Programming assignments, especially those involving intricate data structures like Merkle Trees, can be intimidating. However, with the right approach, these assignments can become manageable and even enjoyable. This blog will guide you through a methodical approach to solving complex programming assignments, using the example of "Merkle Trees Mapping to Dynamic Arrays." Although we will refer to this specific assignment, the strategies discussed are broadly applicable to a wide range of similar programming tasks.
By breaking down the problem into smaller, manageable tasks, planning your approach carefully, and setting up your development environment properly, you can tackle complex problems effectively. Implementing your solution incrementally, writing unit tests, and optimizing your code as you go along will help ensure that your solution is robust and efficient. Additionally, rigorous testing and debugging will be crucial to identify and fix any issues that arise.
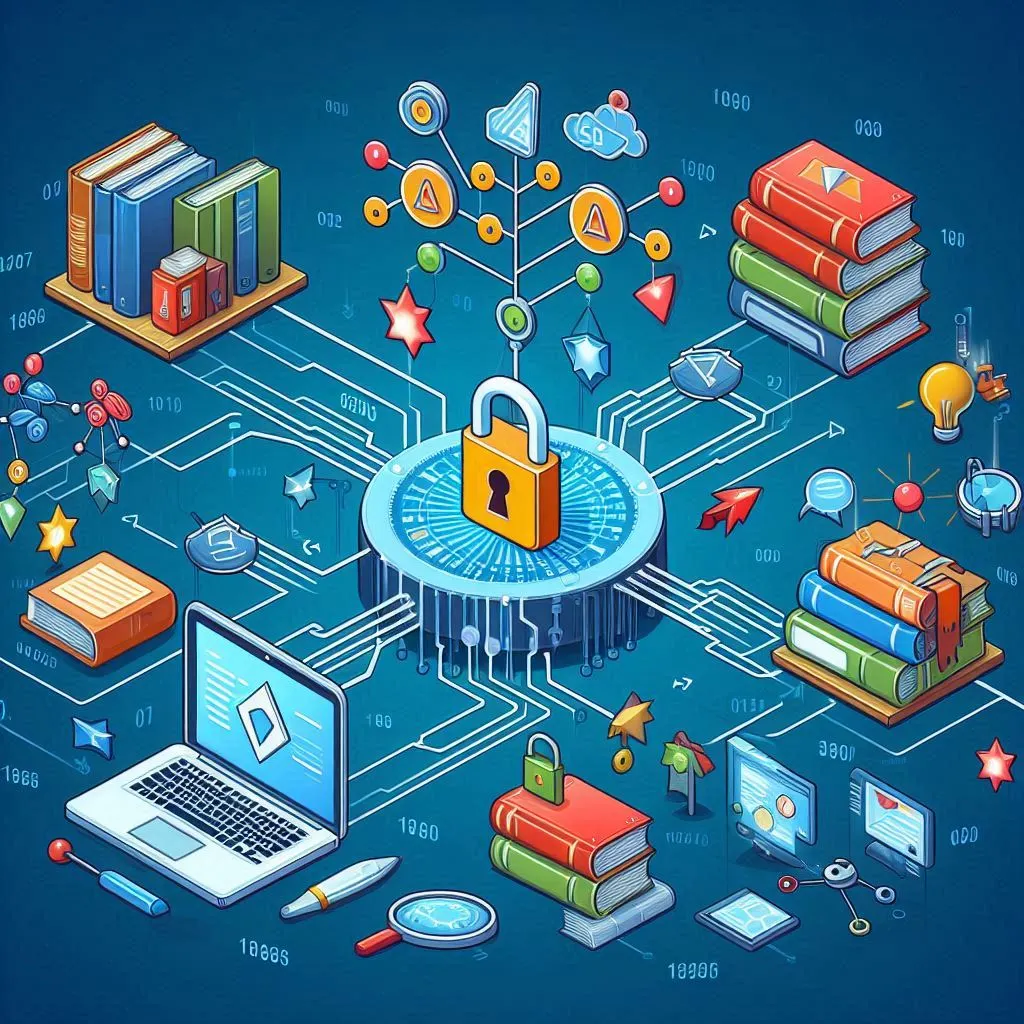
For those seeking additional support, Java assignment help services can provide valuable assistance. These services offer guidance and support to help you complete your assignments with confidence and accuracy. With the right resources and approach, mastering complex data structures like Merkle Trees becomes a more achievable goal.
