodology that you can apply to a wide range of similar Programming assignments. This blog will offer the necessary steps and insights to effectively tackle these puzzles.
The essence of solving combinatorial puzzles lies in breaking down the problem into manageable parts and systematically exploring all possible solutions. With summation puzzles, the task is to assign digits to letters in such a way that a given arithmetic equation is satisfied. This requires both an understanding of the problem and an efficient way to explore potential solutions. Recursion offers a powerful tool for this purpose, as it allows you to explore each possible digit assignment step-by-step and backtrack when necessary.
First, identify the unique letters in the puzzle and understand that each letter needs to be mapped to a distinct digit. This creates a finite set of possible mappings to explore. Using recursion, you can generate these mappings and test each one to see if it solves the puzzle. The recursive function will try assigning each available digit to a letter, and then call itself with the next letter, continuing until all letters are assigned. If at any point the mapping does not work, the function backtracks by removing the last assigned digit and trying the next possibility.
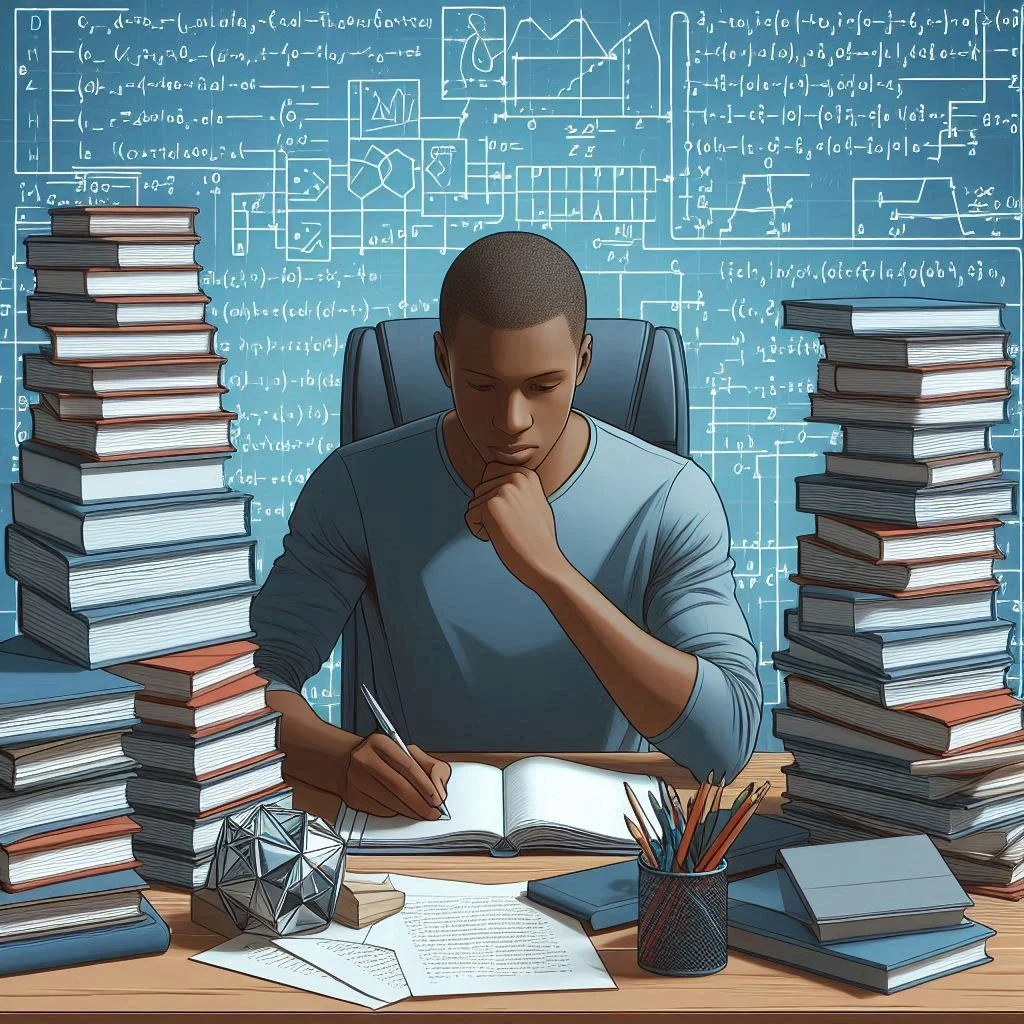
To ensure your recursive approach is effective, it is important to define a clear base case for the recursion to stop. This typically occurs when all letters have been assigned digits and the equation can be checked for validity. If the equation holds true with the current mapping, the solution is found. Otherwise, the function should continue exploring other mappings. This method not only ensures that all potential solutions are considered but also optimizes the process by discarding invalid paths early.
