Java Database Connectivity (JDBC) assignments are fundamental in many database and software engineering courses, providing a hands-on approach to learning how to connect Java applications to databases, execute SQL queries, and manage data effectively. These assignments typically require students to establish connections with databases, execute SQL scripts to set up schema and insert data, and implement a user interface, often through a command-line interface (CLI). Key steps include loading the appropriate JDBC driver, establishing a connection using DriverManager.getConnection, and utilizing Statement or PreparedStatement for executing SQL commands. It's essential to design a robust command-line interface that allows users to interact with the database seamlessly, performing actions such as viewing table contents, searching by specific fields like MovieID, and inserting new data from CSV files. Best practices involve thorough input validation to prevent SQL injection and other common security issues, as well as comprehensive error handling using try-catch blocks to manage exceptions gracefully and provide meaningful feedback to the user. Regular testing is crucial to ensure that each functionality works correctly and to identify and fix bugs early. Additionally, breaking down the assignment into smaller, manageable tasks can help in tackling complex requirements methodically. For extra credit or advanced learning, students can develop a graphical user interface (GUI) using frameworks like JavaFX or web applications using servlets and JSP to offer a more intuitive user experience. Documenting the code and including screenshots or logs demonstrating the program's capabilities are also vital for a clear and complete submission. By following these steps and best practices, students can effectively navigate the challenges of JDBC assignments, gaining valuable skills in database connectivity and application development. This guide will offer valuable assistance with their Java assignment, ensuring they have the support and resources needed to succeed in their JDBC projects.
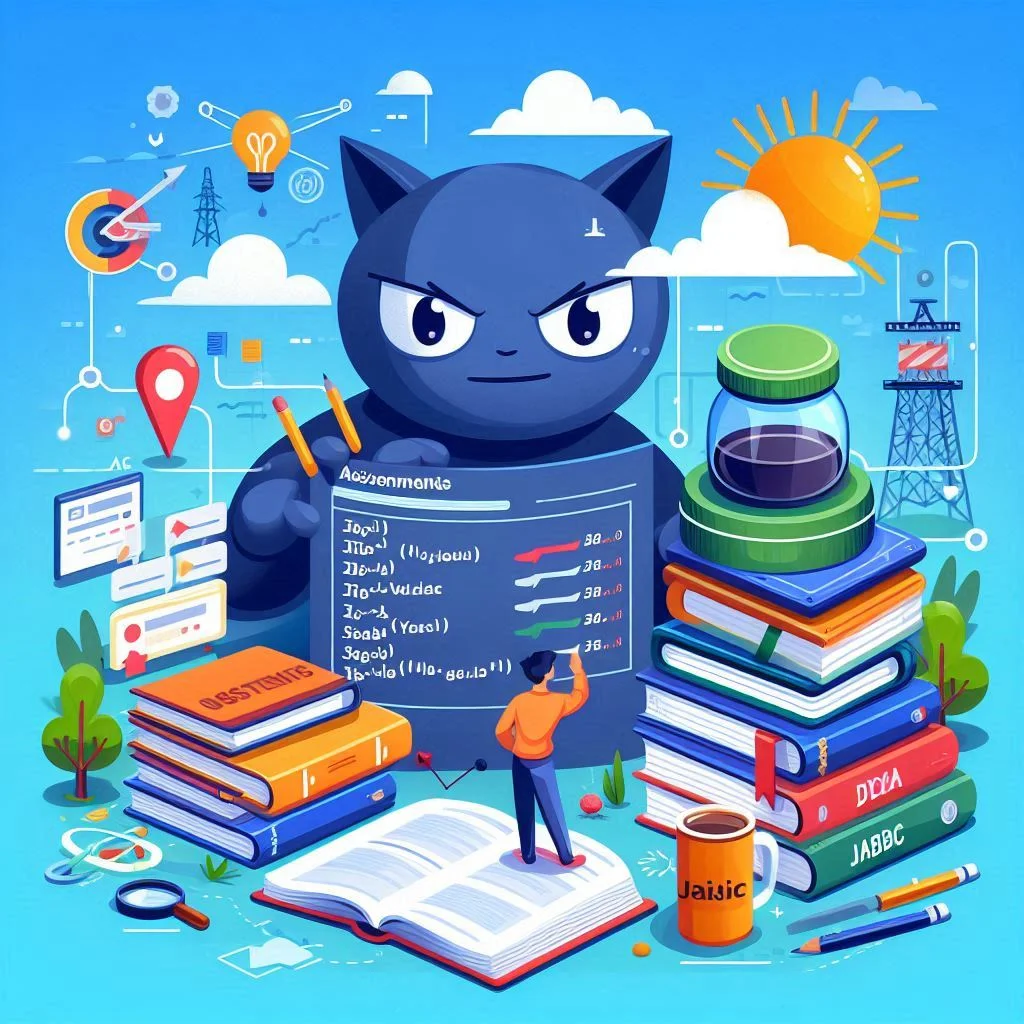
Understanding the Assignment Requirements
Before diving into the code, it's crucial to thoroughly read and understand the assignment requirements. For instance, in the provided sample assignment, the main tasks include:
- Connecting to an Oracle database: This task typically involves using JDBC (Java Database Connectivity) to establish a connection to an Oracle database instance. It requires loading the appropriate JDBC driver and providing the necessary credentials (username and password).
