When tackling programming assignments that focus on authentication and authorization, a solid understanding of the underlying principles and their accurate implementation is crucial. These assignments often build upon previous work and introduce new complexities, such as additional status codes, headers, and security mechanisms. Mastering these elements is essential for developing secure and functional systems. If you need help with programming assignments related to authentication and authorization, understanding these principles thoroughly will guide you in creating effective solutions.
Authentication and authorization are foundational to security in web applications. Authentication verifies the identity of a user or system, ensuring that the entity requesting access is who it claims to be. Common methods include Basic Authentication and Digest Authentication. On the other hand, authorization determines what actions or resources an authenticated user or system is permitted to access, often involving the management of permissions and access control lists.
Understanding Authentication and Authorization
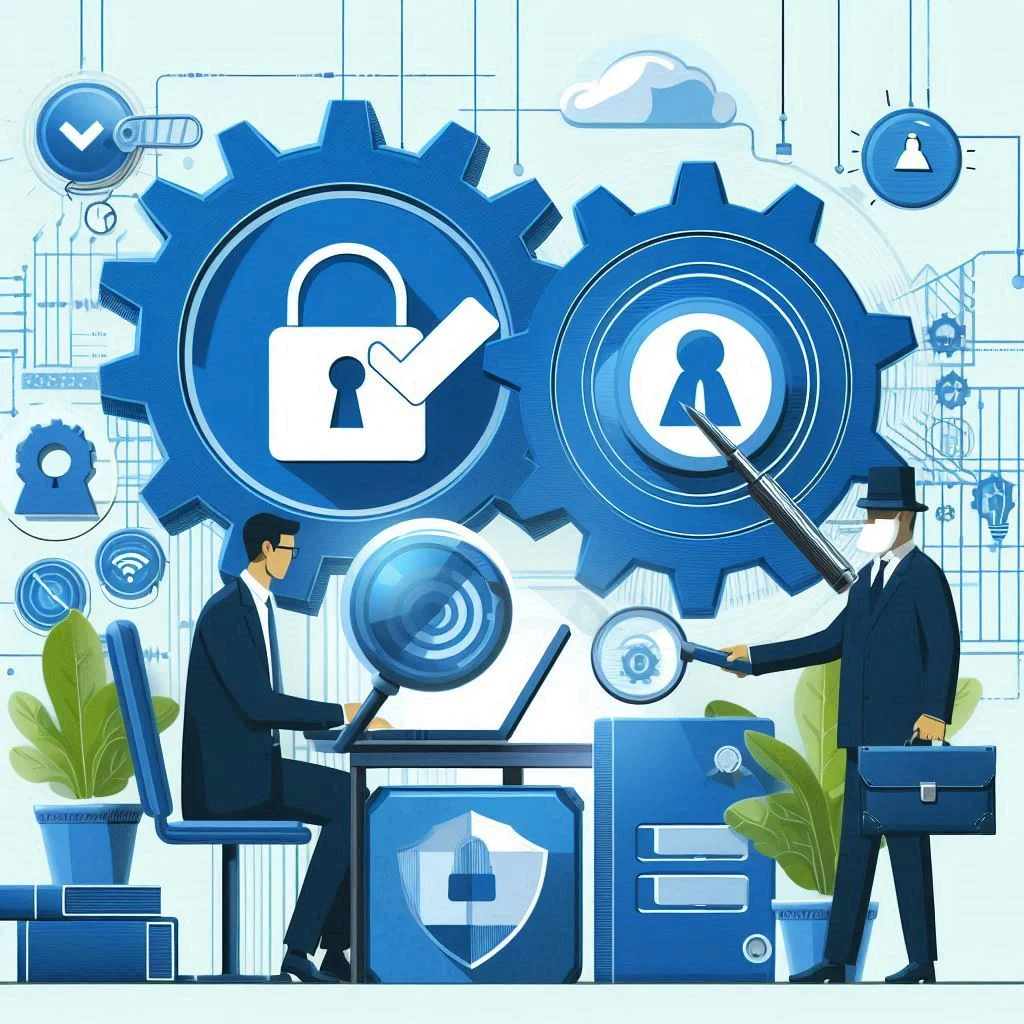
Authentication and authorization are two fundamental concepts in security, each serving a distinct purpose:
- Authentication: This process involves verifying the identity of a user or system. It ensures that the entity requesting access is who it claims to be. Common methods include Basic Authentication and Digest Authentication.
- Authorization: Once a user or system is authenticated, authorization determines what actions or resources they are permitted to access. This often involves managing permissions and access control lists.
Core Concepts of Authentication
Basic Authentication
Basic Authentication is a straightforward method where credentials are transmitted as a base64-encoded string. While it is simple and easy to implement, it should always be used in conjunction with HTTPS to encrypt the transmitted data and protect against eavesdropping.
