Assembly language assignments can be daunting due to their low-level complexity and the precision required for successful implementation. However, adopting a structured approach can significantly ease this process. By breaking down the task into manageable steps, students can effectively tackle various assignments, including coding the Insertion Sort algorithm and implementing the Linear Search algorithm. Understanding the fundamental concepts behind these algorithms is crucial, as it allows you to translate their logic into assembly language. For Insertion Sort, the key is to iteratively insert each element into its proper position within a sorted subset of the array, while Linear Search involves sequentially checking each element to find a specified value. Practicing these algorithms not only enhances your programming skills but also deepens your understanding of how data structures operate at a fundamental level. Utilizing pseudo code to outline your approach before diving into actual coding can further simplify the process. Overall, the techniques and principles you learn while implementing Insertion Sort and Linear Search will serve as a solid foundation for solving similar assembly language assignments, fostering both confidence and competence in your programming journey. For additional support, consider seeking Programming Assignment Help or data structure assignment help to strengthen your skills further.
Understanding the Assignment
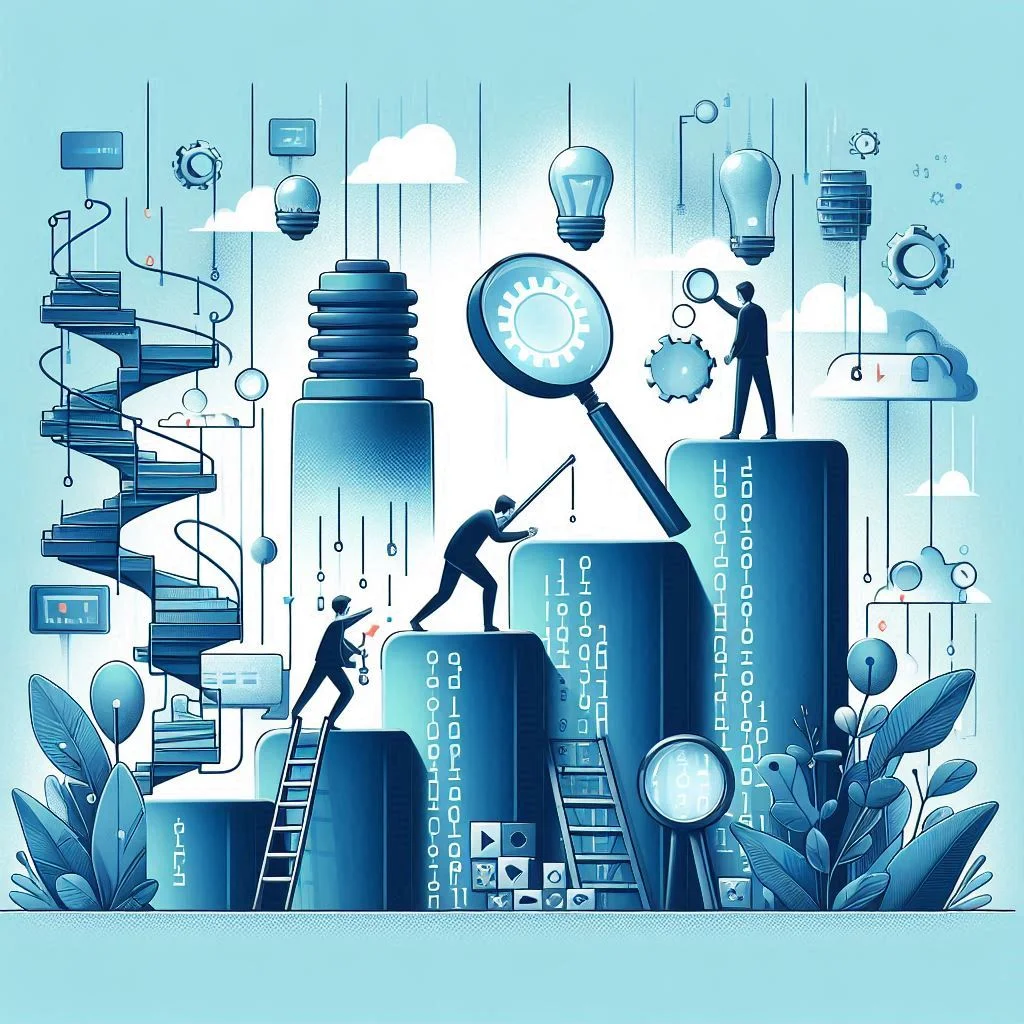
The assignment at hand requires you to code the Insertion Sort algorithm to sort an array of integers and then find the index of a specific value using the Linear Search algorithm. The task is divided into two main parts:
- Insertion Sort Algorithm: Write an assembly language program to sort an array of bytes using the Insertion Sort algorithm.
