Efficient data retrieval stands as a cornerstone for achieving optimal performance in computer systems. At the heart of this endeavor lie disk scheduling algorithms, which play a pivotal role in minimizing disk head movement and thereby enhancing overall system efficiency. This resource is meticulously crafted to equip students with comprehensive knowledge and effective strategies necessary to excel in programming assignment. By delving into the intricate workings of various disk scheduling algorithms and mastering their implementation nuances, students can effectively optimize data access, reduce access times, and bolster system throughput.
Understanding the fundamental principles behind disk scheduling algorithms is crucial. Algorithms like First-Come, First-Served (FCFS), Shortest Seek Time First (SSTF), SCAN, C-SCAN, LOOK, and C-LOOK each offer unique methodologies for managing the positioning of the disk arm and servicing data requests. FCFS, for instance, processes requests in the order they are received, while SSTF prioritizes the closest request to the current disk arm position to minimize seek time. SCAN and C-SCAN move the disk arm across the surface in one direction and then reverse, optimizing access to requests across the disk. LOOK and C-LOOK refine this approach by limiting movement to areas where requests are pending, reducing unnecessary travel.
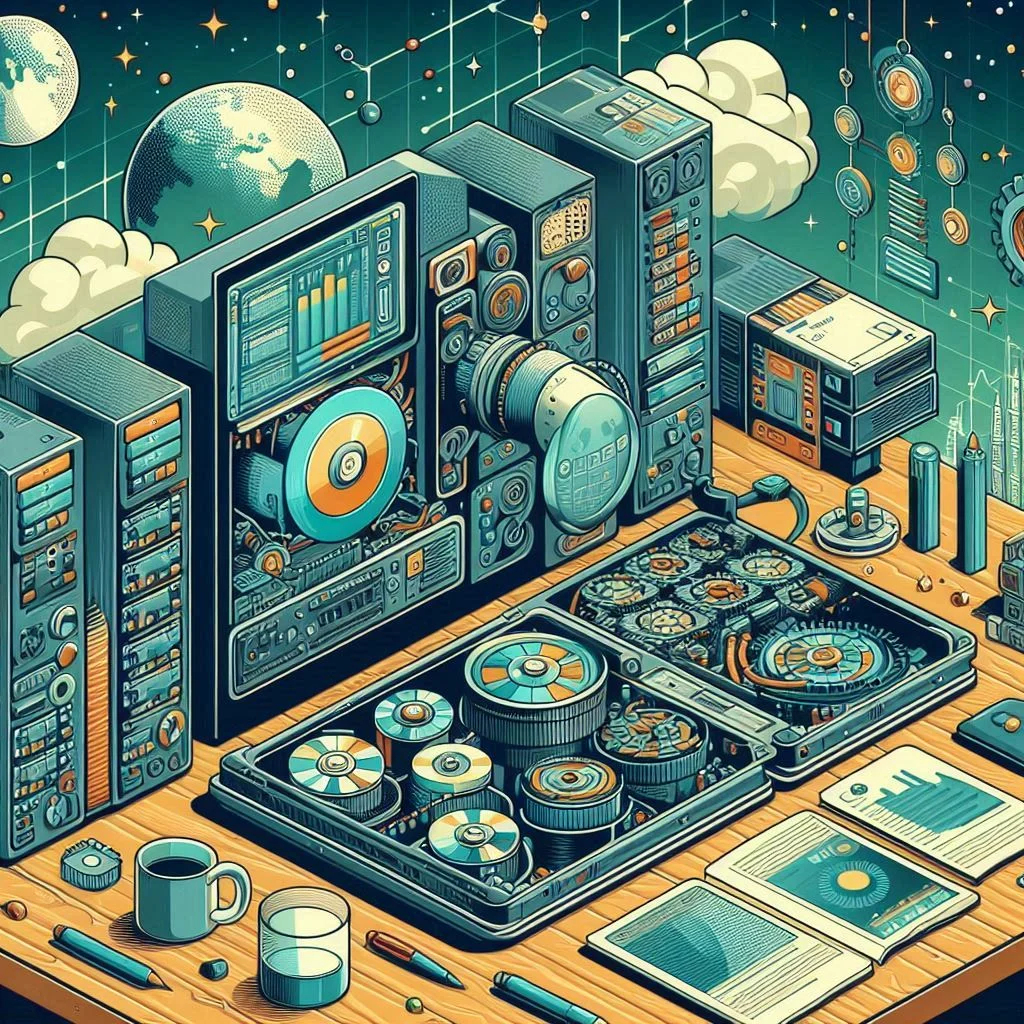
Successful implementation of these algorithms hinges on thorough testing and validation against both textbook examples and randomized data sequences. By validating outputs against expected results, students can ensure the accuracy and reliability of their implementations. This validation process not only confirms the correct functioning of algorithms under ideal conditions but also prepares students to handle real-world scenarios where data access patterns may vary unpredictably.