Implementation Steps:
- Request Handling: The client sends credentials in the Authorization header as Basic base64 (username: password).
- Server Validation: The server decodes the base64 string, retrieves the username and password, and validates them against a stored list or database.
- Response: If credentials are valid, access is granted; otherwise, a 401 Unauthorized response is returned.
Digest Authentication
Digest Authentication is more secure than Basic Authentication as it uses hashing to protect credentials. It involves creating a hashed response based on the credentials, a nonce (a unique value for each request), and other parameters to prevent replay attacks.
Implementation Steps:
- Challenge Response: The server issues a challenge with a 401 Unauthorized response, including a WWW-Authenticate header specifying the authentication scheme.
- Client Response: The client responds with a hashed value in the Authorization header, which includes the username, nonce, and other details.
- Server Verification: The server hashes the credentials and compares them with the received hash to validate the request.
Key Components and Configuration
Server Configuration
In assignments involving authentication and authorization, server configuration is crucial. Here’s what you need to do:
- Private Key: Define a private key for generating nonce and opaque values. While using a simple string for learning purposes is acceptable, it is important to use a more secure method in production environments.
- Directory Protection: Configure how directories and sub-directories are protected. You might use a default name like WeMustProtectThisHouse! to ensure that unauthorized users cannot access protected areas.
Handling Request and Response Headers
- Authorization Header: This header is used by clients to pass authentication credentials to the server. It can contain different schemes like Basic or Digest.
- WWW-Authenticate Header: Sent by the server to indicate that authentication is required and specify the authentication scheme.
- Authorization-Info Header: Optionally included to provide additional details about the authorization context or requirements.
Implementing Authentication and Authorization
Basic Authentication
Step-by-Step Implementation:
- Set Up the Server: Configure the server to handle Authorization headers and decode them to extract credentials.
- Validate Credentials: Compare the decoded credentials with a stored list or database to authenticate the user.
- Respond Appropriately: If authentication is successful, grant access; otherwise, return a 401 unauthorized status with the WWW-Authenticate header.
Code Example:
from flask import Flask, request, Response
app = Flask(__name__)
@app.route('/protected')
def protected():
auth = request.authorization
if auth and auth.username == 'user' and auth.password == 'pass':
return "Welcome to the protected area!"
return Response(
'Could not verify your login!',
401,
{'WWW-Authenticate': 'Basic realm="Login Required"'}
)
if __name__ == '__main__':
app.run()
Digest Authentication
Step-by-Step Implementation:
- Generate a Nonce: The server creates a nonce for each request to prevent replay attacks.
- Challenge the Client: Send a 401 unauthorized response with a WWW-Authenticate header that includes the nonce and other parameters.
- Validate the Response: Check the client’s response by hashing the credentials and comparing it with the received hash.
Code Example:
Implementing Digest Authentication requires more complex logic involving hashing and nonces, which is beyond a simple code snippet. For full implementation, refer to the RFCs and specific libraries that handle Digest Authentication.
Working with Status Codes and Headers
- 401 Unauthorized: Ensure your server correctly responds with this status code when authentication fails. Include the WWW-Authenticate header to specify the required authentication method.
- WWW-Authenticate Header: This header informs the client of the authentication scheme required. For Basic Authentication, it might look like WWW-Authenticate: Basic realm="Login required".
- Authorization-Info Header: If used, this header provides additional information about the authorization context. It is not commonly required but can be useful in certain scenarios.
Testing and Submission
- Create Sample Test Files: Prepare sample test files to validate your implementation. Place them in the document root of your web server for testing.
- Dockerfile Setup: Ensure your Dockerfile is configured to run your server on port 80 by default. This configuration helps streamline deployment and testing processes.
- Tagging and Submitting: Tag your release appropriately (e.g., a4) and follow submission guidelines. Ensure that your code is organized and adheres to the required structure for evaluation.
Tips for Success
- Follow RFCs: Adhering to relevant RFCs (e.g., RFC 7617 for Basic Authentication and RFC 7616 for Digest Authentication) ensures compliance with standards and proper implementation.
- Security Best Practices: While a simple string for nonce and opaque values might be acceptable for learning purposes, always use secure methods in real-world applications.
- Modular Code: Even if the assignment requires all functions to be in a single file for simplicity, consider separating concerns into different modules in your personal practice.
- Documentation: Document your code and configuration thoroughly to make it easier for others (and yourself) to understand, maintain, and debug.
Conclusion
By mastering the principles of authentication and authorization, you will enhance your ability to tackle a wide range of programming assignments and real-world applications. Following these guidelines will help you implement secure and effective authentication mechanisms, manage permissions, and ensure your solutions are robust and reliable. Remember, the skills you develop in these assignments are foundational for creating secure and efficient systems in the future. Happy coding!