- Executing an SQL script to set up the database: Often, assignments require setting up database schema and initial data. This is done by executing SQL scripts provided by the instructor, which define table structures, constraints, and initial data inserts. The Statement or PreparedStatement classes in Java are used to execute these scripts against the connected database.
- Creating a command-line interface (CLI) for database operations: The CLI allows users to interact with the database through text-based commands. It typically includes a menu-driven interface where users can choose operations such as viewing table contents, searching for specific data (like movies by MovieID), inserting new data, and exiting the program.
- Handling user inputs and displaying results: Effective handling of user inputs is critical to ensure the program behaves as expected. This includes validating inputs, handling errors gracefully (such as invalid commands or data), and displaying results in a clear and organized manner. Java's Scanner class is commonly used to read user inputs from the command line.
Additionally, the assignment outlines specific functionalities your program should implement, such as viewing table contents, searching by MovieID, and inserting data from CSV files. Clear understanding of these requirements is the first step toward successfully completing your assignment.
Setting Up Your Development Environment
Ensure you have the necessary tools and libraries installed:
- Java Development Kit (JDK): Make sure you have the latest version of JDK installed. It includes the Java Runtime Environment (JRE), compilers, and tools needed to develop Java applications.
- JDBC Driver for Oracle: Download the Oracle JDBC driver (ojdbc.jar) from Oracle's official website and add it to your project's class path. This driver enables your Java application to connect to the Oracle database.
- Integrated Development Environment (IDE): Use an IDE like IntelliJ IDEA, Eclipse, or NetBeans for easier development and debugging. These IDEs offer features like code completion, syntax highlighting, and integrated debugging tools.
Establishing a Database Connection
Start by writing a Java program to connect to the Oracle database. Use the DriverManager class to load the JDBC driver and establish a connection. Here’s a basic example:
java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
public static Connection getConnection(String username, String password) {
Connection connection = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
connection = DriverManager.getConnection(
"jdbc:oracle:thin:@artemis.vsnet.gmu.edu:1521/vse18c.vsnet.gmu.edu",
username, password);
System.out.println("Connected to the database.");
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
return connection;
}
public static void main(String[] args) {
// Prompt user for username and password
// Call getConnection with the provided credentials
}
}
Executing SQL Script to Set Up Database
Once connected, execute the provided SQL script to create tables and insert initial data. Use the Statement class to run the script. Here’s an example:
java
import java.io.BufferedReader;
import java.io.FileReader;
Import java.io.IOException;
import java.sql.Connection;
import java.sql.Statement;
public class DatabaseSetup {
public static void executeSqlScript(Connection connection, String filePath) {
try (BufferedReader reader = new BufferedReader(new FileReader(filePath));
Statement statement = connection.createStatement()) {
String line;
while ((line = reader.readLine()) != null) {
statement.execute(line);
}
System.out.println("Database setup complete.");
} catch (Exception | SQLException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
// Get database connection
// Prompt user for the path to movies.sql
// Call executeSqlScript with the connection and file path
}
}
Implementing the Command-Line Interface
Create a menu-driven interface to interact with the database. Use Scanner for user input and switch-case to handle different options. Here’s an outline:
Java
import java.sql.Connection;
import java.util.Scanner;
public class MovieDatabaseApp {
public static void main(String[] args) {
// Get database connection
// Execute SQL script to set up database
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Menu:");
System.out.println("1. View table contents");
System.out.println("2. Search by MOVIEID");
System.out.println("3. Insert by MOVIEID");
System.out.println("4. Exit");
System.out.print("Choose an option: ");
int choice = scanner.nextInt();
scanner.nextLine(); // Consume newline
switch (choice) {
case 1:
// View table contents
break;
case 2:
// Search by MOVIEID
break;
case 3:
// Insert by MOVIEID
break;
case 4:
System.out.println("Exiting...");
return;
default:
System.out.println("Invalid option. Please try again.");
}
}
}
