Memory management is a fundamental aspect of computer science that plays a critical role in ensuring efficient and effective use of system resources. Understanding how to allocate, deallocate, and reallocate memory is essential for developing robust programs. This guide will help students approach memory management assignments, providing the foundational knowledge and practical steps needed to tackle similar tasks.
In solving programming assignments, particularly those involving C, memory management can often be a challenging area. Proper memory allocation is crucial to prevent issues such as memory leaks and segmentation faults, which can significantly impact the performance and reliability of your programs. Efficient memory management ensures that applications run smoothly and utilize system resources effectively.
When availing C assignment help services, it's important to understand the key components of memory management: allocation, deallocation, and reallocation. Allocation involves reserving a portion of memory for use, deallocation involves releasing the reserved memory when it is no longer needed, and reallocation involves adjusting the size of an allocated memory block.
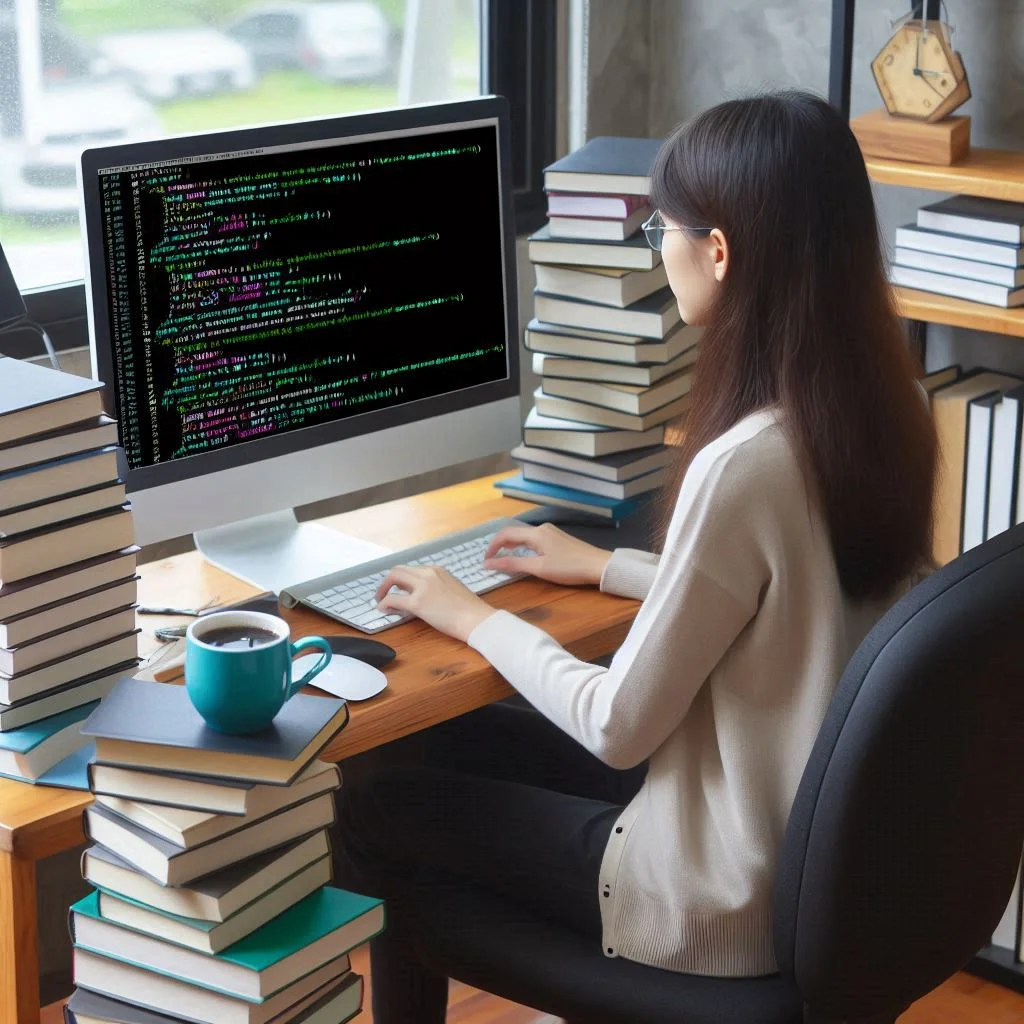
Understanding Memory Management
Memory management involves the allocation, deallocation, and reallocation of memory during the execution of a program. Efficient memory management ensures that applications run smoothly and utilize system resources effectively. The key components of memory management include:
- Allocation: Reserving a portion of memory for use.