Efficient recursion also involves careful management of state. Keep track of used digits to avoid redundant checks and use a map to store the current digit assignment for each letter. This helps in quickly validating the current state of the puzzle and makes the code cleaner and more maintainable. Additionally, documenting your code and breaking down the solution into smaller functions can greatly improve readability and debugging.
Another critical aspect is handling edge cases and ensuring the solution adheres to constraints, such as not allowing digit reuse and managing large numbers. These constraints should be incorporated into your recursive logic to avoid incorrect solutions and infinite loops. Testing your solution with various test cases, from simple to complex, ensures robustness and helps identify any logical errors in your approach.
Understanding the Problem
Summation puzzles involve assigning distinct digits to letters in such a way that a given equation holds true. For example, given the strings POT + PAN = BIB, we need to find a digit mapping for each letter that makes the equation valid. Let’s break down the problem:
- Identify Letters: Extract all unique letters from the given strings.
- Generate Permutations: Try all possible combinations of digits for these letters.
- Validate the Solution: Check if the assigned digits satisfy the equation.
To achieve this, we’ll use recursion to explore all possible digit assignments efficiently.
Extracting Unique Letters
The first step is to extract all unique letters from the strings. This is essential because we need to map each unique letter to a distinct digit. Here’s a function to achieve that:
std::unordered_set
extractUniqueLetters(const std::string& s1, const std::string& s2, const std::string& s3) {
std::unordered_set
uniqueLetters;
for (char c : s1 + s2 + s3) {
uniqueLetters.insert(c);
}
return uniqueLetters;
}
This function concatenates the three input strings and inserts each character into an unordered set, ensuring each letter appears only once.
Setting Up the Recursive Function
Recursion is key to solving these puzzles. We’ll write a helper function that tries all possible mappings of digits to letters using recursion.
bool tryMappings(const std::string& s1, const std::string& s2, const std::string& s3,
std::unordered_set
& usedDigits, std::unordered_map& mapping,
const std::vector
& letters, int index) {
if (index == letters.size()) {
return isValidSolution(s1, s2, s3, mapping);
}
for (int digit = 0; digit <= 9; ++digit) {
if (usedDigits.find(digit) == usedDigits.end()) {
usedDigits.insert(digit);
mapping[letters[index]] = digit;
if (tryMappings(s1, s2, s3, usedDigits, mapping, letters, index + 1)) {
return true;
}
usedDigits.erase(digit);
mapping.erase(letters[index]);
}
}
return false;
}
< /char >
< /char, >
< /int >
This function works as follows:
- Base Case: If all letters are assigned (index == letters.size()), check if the current mapping satisfies the equation.
- Recursive Case: Try each digit (0-9) that hasn’t been used yet. Assign it to the current letter and proceed to the next letter. If a valid mapping is found, return true. Otherwise, backtrack by removing the digit from the used set and the current letter’s mapping.
Checking the Validity of the Solution
Once we have a potential mapping, we need to verify if it satisfies the given equation. Here’s a function to check the validity of a solution:
bool isValidSolution(const std::string& s1, const std::string& s2, const std::string& s3,
const std::unordered_map& mapping) {
auto strToNum = [&](const std::string& str) {
unsigned num = 0;
for (char c : str) {
num = num * 10 + mapping.at(c);
}
return num;
};
return strToNum(s1) + strToNum(s2) == strToNum(s3);
}
< /char, >
This function converts each string into a number based on the current mapping and checks if the sum of the first two numbers equals the third.
Integrating the Components
Now we can integrate these components into the main puzzle solver function:
bool puzzleSolver(const std::string& s1, const std::string& s2, const std::string& s3,
std::unordered_map& mapping) {
std::unordered_set
usedDigits;
auto uniqueLetters = extractUniqueLetters(s1, s2, s3);
std::vector
letters(uniqueLetters.begin(), uniqueLetters.end());
return tryMappings(s1, s2, s3, usedDigits, mapping, letters, 0);
}
< /char >
< /int >
< /char, >
This function extracts the unique letters, initializes the used digits set, and calls the recursive helper function.