- Linear Search Algorithm: Use the sorted array to find the index of a given value using the Linear Search algorithm.
Submission Requirements
- File Naming: Name your file SortSearch.asm.
- Documentation: Include your name, student CWU ID, and the honor code in the file.
- Deadline: Submit the file through Canvas before the specified deadline.
Step-by-Step Guide
Step 1: Setup Your Development Environment
Before writing any code, ensure you have the necessary tools:
- Assembler and Emulator: Choose an assembler and an emulator or simulator for your assembly language. This guide assumes you are using the Easy68K assembler, but the steps apply to any assembler.
- Create a New File: Start by creating a new file named SortSearch.asm in your development environment.
Step 2: Plan Your Program Structure
Planning is crucial for any programming task. Break down the assignment into smaller, manageable parts.
- Identify Main Functions: The assignment requires two main functions: Insertion Sort and Linear Search.
- Write Pseudo-Code: Writing pseudo-code helps you understand the logic before translating it into assembly language.
Insertion Sort Pseudo-Code
InsertionSort(array, size)
for i = 1 to size-1
temp = array[i]
j = i - 1
while j >= 0 and array[j] > temp
array[j + 1] = array[j]
j = j - 1
array[j + 1] = temp
Linear Search Pseudo-Code
LinearSearch(array, size, value)
pos = -1
for i = 0 to size-1
if array[i] == value
pos = i
break
return pos
Step 3: Initialize Your Data
In assembly language, data initialization is done through the data segment. Define your array and other variables.
section .data
array db 7, 5, 2, 3, 6 ; Array to be sorted
size db 5 ; Size of the array
value db 6 ; Value to be searched
loc db -1 ; Variable to store the found index
Step 4: Implement Insertion Sort in Assembly
Translate the pseudo-code for the Insertion Sort algorithm into assembly language instructions.
Insertion Sort Assembly Code
- Load Data: Load the array and size into registers.
- Sorting Loop: Implement the sorting loop as per the pseudo-code.
section .text
global _start
_start:
mov ecx, 1 ; i = 1
mov esi, array ; Load address of the array
insertion_sort:
cmp ecx, size
jge search_value ; If i >= size, end sorting
mov al, [esi + ecx] ; temp = array[i]
mov ebx, ecx
dec ebx ; j = i - 1
insert_loop:
cmp ebx, 0
jl insert_done ; If j < 0, exit loop
mov bl, [esi + ebx]
cmp bl, al
jle insert_done ; If array[j] <= temp, exit loop
mov [esi + ebx + 1], bl ; array[j + 1] = array[j]
dec ebx ; j = j - 1
jmp insert_loop
insert_done:
mov [esi + ebx + 1], al ; array[j + 1] = temp
inc ecx ; i = i + 1
jmp insertion_sort
Step 5: Implement Linear Search in Assembly
Translate the pseudo-code for the Linear Search algorithm into assembly language instructions.
Linear Search Assembly Code
1. Search Loop: Implement the search loop as per the pseudo-code.
search_value:
mov ecx, 0 ; i = 0
mov edi, -1 ; pos = -1
linear_search:
cmp ecx, size
jge end_program ; If i >= size, end search
mov al, [esi + ecx]
cmp al, value
jne next_element ; If array[i] != value, continue
mov edi, ecx ; pos = i
jmp end_program
next_element:
inc ecx ; i = i + 1
jmp linear_search
Step 6: Save Result and End Program
Store the result index in the loc variable and ensure the program terminates correctly.
end_program:
mov [loc], edi
; Exit the program
mov eax, 1 ; syscall number (sys_exit)
int 0x80 ; call kernel
Step 7: Test and Debug
- Assemble and Run: Use your assembler to assemble the code and run it to ensure it functions as expected.
