Process scheduling stands as a cornerstone in the domain of operating systems, where its primary function lies in orchestrating the efficient allocation of computing resources and optimizing task execution. This critical role underscores its significance not only in enhancing system performance but also in ensuring seamless operations across diverse computing environments. Understanding and implementing various process scheduling algorithms are pivotal steps toward mastering these foundational principles.
At the heart of operating systems, process scheduling algorithms dictate how processes are selected for execution by the CPU. These algorithms aim to maximize system throughput, minimize response times, and maintain equitable resource allocation among competing tasks. Through simulation and analysis of different scheduling strategies, students and practitioners alike gain insights into the intricate dynamics that govern system performance under varying conditions.
This comprehensive guide serves as a roadmap for students navigating the complexities of process scheduling assignments. It provides a structured approach to implementing and comparing popular algorithms such as First-In-First-Out (FIFO), Shortest Job First (SJF), Shortest Remaining Time (SRT), Pre-emptive Multi-level Priority Scheduling with Round-Robin (RR), and Multi-level Feedback Queue (MLFQ). These algorithms are fundamental not only in theoretical studies but also in practical applications where real-time performance and efficiency are paramount.
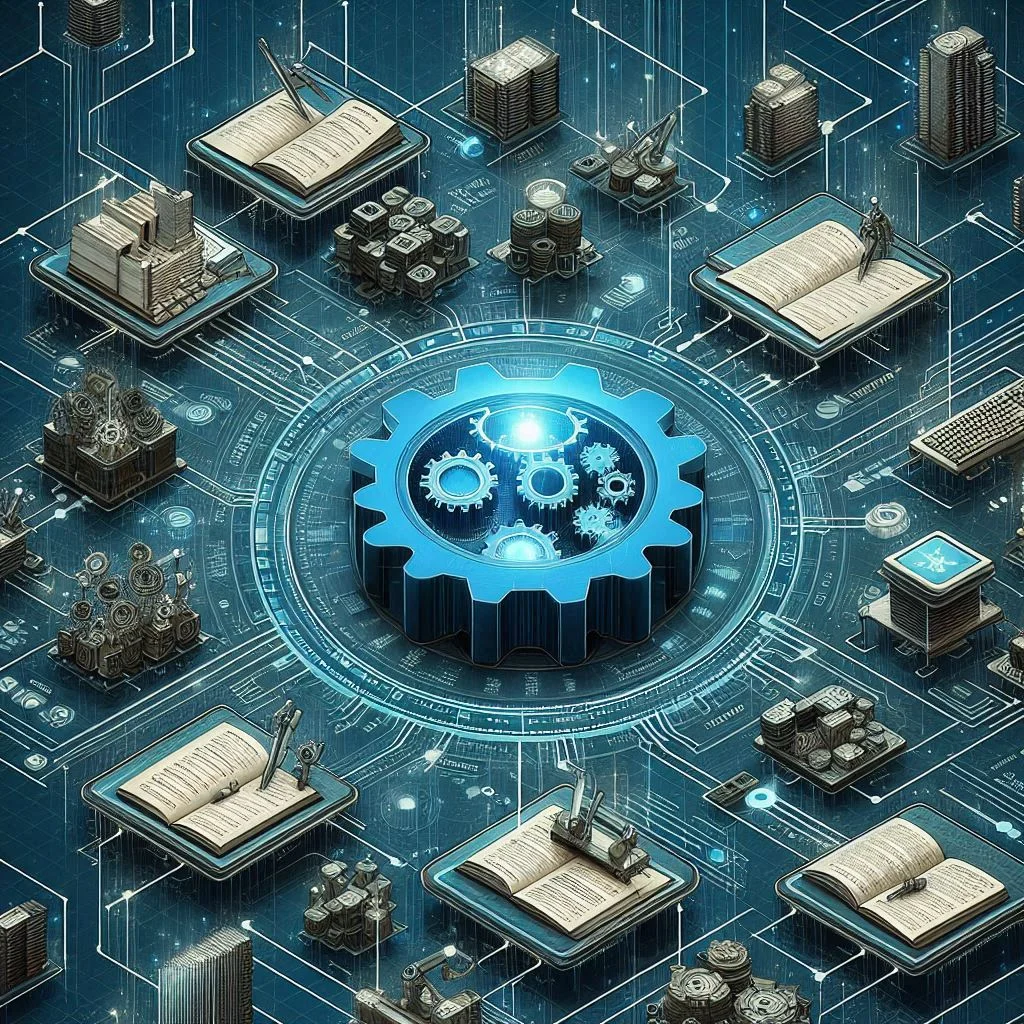
Academic assignments often simulate scenarios that challenge students to apply these algorithms effectively. From understanding arrival times and CPU burst patterns to managing process priorities and quantum slices, each algorithm offers unique strategies for optimizing system responsiveness and resource utilization. By delving into their implementation intricacies and comparative analyses, students gain proficiency in selecting the most appropriate scheduling strategy based on specific system requirements and operational constraints.
Ultimately, mastery of process scheduling algorithms empowers students to contribute to the design and improvement of operating systems, ensuring they meet the demanding performance expectations of modern computing environments. This guide aims to demystify these algorithms, providing clarity and practical insights that equip learners with essential skills for tackling both academic challenges and real-world engineering scenarios.
For those seeking help with programming assignments or needing assistance to solve their algorithm assignment using C, this guide will provide the essential knowledge and practical skills to excel.
