Java programming assignments often require students to develop graphical user interface (GUI) applications using Swing. In this guide, we'll walk through the process of designing, implementing, and testing a Java Shape Selector GUI, similar to assignments commonly encountered in programming courses. This assignment involves creating a GUI that allows users to select shapes, input parameters, and display those shapes dynamically within a Swing-based interface. Graphical user interfaces play a crucial role in modern software development, enabling intuitive interaction between users and applications. The Java Swing library provides a robust framework for creating GUI applications, offering a variety of components and layout managers to build responsive interfaces. This assignment will not only test your understanding of Java programming fundamentals but also your ability to utilize Swing components effectively. Navigating through this guide, you will gain practical insights into each stage of the development lifecycle related to Java assignment help. From conceptualizing the GUI layout and designing class hierarchies to implementing event-driven programming and conducting rigorous testing, each step is meticulously outlined to bolster your proficiency in GUI development and programming assignment. Whether you are a novice programmer seeking foundational knowledge or a seasoned developer refining your skills, mastering the Java Shape Selector GUI assignment is a rewarding endeavour that promises to sharpen your programming acumen and elevate your software engineering prowess.
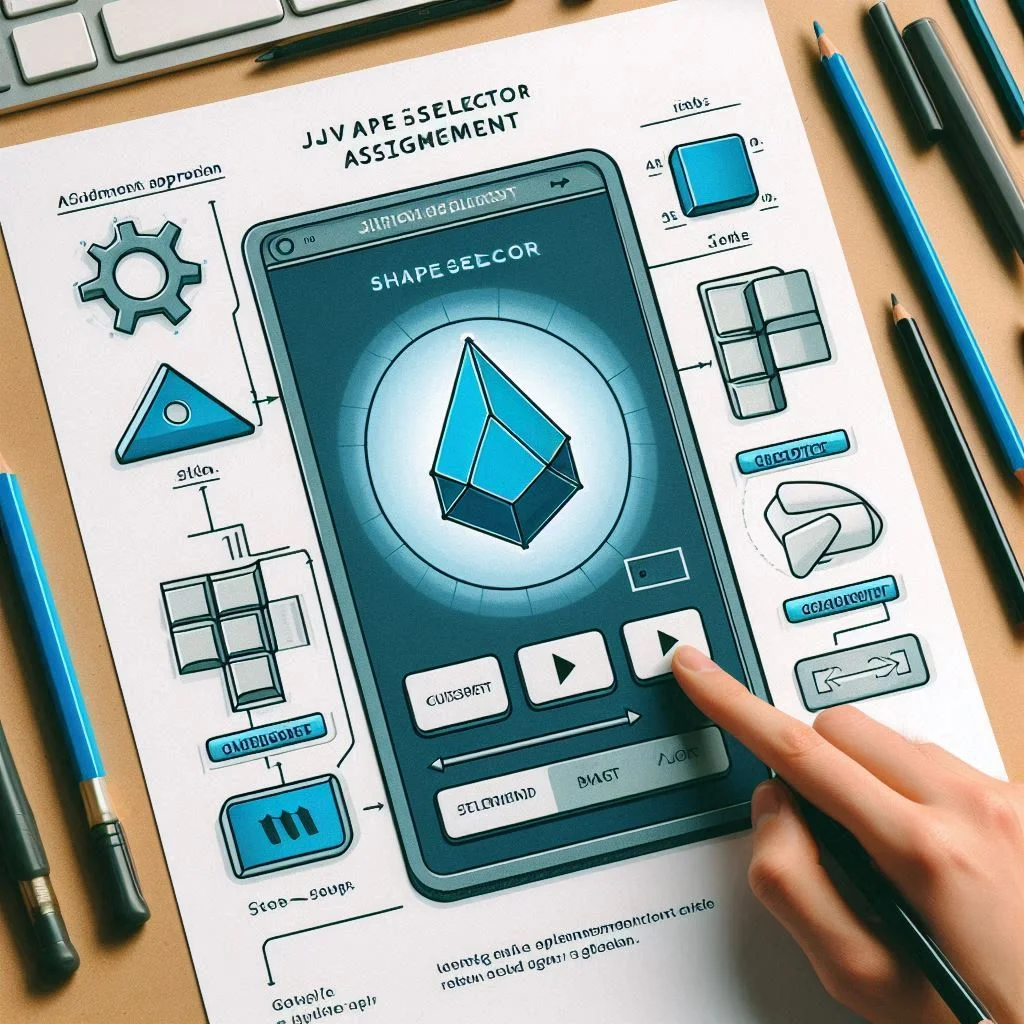
Understanding the Assignment Requirements
Before diving into implementation, it's essential to grasp the assignment requirements thoroughly. Here's an overview of what we need to accomplish:
1. Shape Selection and Parameters:
- Implement a GUI that allows users to select shapes from a predefined list (e.g., Circle, Square, and Triangle).