- Deallocation: Releasing the reserved memory when it is no longer needed.
- Reallocation: Adjusting the size of an allocated memory block.
Memory Allocation
Overview
Memory allocation is the process of reserving a portion of memory during program execution. Various algorithms handle memory allocation, with "First Fit" being a commonly used method. In this algorithm, the allocator searches for the first block of memory that is large enough to satisfy the request.
Steps for Implementation
1. Define a Memory Block Structure: Create a struct to represent each block of memory. This struct should contain information about the block's size, whether it is free or allocated, and pointers to the next and previous blocks in the linked list.
typedef struct Block {
size_t size;
int free;
struct Block *next;
} Block;
2. Initialize Memory: Set up a function to initialize the memory allocator. This function should create a large block of memory and set up the linked list structure.
void init_memory(size_t size) {
head = (Block *)sbrk(size + sizeof(Block));
head->size = size;
head->free = 1;
head->next = NULL;
}
3. Allocate Memory: Implement the allocation function that iterates through the linked list to find the first suitable block.
void *mm_malloc(size_t size) {
Block *current = head;
while (current) {
if (current->free && current->size >= size) {
// Split the block if necessary
if (current->size > size + sizeof(Block)) {
Block *new_block = (Block *)((char *)current + sizeof(Block) + size);
new_block->size = current->size - size - sizeof(Block);
new_block->free = 1;
new_block->next = current->next;
current->size = size;
current->next = new_block;
}
current->free = 0;
return (char *)current + sizeof(Block);
}
current = current->next;
}
return NULL; // No suitable block found
}
Memory Deallocation
Overview
Deallocation is the process of marking a block of memory as free for future use. Handling fragmentation is crucial. Fragmentation occurs when free memory is scattered in small blocks, making it difficult to allocate larger blocks.
Steps for Implementation
1. Free Memory Block: Implement the function to mark a block as free and coalesce adjacent free blocks.
void mm_free(void *ptr) {
if (!ptr) return;
Block *current = (Block *)((char *)ptr - sizeof(Block));
current->free = 1;
// Coalesce adjacent free blocks
Block *next = current->next;
while (next && next->free) {
current->size += next->size + sizeof(Block);
current->next = next->next;
next = next->next;
}
}
Memory Reallocation
Overview
Reallocation adjusts the size of an allocated block. It typically involves allocating a new block of the requested size, copying the old data to the new block, and then freeing the old block.
Steps for Implementation
1. Reallocate Memory Block: Implement the function to resize a block, considering various edge cases.
void *mm_realloc(void *ptr, size_t size) {
if (size == 0) {
mm_free(ptr);
return NULL;
}
if (!ptr) {
return mm_malloc(size);
}
Block *current = (Block *)((char *)ptr - sizeof(Block));
if (current->size >= size) {
// If the new size is smaller or equal, no need to move the block
return ptr;
}
void *new_ptr = mm_malloc(size);
if (!new_ptr) return NULL;
memcpy(new_ptr, ptr, current->size);
mm_free(ptr);
return new_ptr;
}
Additional Tips for Successful Implementation
1. Zero-Fill Allocated Memory: For security and grading purposes, zero-fill the allocated memory before returning a pointer.
void *mm_malloc(size_t size) {
void *ptr = allocate_memory(size);
if (ptr) {
memset(ptr, 0, size);
}
return ptr;
}
2. Handle Edge Cases: Ensure your functions handle cases like zero-size allocation, NULL pointers, and allocation failures gracefully.
if (size == 0) {
return NULL;
}
3. Testing and Validation: Thoroughly test your allocator with various scenarios, including allocating, freeing, and reallocating memory of different sizes. Check for memory leaks and fragmentation issues.
void test_allocator() {
void *ptr1 = mm_malloc(100);
void *ptr2 = mm_malloc(200);
mm_free(ptr1);
void *ptr3 = mm_malloc(50);
ptr2 = mm_realloc(ptr2, 300);
mm_free(ptr2);
mm_free(ptr3);
}
Best Practices for Memory Management Assignments
- Understand the Requirements: Carefully read the assignment prompt and understand what is required. Identify the specific memory management techniques you need to implement.