Understanding Disk Scheduling
Understanding disk scheduling entails efficiently managing the disk arm's movement to retrieve data blocks. The main objective is minimizing seek time, crucial for positioning the disk arm swiftly over the desired track to improve overall throughput. Various algorithms tackle this challenge, each offering unique strategies to optimize disk access. Whether it's First-Come, First-Served (FCFS), Shortest Seek Time First (SSTF), SCAN, C-SCAN, LOOK, or C-LOOK, each algorithm plays a critical role in determining how effectively data can be accessed from the disk. By implementing these algorithms, systems can significantly enhance their performance by reducing unnecessary disk head movements and maximizing data retrieval efficiency.
Problem Description
In a typical disk scheduling simulation project, students are tasked with implementing and comparing various disk scheduling algorithms aimed at optimizing data retrieval efficiency:
- First-Come, First-Served (FCFS): This algorithm processes requests in the order they arrive, straightforward but not necessarily optimal for minimizing disk head movement.
- Shortest Seek Time First (SSTF): Prioritizes servicing the request closest to the current position of the disk arm, minimizing seek time and improving overall throughput.
- SCAN and C-SCAN: These algorithms move the disk arm across the disk surface in one direction, servicing requests encountered along the way, and then reverse direction, reducing wasted movement.
- LOOK and C-LOOK: Similar to SCAN but optimized to only service requests in the direction of travel, thereby further reducing unnecessary disk arm movement and enhancing efficiency in data access.
Theory of Operation and Solution Design
UML Diagram Overview:
In the context of disk scheduling simulations, the UML diagram serves as a blueprint for understanding and implementing various components:
- DiskSchedulingSimulation: This pivotal component initializes the simulation, interacts with users to gather input parameters such as disk characteristics and request sequences, and orchestrates the execution of different scheduling algorithms.
- ScheduleAlgorithm: Acting as an interface, ScheduleAlgorithm defines essential methods that all scheduling algorithms must implement. These methods include calculating total head movements, generating output sequences, and providing a structured approach to data access optimization.
- ScheduleAlgorithmBase: Serving as an abstract class, ScheduleAlgorithmBase provides foundational methods and data structures that streamline the implementation of scheduling algorithms. By encapsulating common functionalities and data management practices, it ensures consistency and efficiency across different algorithm implementations.
- Algorithm Classes: These concrete classes, such as FCFSAlgorithm and SSTFAlgorithm, extend ScheduleAlgorithmBase to specialize in specific disk scheduling strategies. Each class tailors its implementation to optimize disk access based on unique algorithms like First-Come, First-Served (FCFS) or Shortest Seek Time First (SSTF), contributing to the overall effectiveness of the simulation in improving system performance and minimizing overhead.
Steps to Approach Your Assignment
Step 0: Familiarize Yourself Before diving into implementation:
- Understand disk scheduling principles covered in lectures and textbooks.
- Review provided source files (e.g., DiskSchedulingSimulation.java, ScheduleAlgorithm.java) to grasp existing functionality.
Step 1: Implementing Algorithms Begin with:
- FCFSAlgorithm: As a foundational template, FCFSAlgorithm provides insights into structuring and implementing scheduling algorithms. It processes requests strictly in their arrival order, offering simplicity but not necessarily the most efficient utilization of disk access time.
- SSTFAlgorithm: This algorithm optimizes disk access by prioritizing requests closest to the current position of the disk arm. By minimizing seek time, SSTFAlgorithm enhances overall throughput and responsiveness in data retrieval.
- SCANAlgorithm: Designed to manage disk arm movement efficiently, SCANAlgorithm considers both left-to-right and right-to-left scanning directions. This approach ensures that requests are serviced in a sequential manner, reducing unnecessary head movements and optimizing data access performance across the disk surface.
