When tackling programming assignments, especially those involving low-level programming languages like x86 assembly, it’s essential to break down the problem into manageable steps. x86 assembly language, known for its close-to-hardware nature, requires a meticulous approach to problem-solving. This guide offers a comprehensive approach to solving such assignments, using a classic example: determining Armstrong numbers. Armstrong numbers, also known as narcissistic numbers, are used to test understanding of number manipulation and algorithmic logic. These numbers are particularly useful in assignments because they involve calculating powers, summing results, and validating outputs—all of which require a clear understanding of arithmetic operations and program flow control.
In this guide, we will explore how to write an x86 assembly program that identifies Armstrong numbers. We will start by understanding the problem and its requirements, then move on to detailed implementation steps, including input handling, error checking, main logic, and output results. By breaking down the problem into these key components, you will learn how to manage user input, perform necessary calculations, and ensure your program behaves correctly in various scenarios. This structured approach not only helps you solve your assembly language assignment but also builds a solid foundation for addressing similar challenges in assembly language programming.
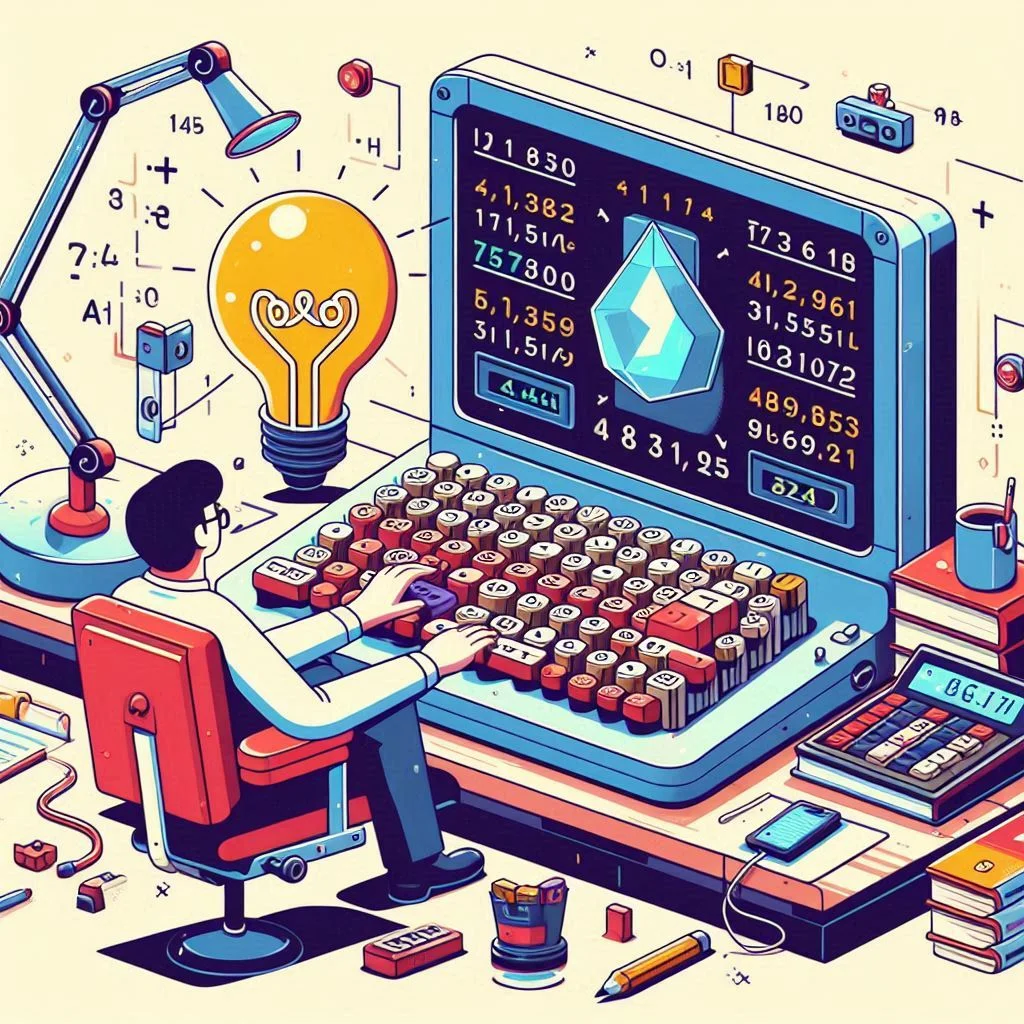
Understanding the Armstrong Number Problem
An Armstrong number, also known as a narcissistic number, is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, the number 153 is an Armstrong number because:
13+53+33=1531^3 + 5^3 + 3^3 = 15313+53+33=153
To solve this problem, you need to:
- Accept Input: Get a positive integer from the user.
- Error Handling: Check for invalid or negative inputs.
- Processing: Determine if the number is an Armstrong number.
- Output: Display results and handle user prompts.
Let’s dive into how to approach these tasks systematically.
1. Breaking down the Problem
a. Input Handling
The first step is to prompt the user for input. In x86 assembly, this involves using system calls to read from standard input. You need to handle different types of inputs:
- Valid Input: A positive integer.
- Invalid Input: Non-integer values or characters.
- Negative Input: Any negative number.
b. Error Checking
Before processing the input, you must validate it. This involves checking whether the input is a valid integer and if it is positive. If the input fails validation, you should display an appropriate error message and prompt the user to try again.
c. Main Logic
To determine if a number is an Armstrong number, follow these steps:
- Calculate the Number of Digits: Count the number of digits (m) in the number.
