Programming assignments involving vectors and maps in C++ are designed to assess your understanding of these fundamental data structures and your ability to manipulate them effectively. These assignments often require you to use vectors and maps to solve problems, making it essential to have a solid grasp of how these data structures work.
In this blog, we will delve into strategies for tackling C++ assignments involving vectors and maps. We’ll provide detailed guidance, practical examples, and step-by-step solutions to help you navigate these tasks successfully. By the end of this post, you will have a clear understanding of how to approach similar programming challenges, enhancing your problem-solving skills in C++.
Just as with other complex subjects, having a structured approach to C++ assignments is crucial. If you find yourself struggling, remember that seeking C++ assignment help can make a significant difference. Professional assistance can guide you through challenging problems and ensure you meet your academic goals
Understanding the Assignment Requirements
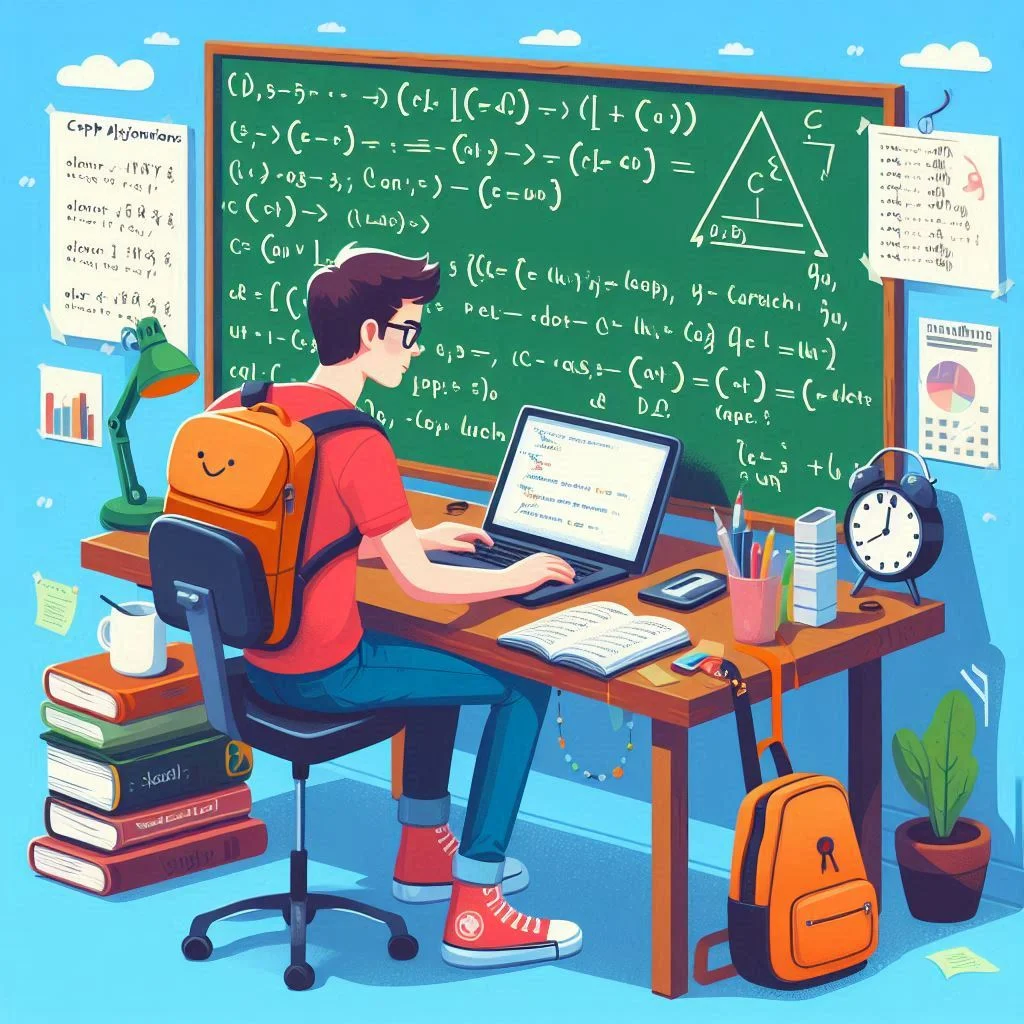
Before diving into the coding process, it’s crucial to thoroughly understand the assignment requirements. Let’s break down a typical assignment involving vectors and maps to illustrate the process.
Assignment Example:
1. Objective: You are required to implement functions that work with vectors and maps. Specifically:
- Part 1 (Vector): Write a function to insert a value at a specified index in a C++ vector using iterators.
- Part 2 (Map): Write a function to convert a vector of strings into a map where each key is a string and its value is the number of times the string appears in the vector.
2. Grading Criteria: Pay attention to the documentation header requirements, the use of concepts taught in the course, and the proper implementation of vectors and maps.
Key Concepts to Review
To effectively complete assignments involving vectors and maps, ensure you have a solid grasp of the following concepts:
Vectors
- Dynamic Array: Vectors are dynamic arrays that can grow and shrink in size. They are part of the C++ Standard Library and provide numerous methods for element manipulation.
- Common Methods: Key methods include push_back for adding elements, insert for inserting elements at specific positions, and iterators for traversing elements.
- Iterators: Iterators are used to access elements in a vector and perform operations like insertion and deletion. They provide a way to traverse containers in a standardized manner.
Maps
- Associative Container: Maps store elements as key-value pairs. Each key in the map is unique, and each key maps to a specific value.
