Programming assignments can often be complex, especially when dealing with low-level languages like assembly. To tackle these challenges effectively, it's crucial to follow a structured approach. In this blog, we'll provide a detailed guide on how to approach programming assignments, using a sample assignment to illustrate key strategies. By adhering to these principles, you can produce well-commented, organized, and effective solutions to a variety of programming tasks.
When completing your programming assignments, especially those involving assembly languages such as MARIE, the clarity of your code is paramount. Proper indentation and spacing not only enhance the readability of your code but also make it easier to debug and maintain. Proper formatting helps to ensure that each part of your code is easily identifiable and logically organized.
Programming assignments often require a step-by-step approach to problem-solving. Breaking down a complex problem into smaller, manageable tasks can make it more approachable. For instance, in a MARIE assembly assignment, you might need to write code to handle user inputs, perform calculations, and print results. By using clear indentation and spacing, you ensure that each section of your code is easy to follow, which simplifies the process of identifying and fixing any issues that arise.
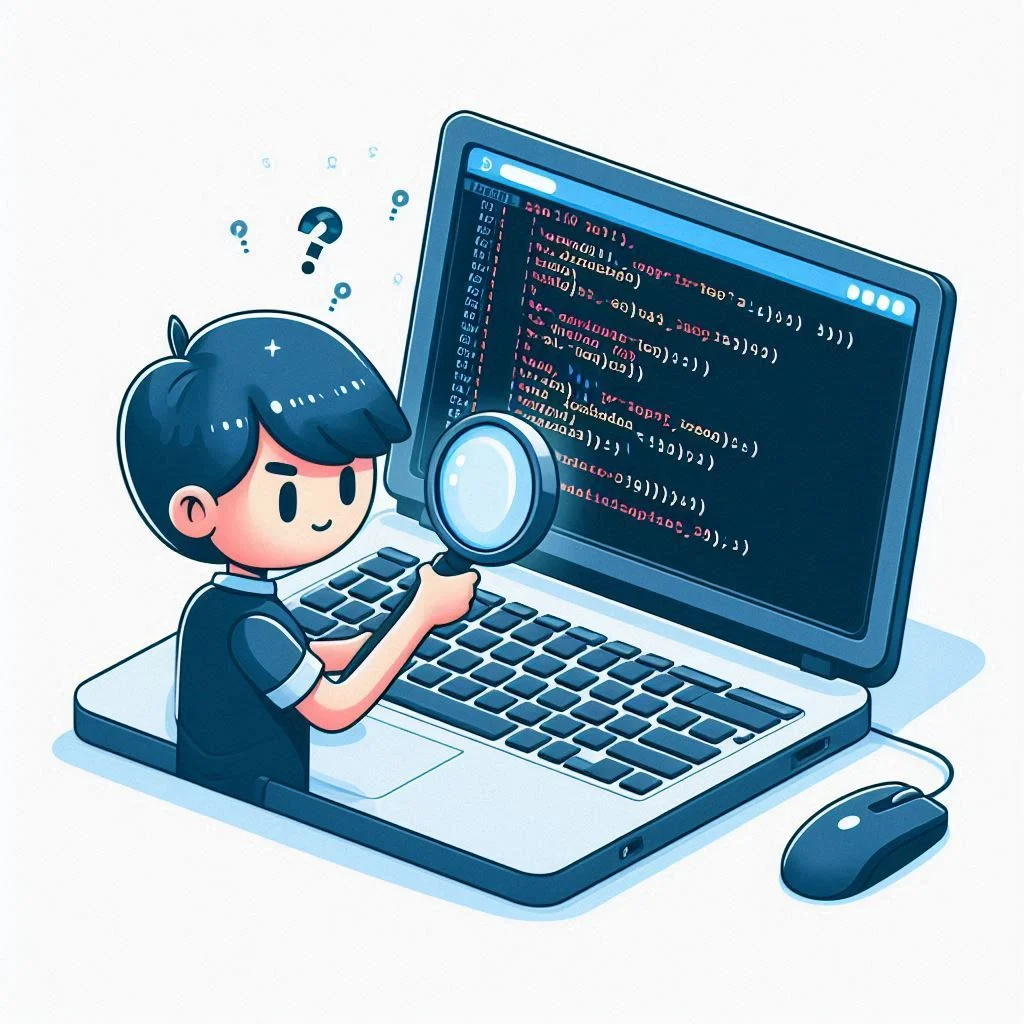
Understanding the Assignment
Before starting any programming task, take the time to thoroughly understand the requirements. Let's consider an example assignment to illustrate this point:
Assignment Example:
- Problem 1: Write MARIE assembly code to sum user inputs until a negative number or a number greater than 100 is entered. Use a subroutine for addition and print the sum. Submit this as problem1.mas.