- Debugging: Utilize debugging tools to step through the code, identify, and fix any issues.
Step 8: Documentation
Proper documentation is crucial for understanding and maintaining code.
- Add Comments: Include comments in your code to explain each step and logic clearly.
- File Header: Add your name, student CWU ID, and the honor code at the beginning of your file.
assembly
; Name: Your Name
; CWU ID: Your CWU ID
; Honor Code: Your Honor Code
section .data
...
Step 9: Submission
- Review Requirements: Ensure all submission requirements are met, including documentation and file naming.
- Submit on Time: Submit your file through Canvas before the deadline to avoid penalties.
Detailed Breakdown of Each Step
Step 1: Setup Your Development Environment
Setting up your environment involves selecting the right tools. An assembler like Easy68K provides a user-friendly interface for writing and testing assembly language programs. Ensure you have installed it correctly and understand its basic functionality.
Step 2: Plan Your Program Structure
Planning involves understanding the requirements and breaking down the task. Writing pseudo-code helps clarify the logic and sequence of operations. For example, understanding how Insertion Sort works—by iteratively inserting elements into their correct positions—helps translate the process into assembly language.
Step 3: Initialize Your Data
In assembly language, data initialization is crucial. By defining variables in the data segment, you can allocate memory for arrays and other necessary data. This step ensures that your program has access to the data it needs for processing.
Step 4: Implement Insertion Sort in Assembly
Translating the pseudo-code into assembly involves careful handling of registers and memory addresses. Each step of the pseudo-code corresponds to specific assembly instructions:
- Loading Data: Use mov instructions to load data from memory into registers.
- Sorting Loop: Implement loops using cmp, jge, jl, and jmp instructions to control the flow.
Step 5: Implement Linear Search in Assembly
Similar to the sorting algorithm, the Linear Search involves translating the pseudo-code into assembly instructions. The key is to use conditional jumps (jne, je) and loops to iterate through the array and compare each element with the target value.
Step 6: Save Result and End Program
Saving the result involves moving the final index value into a designated memory location. Ending the program correctly is crucial, as it ensures the program terminates gracefully and returns control to the operating system.
Step 7: Test and Debug
Testing and debugging are critical steps to ensure your program works as expected. Assemble your code and run it. Use debugging tools to step through each instruction, check register values, and verify the correctness of your program.
Step 8: Documentation
Good documentation makes your code understandable and maintainable. Comments should explain the purpose of each section of the code, the logic behind loops and conditions, and any tricky parts of the implementation. A file header with your details ensures compliance with submission requirements.
Step 9: Submission
Before submitting, double-check that your file meets all the requirements. Ensure your code is well-documented, the file is correctly named, and it includes your personal information and honour code. Submit the file through the designated platform before the deadline.
Conclusion
By adhering to this comprehensive guide, you can approach any assembly language assignment with increased confidence and clarity. The key to success lies in thoroughly understanding the problem at hand, planning your approach strategically, and systematically implementing and testing your code. Each of these steps plays a crucial role in ensuring that your assignment is completed accurately and efficiently. Whether you’re tasked with sorting an array using Insertion Sort or searching for a specific value with Linear Search, these foundational principles will empower you to tackle any assembly language task with effectiveness. Additionally, practicing these algorithms will enhance your programming skills and deepen your understanding of low-level operations. Utilizing pseudo code to outline your logic can further streamline the coding process, making it easier to translate high-level concepts into assembly language. As you gain experience with these techniques, you will develop a more profound grasp of data structures and algorithmic efficiency, ultimately preparing you for more complex programming challenges. Embrace the learning process and remember that each assignment is an opportunity to sharpen your skills and expand your knowledge in the fascinating world of assembly language programming.