Optimization Tips for Recursion
Efficiently implementing recursion is crucial for solving combinatorial puzzles within practical time limits. Here are some tips to optimize your recursive solution:
- Base Case: Ensure your base case is correct and stops recursion when all letters are mapped.
- Pruning: Eliminate impossible paths early by checking partial sums or constraints.
- Memorization: Store and reuse results of sub problems to avoid redundant calculations.
Writing Test Cases
Testing is an essential part of the development process. Write test cases to ensure your solution works correctly and efficiently. Here are some tips for testing:
- Start Small: Begin with simple puzzles to verify correctness.
- Include Edge Cases: Test puzzles with leading zeros, maximum digit constraints, and large inputs.
- Measure Performance: Ensure your solution runs within the specified time limits.
Example Test Cases
void runTests() {
std::unordered_map mapping;
// Test case 1: Simple valid puzzle
assert(puzzleSolver("POT", "PAN", "BIB", mapping));
// Mapping: P:2, O:3, T:1, A:7, N:4, B:5, I:0
// Test case 2: Puzzle with leading zeros
mapping.clear();
assert(puzzleSolver("UCI", "ALEX", "MIKE", mapping));
// Mapping: U:5, C:7, I:2, A:8, L:6, E:3, X:1, M:9, K:0
// Test case 3: Larger puzzle
mapping.clear();
assert(puzzleSolver("SEND", "MORE", "MONEY", mapping));
// Mapping: S:9, E:5, N:6, D:7, M:1, O:0, R:8, Y:2
}
< /char, >
These test cases check for basic functionality, handling of leading zeros, and performance with larger inputs.
Common Pitfalls and Troubleshooting
When working with combinatorial puzzles, you may encounter some common issues. Here’s how to troubleshoot them:
- Incorrect Base Case: Ensure your base case correctly stops recursion and returns the appropriate result.
- Digit Reuse: Make sure each digit is used only once. Use a set to track used digits.
- Overflow Issues: Although overflow is not a concern in this assignment, be mindful of it in general.
- Efficiency: If your solution is slow, try optimizing the recursive calls and pruning unnecessary paths.
Extending the Approach to Other Puzzles
The recursive approach outlined here can be extended to solve various other combinatorial puzzles, such as:
- Cryptarithms: Similar to summation puzzles but with different operations (e.g., multiplication, division).
- N-Queens Problem: Place N queens on an NxN chessboard such that no two queens attack each other.
- Sudoku: Fill a 9x9 grid with digits so that each column, each row, and each of the nine 3x3 subgrids contain all digits from 1 to 9.
By adapting the recursive methodology, you can tackle a wide range of combinatorial problems.
Practice Problems
To further hone your skills, practice with the following problems:
- Verbal Arithmetic Puzzles: Solve puzzles like "SEND + MORE = MONEY".
- Magic Squares: Arrange numbers in a grid so that the sums of the numbers in each row, column, and diagonal are the same.
- Knight’s Tour: Find a sequence of moves for a knight on a chessboard such that it visits every square exactly once.
These problems will help you develop a deeper understanding of recursion and combinatorial problem-solving.
Conclusion
Solving combinatorial puzzles using recursion is both challenging and rewarding. By breaking down problems into smaller components and using recursion effectively, you can develop robust solutions. Practice regularly with different puzzles to enhance your problem-solving skills and deepen your understanding of recursion.
The essence of recursive problem-solving is in dividing a complex problem into manageable sub-problems. This method allows you to focus on one part at a time while recursion handles the rest. Backtracking is crucial in this approach, as it enables you to explore all possible solutions systematically, ensuring no potential answers are overlooked.
Developing effective recursive solutions requires identifying appropriate base cases and termination conditions to prevent infinite loops and ensure convergence. With practice, you’ll learn to set these up efficiently, building a strong foundation for more complex challenges.
Optimization is also key. Recursion can lead to performance issues, so it’s important to optimize your functions to reduce unnecessary computations. Techniques like memoization, which stores results of expensive calls for reuse, can significantly improve efficiency.
Engaging with combinatorial puzzles hones your mathematical reasoning and logical thinking, making you proficient in solving a wide range of problems. Keep experimenting, learning, and refining your approach to become adept at tackling these intriguing challenges.