// Implement methods for each case (viewTableContents, searchByMovieID, insertByMovieID)
}
Viewing Table Contents
Write a method to retrieve and display the contents of the Movies and Ratings tables. Use Result Set to fetch data and format the output properly:
java
public static void viewTableContents(Connection connection) {
try (Statement statement = connection.createStatement()) {
System.out.println("Movies Table:");
ResultSet movies = statement.executeQuery("SELECT * FROM Movies");
while (movies.next()) {
System.out.printf("%d, %s, %s, %s, %s, %s, %d%n",
movies.getInt("MovieID"),
movies.getString("Title"),
movies.getString("Language"),
movies.getString("Production_company"),
movies.getString("Production_country"),
movies.getDate("Release_date"),
movies.getInt("Runtime"));
}
System.out.println("Ratings Table:");
ResultSet ratings = statement.executeQuery("SELECT * FROM Ratings");
while (ratings.next()) {
System.out.printf("%d, %d, %d%n",
ratings.getInt("ReviewerID"),
ratings.getInt("MovieID"),
ratings.getInt("Rating"));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
Searching by MovieID
Write a method to search for a movie by its ID and display its details along with the average rating:
java
public static void searchByMovieID(Connection connection, int movieID) {
try (Statement statement = connection.createStatement()) {
String query = "SELECT M.*, AVG(R.Rating) AS AverageRating " +
"FROM Movies M LEFT JOIN Ratings R ON M.MovieID = R.MovieID " +
"WHERE M.MovieID = " + movieID + " GROUP BY M.MovieID, M.Title, M.Language, M.Production_company, " +
"M.Production_country, M.Release_date, M.Runtime";
ResultSet result = statement.executeQuery(query);
if (result.next()) {
System.out.printf("%d, %s, %s, %s, %s, %s, %d, %.2f%n",
result.getInt("MovieID"),
result.getString("Title"),
result.getString("Language"),
result.getString("Production_company"),
result.getString("Production_country"),
result.getDate("Release_date"),
result.getInt("Runtime"),
result.getDouble("AverageRating"));
} else {
System.out.println("No results found.");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
Inserting Data from CSV Files
Write a method to read data from movies.csv and ratings.csv and insert it into the database:
java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.sql.Connection;
import java.sql.Statement;
public static void insertByMovieID(Connection connection, String moviesCsvPath, String ratingsCsvPath, int movieID) {
try (BufferedReader moviesReader = new BufferedReader(new FileReader(moviesCsvPath));
BufferedReader ratingsReader = new BufferedReader(new FileReader(ratingsCsvPath));
Statement statement = connection.createStatement()) {
// Insert movie data
String movieLine;
while ((movieLine = moviesReader.readLine()) != null) {
String[] movieData = movieLine.split(",");
if (Integer.parseInt(movieData[0]) == movieID) {
String insertMovieQuery = String.format(
"INSERT INTO Movies VALUES (%d, '%s', '%s', '%s', '%s', TO_DATE('%s', 'YYYY-MM-DD'), %d)",
Integer.parseInt(movieData[0]), movieData[1], movieData[2], movieData[3], movieData[4], movieData[5], Integer.parseInt(movieData[6]));
statement.execute(insertMovieQuery);
break;
}
}
// Insert ratings data
String ratingLine;
int ratingCount = 0;
while ((ratingLine = ratingsReader.readLine()) != null) {
String[] ratingData = ratingLine.split(",");
if (Integer.parseInt(ratingData[1]) == movieID) {
String insertRatingQuery = String.format(
"INSERT INTO Ratings VALUES (%d, %d, %d)",
Integer.parseInt(ratingData[0]), Integer.parseInt(ratingData[1]), Integer.parseInt(ratingData[2]));
statement.execute(insertRatingQuery);
ratingCount++;
}
}
System.out.printf("Inserted MovieID: %d with %d ratings.%n", movieID, ratingCount);
} catch (IOException | SQLException e) {
e.printStackTrace();
}
}
Handling Errors Gracefully
Ensuring your program handles invalid inputs and errors gracefully is crucial for creating a robust and user-friendly application. Use try-catch blocks extensively to manage exceptions that may arise during runtime, such as database connection failures, SQL errors, and file handling issues. When an error occurs, catch the exception and provide meaningful error messages to inform the user about what went wrong and how they can correct it. For example, if the user provides an invalid MovieID, catch the NumberFormatException and prompt the user to enter a valid integer. Similarly, if the CSV files contain incorrect data or formatting issues, catch the IOException and notify the user with a clear message indicating the problem. Ensuring your program does not crash and instead provides informative feedback helps users understand and rectify their mistakes, leading to a better overall experience. By anticipating potential errors and handling them gracefully, you can significantly enhance the reliability and usability of your application.