Step 2: Testing and Validation
- Validate each algorithm against provided textbook examples and random data sequences.
- Ensure outputs include total head movements and the sequence in which requests are serviced, matching expected results.
Step 3: Implementing Additional Algorithms
Implementing Additional Algorithms
- CSCANAlgorithm: This algorithm enhances traditional SCAN by ensuring the disk arm returns to the opposite end of the disk after completing a scan. By focusing on servicing requests in a continuous circular manner, CSCANAlgorithm minimizes head movements and improves efficiency, particularly in systems with high request rates or large data sets.
- LOOKAlgorithm: Similar to SCAN, LOOKAlgorithm services requests only in the direction of travel, omitting unnecessary head movements. This targeted approach reduces latency and optimizes disk access time, crucial for applications requiring rapid data retrieval and response.
- CLOOKAlgorithm: Building on CLOOK, this algorithm prioritizes efficiency by focusing on contiguous blocks of data requests. By handling requests in a circular manner, CLOOKAlgorithm minimizes seek time and maximizes disk utilization, making it ideal for environments with fluctuating workload demands or variable request patterns.
- Directional Dependencies: Consider the impact of directional dependencies on algorithm performance. Algorithms like SCAN and CLOOK may behave differently based on initial arm position or scanning direction. Adjust algorithm behaviors to handle edge cases effectively, ensuring consistent and reliable performance across various disk access scenarios.
Output and Reporting
To effectively demonstrate the success of your disk scheduling simulation project, it's crucial to focus on the following key aspects:
- Comprehensive Reports: Your simulation should generate detailed reports that not only list algorithm names but also include critical metrics such as total head movements and the exact sequence of disk access for each algorithm. These reports provide a clear overview of how each scheduling strategy performs under different scenarios.
- Validation against Expected Results: It's essential to validate simulation outputs against expected results meticulously. This verification process ensures the accuracy and reliability of your implementations, confirming that each algorithm behaves as intended and meets the specified performance criteria.
- Analysis and Interpretation: Beyond generating reports, consider providing an analysis of the results. Discuss any observed trends or differences between algorithms, highlight strengths and weaknesses, and offer insights into how each approach impacts overall system performance and efficiency.
- Visual Representation: Utilize graphs, charts, or tables where appropriate to visually represent key findings from your simulation. Visual aids can enhance understanding and make complex data more accessible to your audience.
- Documentation: Document your approach to generating reports and validating results in your project documentation. This ensures transparency and helps future developers or researchers understand your methodology and conclusions.
Submission Requirements
When preparing your disk scheduling simulation project for submission, ensure the following steps are completed:
- Compile and Submit Java Source Files: Gather all Java source files, ensuring they are properly compiled and organized according to assignment guidelines. This includes verifying that files are in the correct format and adhere to naming conventions.
- Clarity and Organization: Emphasize clarity and organization in your code structure. Use meaningful variable names, adhere to consistent coding style, and employ comments to explain complex logic and algorithm implementations.
- Comprehensive Documentation: Include detailed documentation outlining each algorithm's approach, the rationale behind its implementation choices, and comprehensive testing outcomes. This documentation should provide insights into how each algorithm optimizes disk access and how it performs under different scenarios or test cases.
- Adherence to Assignment Guidelines: Double-check that your submission meets all specified requirements, including file formats, submission deadlines, and any additional instructions provided by your instructor. Clear adherence to guidelines ensures your project is evaluated accurately and fairly.
Conclusion
Achieving proficiency in disk scheduling simulations demands a solid grasp of both theory and practical implementation. By rigorously exploring each algorithm and following outlined steps, students can confidently approach and excel in similar assignments. Disk scheduling algorithms play a critical role in optimizing system performance, making skilled implementation essential for future software engineers and system designers.
Practical implementation through thorough testing and validation across various scenarios reinforces understanding and ensures the reliability of algorithmic solutions. This hands-on approach fosters critical thinking and problem-solving skills crucial for navigating complex computing environments.
Additionally, documenting algorithmic choices and outcomes enhances clarity and transparency in project development, providing a valuable reference for future optimizations.
Ultimately, mastering disk scheduling simulations equips students with the skills to optimize data retrieval, minimize latency, and enhance overall system efficiency. This preparation prepares them to innovate and contribute effectively to advancing system design and performance in evolving computing landscapes.