- Common Operations: Essential operations include inserting key-value pairs, accessing values by keys, and iterating over map entries.
- Iteration: Maps can be iterated using iterators to access and manipulate key-value pairs.
Step-by-Step Solution Approach
Let’s break down the approach to solving each part of the assignment:
Part 1: Working with Vectors
Objective: Implement a function called insertNth that inserts a value at a specified index in a vector using iterators.
Steps:
- Define the Function: Create a template function insertNth that can work with any data type. The function should accept a reference to a vector, an index, and a value to be inserted.
- Check Index Validity: Ensure that the index is within the valid range of the vector. If the index is invalid, return false. Otherwise, proceed with the insertion.
- Insert Value: Use the vector's insert method to place the value at the given index. The insert method takes an iterator pointing to the insertion position and the value to be inserted.
- Return Status: Return true if the insertion was successful, and false if the index was invalid.
Example Code:
#include < iostream >
#include < vector >
template
bool insertNth(std::vector
& vec, int index, T value) {
// Check if the index is within bounds
if (index >= 0 && index <= vec.size()) {
// Create an iterator pointing to the insertion position
auto it = vec.begin() + index;
// Insert the value at the specified index
vec.insert(it, value);
return true;
}
return false; // Index is out of range
}
int main() {
std::vector
vec = {1, 2, 3, 4, 5};
// Test insertion with valid index
if (insertNth(vec, 2, 99)) {
std::cout << "Insertion successful. Vector contents: ";
for (int i : vec) std::cout << i << " ";
std::cout << std::endl;
} else {
std::cout << "Insertion failed. Index out of range." << std::endl;
}
// Test insertion with invalid index
if (!insertNth(vec, 10, 77)) {
std::cout << "Insertion failed. Index out of range." << std::endl;
}
return 0;
}
Explanation:
- The insertNth function takes a vector, an index, and a value. It checks if the index is valid and uses an iterator to insert the value at the specified position.
- In main(), the function is tested with both valid and invalid indices to ensure it works correctly.
Part 2: Working with Maps
Objective: Implement a function called vectorToMap that converts a vector of strings into a map where each key is a string, and its value is the number of occurrences in the vector.
Steps:
- Define the Function: Create a function vectorToMap that accepts a vector of strings and returns a map with the frequency of each string.
- Iterate Over Vector: Use a loop to traverse the vector and update the map. For each string in the vector, increment its count in the map.
- Update Map: Use the map's key-value pairs to store each string as a key and its frequency as the value.
Example Code:
#include < iostream >
#include < vector >
#include < map >
#include < string >
std::map vectorToMap(const std::vector
& vec) {
std::map freqMap;
// Iterate over each string in the vector
for (const auto& str : vec) {
// Increment the count for each string
++freqMap[str];
}
return freqMap;
}
int main() {
std::vector
words = {"roses", "red", "violets", "blue", "red", "roses", "blue", "red"};
std::map resultMap = vectorToMap(words);
// Print the resulting map
for (const auto& pair : resultMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Explanation:
- The vectorToMap function creates a map and iterates through the vector to count the frequency of each string.
- The main() function demonstrates how to use vectorToMap and prints the resulting map.
Testing and Debugging
Testing: Thoroughly test your functions to ensure they work as expected:
- Vectors: Test the insertNth function with various valid and invalid indices. Check how the function handles different data types, such as integers, doubles, and strings.
- Maps: Test the vectorToMap function with different vectors of strings. Verify that the map correctly counts occurrences of each string.
Debugging:
- Print Statements: Use print statements to display intermediate results and verify that your functions are working correctly.
- Breakpoints: Utilize debugging tools to set breakpoints and inspect the values of variables at different stages of execution.
- Edge Cases: Consider edge cases, such as empty vectors, large vectors, and vectors with duplicate strings, to ensure your functions handle all scenarios.
Documentation and Submission
Documentation:
- Comments: Add comments to explain the purpose and functionality of your code. This helps others (and yourself) understand the logic behind your implementation.
- Documentation Headers: Include a documentation header at the beginning of each file as specified by your course guidelines. This should include information such as your name, assignment number, and a brief description of the code.
Example Documentation Header:
/*
* Filename: lastname_firstname_assn10.cpp
* Author: Your Name
* Assignment: Assignment 10
* Date: YYYY-MM-DD
* Description: This program demonstrates the use of vectors and maps in C++.
* It includes functions for inserting values into vectors and
* converting vectors to maps with frequency counts.
*/
Submission:
- File Naming: Follow the file naming convention specified in the assignment. Ensure your file is named appropriately, such as lastname_firstname_assn10.cpp.
- Submission Guidelines: Adhere to any additional submission guidelines provided by your instructor. This may include formatting requirements or specific instructions for uploading your work.
Best Practices
- Write Clean Code: Ensure your code is well-organized and easy to read. Use meaningful variable names and follow consistent coding standards.
- Optimize Performance: While not always required, consider optimizing your code for better performance, especially for large inputs.
- Understand the Concepts: Make sure you understand the underlying concepts of vectors and maps, as this will help you solve similar problems in the future.
Conclusion
Programming assignments involving vectors and maps are an excellent way to test and improve your skills in C++. By following the step-by-step approach outlined in this blog, you can systematically solve similar assignments and build a solid foundation in data structures. Remember to thoroughly test your code, document your work, and adhere to submission guidelines to achieve the best results.