Understanding the Assignment Requirements
Imagine a simulation where you're tasked with mimicking the execution of multiple processes under different scheduling algorithms. Here’s a breakdown of the simulated environment and key parameters:
- Processes: A set of n processes with distinct characteristics such as arrival time (Ai), total CPU time (Ti), remaining CPU time (Ri), turnaround time (TTi), and priority level (Li for some algorithms).
- Initialization: Processes arrive at random times Ai within a specified interval [0, k]. CPU times Ti follow a normal distribution with mean d and standard deviation v.
- Simulation Parameters: Parameters include the number of processes (n), arrival interval (k), mean CPU time (d), standard deviation of CPU time (v), and time quantum (q) for some scheduling algorithms.
Implementing the Simulation
To begin, let's delve into the step-by-step implementation of the simulation:
1. Initialization
Start by initializing the process table and setting initial values for each process:
import random
# Function to initialize processes
def initialize_processes(n, k, d, v):
processes = []
for i in range(n):
Ai = random.randint(0, k) # Random arrival time
Ti = max(1, int(random.normalvariate(d, v))) # Random CPU time
Ri = Ti # Remaining CPU time initially equals total CPU time
TTi = 0 # Turnaround time, initialized to 0
Li = random.randint(1, 10) # Priority level (for some algorithms)
active = 1 if Ai == 0 else 0 # Active flag, starts with arrival
processes.append({'Ai': Ai, 'Ti': Ti, 'Ri': Ri, 'TTi': TTi, 'Li': Li, 'active': active})
return processes
# Example initialization
n = 100 # Number of processes
k = 1000 # Arrival interval
d = 50 # Mean CPU time
v = 10 # Standard deviation of CPU time
processes = initialize_processes(n, k, d, v)
2. Simulation Steps
Proceed with executing the simulation based on the specified scheduling algorithms:
def simulate_scheduling(processes, q):
t = 0 # Initialize current time
while any(p['Ri'] > 0 for p in processes):
while not any(p['active'] == 1 for p in processes):
t += 1 # Increment time if no process is ready
# Implement scheduling algorithm (FIFO, SJF, SRT, Preemptive Multi-level Priority, MLF)
# Update Ri, active status, TTi, and compute average turnaround time (ATT)
for p in processes:
if p['active'] == 1:
p['Ri'] -= 1 # Decrement remaining CPU time
if p['Ri'] == 0:
p['active'] = 0 # Process terminates
p['TTi'] = t - p['Ai'] # Calculate turnaround time
# Additional logic for scheduling algorithms goes here
return processes
# Example simulation call
q = 5 # Time quantum for round-robin scheduling
simulated_processes = simulate_scheduling(processes, q)
3. Plotting Performance
After simulating each scheduling algorithm under different scenarios (e.g., (n, k) pairs), plot the performance metrics:
import matplotlib.pyplot as plt
def plot_performance(processes, algorithm_name):
# Calculate d/ATT ratios
d_vals = []
d_att_ratios = []
for d in range(int(k / n), 25 * int(k / n) + 1):
total_att = 0
for p in processes:
if p['Ri'] == 0:
total_att += p['TTi']
ATT = total_att / len(processes)
d_vals.append(d)
d_att_ratios.append(d / ATT)
# Plotting
plt.plot(d_vals, d_att_ratios, label=algorithm_name)
plt.xlabel('Value of d')
plt.ylabel('d/ATT')
plt.title('Comparison of Scheduling Algorithms Performance')
plt.grid(True)
plt.legend()
# Example plotting for FIFO scheduling
plot_performance(processes, 'FIFO')
# Display the plot
plt.show()
Comparing Algorithms and Interpreting Results
Upon generating plots of d/ATT over d for each scheduling algorithm, analyze the results:
- Performance Variations: Evaluate how FIFO, SJF, SRT, Preemptive Multi-level Priority Scheduling with RR, and MLF algorithms perform under different workload intensities, varying d values relative to k/n.
- Impact on CPU Utilization: Analyze how each scheduling policy affects process turnaround times and overall CPU efficiency, considering varying levels of process competition and resource contention.
- Algorithm Efficiency: Compare the effectiveness and performance of FIFO, SJF, SRT, Preemptive Multi-level Priority Scheduling with RR, and MLF algorithms based on plotted data, highlighting differences in system responsiveness and throughput.
Conclusion
In conclusion, the implementation and comparison of process scheduling algorithms through simulation provide invaluable insights into system performance optimization. By systematically experimenting with different parameters such as arrival times, CPU burst patterns, and scheduling policies like FIFO, SJF, SRT, Preemptive Multi-level Priority Scheduling with RR, and MLFQ, you can deepen your understanding of how these decisions impact overall system efficiency. This empirical approach not only enhances your theoretical knowledge but also hones your practical skills in tackling real-world challenges.
Further exploration into advanced scheduling techniques, such as dynamic priority adjustments, real-time scheduling constraints, and adaptive scheduling algorithms, opens avenues for enhanced system responsiveness and resource utilization. Understanding these nuances is crucial for designing robust operating systems capable of meeting the demands of modern computing environments.
Continued study and application of process scheduling algorithms empower you to contribute meaningfully to the field of operating systems design. By staying abreast of emerging trends and innovations, you'll be well-prepared to address complex computing challenges and optimize system performance effectively. Embrace the opportunity to delve deeper into these concepts, refine your analytical capabilities, and make significant strides in advancing operating system efficiency and reliability.