- Plan Your Approach: Before writing code, outline the structure of your memory allocator. Decide how you will implement the linked list, handle allocation, deallocation, and reallocation, and manage edge cases.
- Break Down the Problem: Divide the assignment into smaller tasks, such as implementing the memory block structure, creating the initialization function, and writing the allocation, deallocation, and reallocation functions.
- Write Clean and Modular Code: Keep your code organized and modular. Use functions to encapsulate different parts of the memory management process. This makes your code easier to read, debug, and maintain.
- Test Thoroughly: Create a comprehensive set of test cases to validate your memory allocator. Test various allocation sizes, deallocation scenarios, and reallocation cases. Check for memory leaks and fragmentation.
- Document Your Code: Add comments to explain your code. Document the purpose of each function, describe the parameters and return values, and provide an overview of how your memory allocator works.
- Seek Feedback: If possible, get feedback from peers or instructors. Review your code and identify areas for improvement. Make necessary adjustments based on the feedback.
Example Assignment Walkthrough
Let's walk through an example assignment related to memory allocation, deallocation, and reallocation. This example will provide a step-by-step guide to help you approach similar assignments.
Assignment: Implement a Memory Allocator
Task: Implement a memory allocator using a linked list of memory blocks. The allocator should handle memory allocation, deallocation, and reallocation.
Requirements:
- Allocation function: void *mm_malloc(size_t size)
- Deallocation function: void mm_free(void *ptr)
- Reallocation function: void *mm_realloc(void *ptr, size_t size)
Steps:
1. Define the Memory Block Structure: Create a struct to represent each block of memory.
typedef struct Block {
size_t size;
int free;
struct Block *next;
} Block;
2. Initialize Memory: Implement a function to initialize the memory allocator.
void init_memory(size_t size) {
head = (Block *)sbrk(size + sizeof(Block));
head->size = size;
head->free = 1;
head->next = NULL;
}
3. Allocate Memory: Implement the allocation function.
void *mm_malloc(size_t size) {
Block *current = head;
while (current) {
if (current->free && current->size >= size) {
if (current->size > size + sizeof(Block)) {
Block *new_block = (Block *)((char *)current + sizeof(Block) + size);
new_block->size = current->size - size - sizeof(Block);
new_block->free = 1;
new_block->next = current->next;
current->size = size;
current->next = new_block;
}
current->free = 0;
return (char *)current + sizeof(Block);
}
current = current->next;
}
return NULL; // No suitable block found
}
4. Deallocate Memory: Implement the deallocation function.
void mm_free(void *ptr) {
if (!ptr) return;
Block *current = (Block *)((char *)ptr - sizeof(Block));
current->free = 1;
// Coalesce adjacent free blocks
Block *next = current->next;
while (next && next->free) {
current->size += next->size + sizeof(Block);
current->next = next->next;
next = next->next;
}
}
5. Reallocate Memory: Implement the reallocation function.
void *mm_realloc(void *ptr, size_t size) {
if (size == 0) {
mm_free(ptr);
return NULL;
}
if (!ptr) {
return mm_malloc(size);
}
Block *current = (Block *)((char *)ptr - sizeof(Block));
if (current->size >= size) {
return ptr;
}
void *new_ptr = mm_malloc(size);
if (!new_ptr) return NULL;
memcpy(new_ptr, ptr, current->size);
mm_free(ptr);
return new_ptr;
}
Testing and Validation
To ensure the correctness of your memory allocator, create a comprehensive set of test cases. These tests should cover various scenarios, including allocating different sizes of memory, freeing memory, and reallocating memory to larger and smaller sizes.
void test_allocator() {
void *ptr1 = mm_malloc(100);
void *ptr2 = mm_malloc(200);
mm_free(ptr1);
void *ptr3 = mm_malloc(50);
ptr2 = mm_realloc(ptr2, 300);
mm_free(ptr2);
mm_free(ptr3);
}
Conclusion
Mastering memory management is essential for developing efficient and reliable programs. By understanding the principles of memory allocation, deallocation, and reallocation, and following a structured approach, you can effectively tackle memory management assignments. This guide provides a comprehensive framework to help you succeed in your memory management tasks, ensuring that you develop robust and efficient solutions. Remember to thoroughly test your allocator and seek feedback to continually improve your skills.