- Compute Powers: For each digit, compute its m-th power.
- Sum Powers: Add these powers together.
- Compare: Check if the sum equals the original number.
d. Output
Finally, you need to display the results:
- The m-th power of each digit.
- The sum of these powers.
- Whether the number is an Armstrong number or not.
- A prompt asking if the user wants to continue.
Implementing the Solution in x86 Assembly
Here’s a step-by-step breakdown of how to write the x86 assembly program for the Armstrong number problem.
a. Setting Up
Start by setting up your data section and buffer for user input. Here’s a basic setup:
section .data
prompt db "Input Number: ", 0
error_msg db "Error: Invalid input", 0
armstrong_msg db "Armstrong Number: ", 0
continue_msg db "Do you want to continue (Y/N)? ", 0
newline db 0xA, 0
b. Input Handling and Error Checking
Use system calls to read user input and handle different cases:
section .bss
number resb 10 ; Buffer for user input
input_length resb 1 ; Variable to store input length
section .text
global _start
_start:
; Display prompt
mov eax, 4 ; sys_write
mov ebx, 1 ; File descriptor (stdout)
mov ecx, prompt ; Message to write
mov edx, 13 ; Message length
int 0x80 ; Call kernel
; Read user input
mov eax, 3 ; sys_read
mov ebx, 0 ; File descriptor (stdin)
mov ecx, number ; Buffer to store input
mov edx, 10 ; Number of bytes to read
int 0x80 ; Call kernel
c. Convert Input and Validate
You need to convert the ASCII input to an integer and check for validity:
; Convert ASCII to integer
mov esi, number ; Pointer to input buffer
xor eax, eax ; Clear EAX (will hold the integer)
convert_loop:
movzx ebx, byte [esi] ; Load byte from buffer
sub ebx, '0' ; Convert ASCII to integer
imul eax, eax, 10 ; Multiply current result by 10
add eax, ebx ; Add new digit
inc esi ; Move to the next character
cmp byte [esi], 0 ; Check if end of string
jne convert_loop ; Continue if not end of string
; Error checking
cmp eax, 0 ; Check if number is non-positive
jl invalid_input
d. Main Logic for Armstrong Number
Calculate the number of digits, compute powers, and sum them:
; Count digits
mov ecx, eax ; Store number in ECX
xor edx, edx ; Clear EDX (will be the digit count)
count_digits:
xor eax, eax ; Clear EAX for division
div dword [ten] ; Divide ECX by 10
inc edx ; Increment digit count
test eax, eax ; Check if quotient is zero
jnz count_digits ; Continue if not zero
; Compute m-th power of each digit
mov eax, ecx ; Restore number in EAX
mov esi, number ; Pointer to input buffer
xor ebx, ebx ; Clear EBX (will hold sum of powers)
compute_powers:
movzx edx, byte [esi] ; Load digit
sub edx, '0' ; Convert ASCII to integer
; Calculate power
; (Use a loop or a power function)
; Add to sum
inc esi
cmp byte [esi], 0
jne compute_powers
; Compare sum with original number
cmp ebx, eax
je armstrong_yes
armstrong_no:
; Output result
; (Print the results)
jmp continue_prompt
armstrong_yes:
; Output result
; (Print the results)
e. Output Results and Continue Prompt
Use system calls to print the results and handle user continuation:
continue_prompt:
; Display continue prompt
mov eax, 4 ; sys_write
mov ebx, 1 ; File descriptor (stdout)
mov ecx, continue_msg ; Message to write
mov edx, 30 ; Message length
int 0x80 ; Call kernel
; Read user response
mov eax, 3 ; sys_read
mov ebx, 0 ; File descriptor (stdin)
mov ecx, number ; Buffer to store response
mov edx, 1 ; Number of bytes to read
int 0x80 ; Call kernel
; Check response
cmp byte [number], 'Y'
je _start ; Restart if Yes
cmp byte [number], 'N'
je exit_program ; Exit if No
invalid_input:
; Output error message
; (Print the error message and prompt again)
jmp _start
exit_program:
; Exit the program
mov eax, 1 ; sys_exit
xor ebx, ebx ; Status code 0
int 0x80 ; Call kernel
Testing and Debugging
Testing is crucial to ensure that your program works correctly. Here’s how to approach testing and debugging:
a. Test with Various Inputs
- Valid Armstrong Numbers: Test numbers like 153 and 370.
- Invalid Inputs: Test with non-integer values and negative numbers.
- Edge Cases: Test with single-digit numbers and very large numbers.
b. Debugging Tools
Use debugging tools such as gdb to step through your code, inspect registers, and ensure that each part of the program works as expected.
Review and Refine
After testing, review your code to ensure it meets all requirements:
- Error Handling: Check that all errors are handled properly.
- Output Accuracy: Verify that outputs match expected results.
- Code Efficiency: Refactor code for efficiency and readability if needed.
Conclusion
Solving programming assignments, especially in low-level languages like x86 assembly, involves a methodical approach to problem-solving. By understanding the problem, planning your approach, implementing the solution, and thoroughly testing and debugging, you can effectively tackle assignments like the Armstrong number problem. This approach not only helps in solving specific problems but also builds foundational skills for tackling similar challenges in the future.