Understanding the Problem Statement
Before diving into coding, it’s essential to thoroughly understand the problem you’re solving. Many students make the mistake of jumping straight into writing code without fully grasping the requirements, leading to wasted time and frustration.
- Read the Assignment Carefully: Start by reading the entire assignment prompt several times. Pay attention to every detail, including the requirements, restrictions, and the overall objective of the assignment. For example, in the case of Merkle Trees Mapping to Dynamic Arrays, you’re not only asked to implement a specific data structure but also to ensure that it meets certain performance criteria and integrates with existing code in a particular way.
- Break down the Problem: Once you understand the overall goal, break down the problem into smaller, more manageable tasks. For instance, in the Merkle Tree assignment, you could break it down into the following tasks:
- Implement the basic structure of a Merkle Tree.
- Implement the hashing mechanism.
- Map the Merkle Tree to a dynamic array.
- Implement the integrity verification algorithm.
- Clarify Any Doubts: If you find any part of the assignment unclear, don’t hesitate to ask your instructor or classmates for clarification. It’s better to spend a few minutes asking questions than to waste hours working on the wrong solution.
Planning Your Approach
Planning is crucial for tackling complex assignments effectively. A well-thought-out plan will save you time and help you avoid common pitfalls.
- Identify the Key Components: After breaking down the problem, identify the key components you need to implement. For the Merkle Tree assignment, these might include:
- Node creation and manipulation.
- Hash function implementation.
- Dynamic array conversion.
- Integrity verification.
- Determine the Order of Implementation: Decide in what order you will implement the components. Typically, it’s best to start with the most fundamental parts and build up to the more complex features. For instance, you would first implement the Merkle Tree structure before working on mapping it to a dynamic array.
- Create a Timeline: Estimate how much time you will need to complete each component and create a timeline for your work. Be realistic about how much time you have available, and try to build in some buffer time in case you encounter unexpected challenges.
- Write Pseudocode: Before you start coding, write pseudocode for the key functions and algorithms. Pseudocode allows you to outline your logic without worrying about syntax, making it easier to spot potential issues early on. For example, you might write pseudocode for the Merkle Tree’s hash calculation function or the dynamic array conversion process.
Setting Up Your Development Environment
Having the right tools and environment set up before you begin coding is crucial for efficient programming.
- Choose Your IDE: Select an Integrated Development Environment (IDE) that you are comfortable with and that supports the language you are using. For Java, popular choices include IntelliJ IDEA, Eclipse, and NetBeans.
- Install Necessary Libraries: Ensure that all necessary libraries and dependencies are installed. For the Merkle Tree assignment, you might need libraries for cryptographic functions, such as java.security.MessageDigest for SHA-256 hashing.
- Set Up Version Control: Use a version control system like Git to keep track of your changes. This will allow you to revert to previous versions if something goes wrong. You can also use platforms like GitHub or Bitbucket to store your code online.
- Configure the Style Checker: Since the assignment requires adherence to specific style guidelines, configure your IDE to automatically check your code style. Many IDEs allow you to install plugins that will highlight style violations as you code.
- Prepare the Readme File: The assignment mentions a readme.txt file that needs to be filled out with your information. This is often overlooked, but it’s important to set up early to ensure all required information is accurately recorded.
Implementing the Solution
Now that you have a plan and your environment is set up, it’s time to start coding.
- Start Small and Test Often: Begin by implementing the smallest, most fundamental components of your solution. For example, you might start by writing a basic MerkleTreeNode class that can hold data and references to parent and child nodes. Once this is done, test it thoroughly before moving on.
- Build Incrementally: Add new features gradually, testing each one before moving on to the next. For instance, after implementing the basic node structure, you might add the hashing function, then test it with a few simple inputs to ensure it produces the correct output.