- Provide input fields for dimensional parameters specific to each shape (e.g., radius for Circle, side length for Square).
2. GUI Display:
- Display selected shapes dynamically within a Swing-based frame.
- Use appropriate layout managers to ensure the GUI is organized and responsive.
3. Advanced Feature for 3-D Shapes:
- For 3-D shapes (e.g., Sphere, Cube), load representative images from files and display them within the GUI.
- Ensure smooth integration of image loading and display functionalities.
Planning Your Java Classes
A well-designed class structure is fundamental to the success of any Java application. For this assignment, consider the following class hierarchy and relationships:
Inheritance and Composition:
- Design classes for each shape that reflect "is-a" and "has-a" relationships (e.g., Circle extends Shape).
- Utilize composition where applicable to encapsulate behaviors and properties.
UML Class Diagram:
- Provide a visual representation of your class hierarchy using Unified Modeling Language (UML).
- Illustrate how classes interact and inherit from each other to meet the project's requirements.
Setting Up the Swing GUI
Swing offers a rich set of components and layout managers to build flexible GUIs. Here's how you can set up your Swing-based interface effectively:
1. Layout Managers:
- Choose appropriate layout managers (e.g., Border Layout, GridLayout) to organize components within the frame.
- Ensure components resize and align correctly as the window size changes.
2. Event Handling and Listeners:
- Implement event handlers and listener interfaces to respond to user interactions (e.g., button clicks).
- Use Adapter classes or inner classes for cleaner event handling implementations.
3. Widgets and Components:
- Integrate buttons, text fields, and other widgets to facilitate shape selection and parameter input.
- Customize components to enhance usability and visual appeal.
Implementing the Shape Selection and Display Logic
With the GUI framework in place, it's time to implement the core functionality of selecting and displaying shapes:
1. Handling User Input:
- Capture user selections and parameter inputs using appropriate event listeners.
- Validate input to ensure it meets specified constraints (e.g., non-negative dimensions).
2. Dynamic Shape Display:
- Based on user selections, instantiate corresponding shape objects.
- Utilize Swing's drawing capabilities (e.g., Graphics2D) to render shapes within designated areas of the GUI.
3. Loading 3-D Shape Images:
- Implement image loading from files for 3-D shapes such as Sphere, Cube, etc.
- Display loaded images alongside shape renderings to provide a visual representation.
Testing Your Application
Testing is critical to ensure your application functions as intended across various scenarios:
1. Test Plan Creation:
- Develop a comprehensive test plan covering different user interactions and edge cases.
- Document expected outputs and compare them against actual results during testing.
2. Debugging and Error Handling:
- Identify and resolve any coding errors or logical inconsistencies.
- Handle exceptions gracefully to prevent application crashes during runtime.
3. User Experience Validation:
- Gather feedback on usability and responsiveness from test users (if possible).
- Incorporate user feedback to improve the application's design and functionality.
Documenting and Submitting Your Project
Proper documentation is essential for clarity and ease of evaluation:
1. Source Code Documentation:
- Include header comments in each Java source file detailing file name, author, date, and purpose.
- Use meaningful variable and function names and maintain consistent coding style and indentation.
2. Submission Preparation:
- Compile all Java source files into a single compressed zip archive.
- Include necessary data files, configuration files, and any external resources used in the project.
3. Developer’s Guide and User’s Guide:
- Prepare a developer’s guide outlining how to compile, execute, and test the application.
- Create a user’s guide with step-by-step instructions on setting up and using the GUI application.
4. Reflections and Lessons Learned:
- Reflect on challenges faced during the project and how they were overcome.
- Document lessons learned and insights gained from the assignment.
Conclusion
In conclusion, developing a Java Shape Selector GUI using Swing is a rewarding exercise that combines Java programming skills with GUI design principles. By following the steps outlined in this guide, you can effectively tackle similar programming assignments and hone your proficiency in Java development. Remember to prioritize clear documentation, thorough testing, and user-centric design to deliver a polished and functional application.
Implementing GUI applications in Java not only enhances your technical skills but also prepares you for real-world software development scenarios where user interaction and usability are paramount. Embrace the learning journey and enjoy the process of crafting intuitive and engaging GUIs with Java Swing!