Testing Your Program
Thoroughly test your program to ensure all functionalities work as expected. Create different test cases, such as:
- Viewing contents of both tables when they are empty and when they contain data.
- Searching for existing and non-existing MovieID.
- Inserting data with valid and invalid MovieID.
- Handling invalid menu options gracefully.
Extra Credit: GUI Interface
For extra credit, consider creating a graphical user interface (GUI) or a web application. This enhancement involves using frameworks like JavaFX for desktop applications or servlets/JSP for web applications. A well-designed GUI can greatly improve the user experience by providing a more intuitive and visually appealing way to interact with your program. Ensure that the GUI replicates all the functionalities of the CLI, such as viewing table contents, searching by Movie ID, and inserting data from CSV files. Focus on making the interface user-friendly, with clear navigation, responsive design, and informative error messages to guide the user through each process.
Final Deliverables
When submitting your assignment, ensure you include all relevant source files, such as the main Java classes and any additional utility classes that support your program's functionality. Organize these files clearly within a well-structured directory to facilitate easy navigation and review.
Additionally, provide comprehensive documentation that demonstrates your program running each menu option. This can be in the form of screenshots or logs that capture the output and behavior of your application during execution. Each screenshot or log should clearly show the execution of key functionalities like viewing table contents, searching by Movie ID, and inserting data from CSV files.
Tips for Success
Success in completing JDBC assignments requires not only technical proficiency but also effective project management and problem-solving skills. Here are additional tips to enhance your approach:
- Read the Documentation: JDBC and SQL documentation provide comprehensive resources on methods, classes, and best practices. Regularly refer to these documents to deepen your understanding of JDBC functionalities and SQL syntax. Understanding the underlying mechanisms will empower you to write more efficient and effective code.
- Break down the Problem: Large assignments can seem daunting at first glance. Break down the assignment into smaller, manageable tasks. Start with setting up the database connection, and then proceed to executing SQL scripts, implementing the CLI interface, and handling user inputs. Tackling each task incrementally allows you to focus on one aspect at a time, reducing complexity and ensuring thoroughness in your implementation.
- Test frequently and rigorously: Testing is crucial in software development to identify and fix issues early. Write comprehensive test cases that cover different scenarios, including typical use cases and edge cases. Test for valid inputs as well as invalid inputs to ensure robust error handling. Automated testing frameworks can streamline this process, but manual testing is also valuable for exploring unexpected behaviour.
- Seek Help When Needed: Don't hesitate to seek assistance when facing challenges. Collaborate with classmates, participate in online forums, or consult your instructor for guidance. Explaining your problem to others often leads to new perspectives and solutions. Additionally, platforms like Stack Overflow and developer communities provide valuable insights and solutions to common problems encountered in JDBC assignments.
Conclusion
JDBC assignments offer valuable insights into database connectivity and data manipulation using Java. They provide hands-on experience in establishing connections, executing SQL queries, and managing database interactions effectively. By following the steps outlined in this guide, you can approach JDBC assignments confidently and develop applications that seamlessly interact with databases.
Start by planning your assignment thoroughly. Break down tasks into manageable steps, from setting up database connections to implementing user interfaces or CLI functionalities. This structured approach not only simplifies complex tasks but also ensures that each component of your application functions as intended.
When writing code, prioritize clarity and modularity. Use meaningful variable names, adhere to coding standards, and consider the readability of your codebase. This not only helps you understand your code better but also facilitates collaboration and maintenance.
Testing is crucial to identify and fix errors early in the development process. Write comprehensive test cases to validate different scenarios, including edge cases and error conditions. Regular testing ensures that your application handles unexpected inputs gracefully and maintains robust performance.
Throughout your assignment, leverage resources such as JDBC documentation, online tutorials, and peer collaboration to deepen your understanding and troubleshoot challenges effectively. Learning from both successes and setbacks enhances your skills in Java programming and database management.