- Write Unit Tests: Writing unit tests is crucial for ensuring that each component of your code works as expected. For the Merkle Tree assignment, you could write tests for the hash function, the tree construction, and the dynamic array conversion. Use a testing framework like JUnit to automate your tests and make it easier to run them frequently.
- Optimize as You Go: Pay attention to the performance requirements specified in the assignment. If a method is required to run in O(log n) time, ensure that your implementation meets this requirement. Optimize your code as you go along, rather than waiting until the end.
- Comment Your Code: As you write your code, include detailed comments in JavaDoc style. This will not only help you pass the style checker but also make your code easier to understand when you revisit it later.
Testing and Debugging
Testing is a critical part of the development process, especially for complex assignments like this one.
- Run Your Tests Regularly: As mentioned earlier, write unit tests for each component and run them frequently. This will help you catch bugs early, before they become more difficult to fix.
- Use a Debugger: Most IDEs come with built-in debuggers that allow you to step through your code line by line. This can be incredibly helpful for tracking down elusive bugs. For example, if your Merkle Tree isn’t producing the correct hash values, you can use the debugger to inspect the state of the tree at each step of the calculation.
- Test Edge Cases: Be sure to test your code with edge cases, such as an empty tree or a tree with only one node. These cases can often reveal bugs that wouldn’t appear with more typical input.
- Validate against the Problem Statement: Continuously validate your solution against the problem statement to ensure you’re meeting all the requirements. For instance, the assignment specifies that you can only use the ArrayList data structure from java.util. Make sure your code adheres to this restriction.
- Handle Errors Gracefully: Implement proper error handling to manage unexpected situations without crashing the program. For example, if the integrity verification algorithm detects that a file has been tampered with, the program should halt the operation and provide a meaningful error message to the user.
Finalizing and Submitting Your Assignment
Once your solution is complete and thoroughly tested, it’s time to prepare for submission.
- Review the Submission Requirements: Go back to the assignment prompt and carefully review the submission instructions. Ensure that you have followed all the guidelines, such as filling out the readme.txt file, and removing any unnecessary files before submission.
- Clean Up Your Code: Before submitting, clean up your code by removing any commented-out sections or debugging statements. Double-check that your code adheres to the required style guidelines and that all comments are in JavaDoc format.
- Create the Submission Package: Follow the instructions for packaging your submission. For example, the assignment might require you to zip your user folder with a specific naming convention. Make sure you follow these instructions precisely to avoid losing points on technicalities.
- Backup Your Work: Before submitting, create a backup of your final solution. This ensures that you have a copy in case something goes wrong with the submission process.
- Submit on Time: Make sure you submit your assignment before the deadline. Late submissions can often result in penalties or even a zero grade, so it’s important to manage your time effectively and avoid last-minute issues.
Reflecting on Your Work
After you’ve submitted your assignment, take some time to reflect on what you’ve learned.
- Review Your Approach: Think about the strategies you used and consider what worked well and what didn’t. For example, did your initial plan hold up, or did you have to make significant changes along the way? Understanding what worked can help you refine your approach for future assignments.
- Learn from Mistakes: If you encountered any difficulties or made mistakes, take note of them so you can avoid repeating them in the future. For instance, if you struggled with the performance requirements, consider spending more time studying algorithm optimization.
- Seek Feedback: If possible, seek feedback from your instructor or peers. Constructive criticism can help you improve your skills and approach for future assignments.
- Build on Your Knowledge: Finally, think about how you can apply what you’ve learned to other assignments or projects. The concepts you’ve mastered in this assignment, such as data structures, cryptography, and performance optimization, are likely to be useful in many other areas of computer science.
Conclusion
Tackling complex programming assignments like Merkle Trees Mapping to Dynamic Arrays can be challenging, but with the right approach, it’s entirely manageable. By thoroughly understanding the problem, planning your approach, setting up your environment, and methodically implementing, testing, and refining your solution, you can successfully complete even the most daunting assignments. Remember that every assignment is an opportunity to learn and grow as a programmer, so take the time to reflect on what you’ve accomplished and how you can apply these lessons in the future.