- Problem 2: Modify the program from Problem 1 to keep track of every number the user enters. Print the sum and every entered number. Save this as problem2.mas.
- Problem 3: Describe and compare the compilation, assembly, and linking processes in detail. This description should be thorough and specific, based on Chapter 8.4 of your textbook.
Submission Requirements:
- Include your name and the assignment name at the top of each file.
- Submit your work in the specified format (Microsoft Word/PDF for descriptions and .mas files for assembly code).
Step-by-Step Solution Approach
To solve programming assignments effectively, follow these systematic steps:
1. Writing Assembly Code
When tasked with writing assembly code, such as in Problem 1 and Problem 2, use the following approach:
a. Plan Your Solution
- Break down the Problem: Start by breaking down the problem into manageable tasks. For Problem 1, the tasks are: taking user input, summing the inputs, and printing the sum.
- Define Variables: Identify and define the necessary variables. Use descriptive names like SUM for the total sum and INPUT for user input.
b. Write the Code
- Start with Input Handling: Begin by writing code to handle user input. In MARIE assembly, you can use IN to read input and OUT to display results.
- Implement Logic: Develop the logic to continuously prompt the user for input until a termination condition is met (negative number or number > 100). Use a subroutine (JNS) for summing the input values.
- Add Comments: Include comments to explain each line or block of code. Comments should clarify why the code is doing what it’s doing, not just repeat instructions.
Example for Problem 1:
ORG 100 / Start program at memory address 100
MAIN, DEC 0 / Variable to hold the sum of inputs
LOOP, IN / Take user input
SKIPCOND 800 / Skip if input is negative or greater than 100
JUMP END / End loop if condition is met
JNS ADD / Jump to subroutine to add input to sum
JMP LOOP / Repeat loop
END, OUT MAIN / Output the sum
HALT / End the program
ADD, CLA / Clear accumulator
ADD MAIN / Add input to sum
STA MAIN / Store updated sum
RET / Return from subroutine
c. Testing and Debugging
- Test Thoroughly: Run your program with different inputs to ensure it behaves as expected. Test edge cases such as negative numbers and numbers just above 100.
- Debug: If your program doesn’t work as expected, use debugging techniques to trace through the code and identify issues. Look for logical errors and ensure your code handles all specified conditions.
2. Modifying and Extending Code
For Problem 2, where you need to track and print each number entered:
a. Extend Your Code
- Additional Variables: Create an additional variable or data structure to store each user input. For example, you might use an array or list if your assembly language supports it.
- Update Logic: Modify the existing loop to store each input value in your data structure and print out all the numbers along with the sum.
Example for Problem 2:
ORG 100 / Start at memory address 100
MAIN, DEC 0 / Variable to hold sum
NUM_LIST, DEC 0 / Variable to hold each number
LOOP, IN / Take user input
SKIPCOND 800 / Skip if input is negative or > 100
JUMP END / End if termination condition met
STA NUM_LIST / Store input in NUM_LIST
JNS ADD / Add input to sum
JMP LOOP / Repeat loop
END, OUT MAIN / Output the sum
/ Print each number in NUM_LIST
HALT
b. Add Comments and Documentation
- Document Changes: Update comments to reflect new variables and logic. Explain why each addition was made and how it fits into the overall program.
3. Describing Compilation, Assembly, and Linking
a. Compilation
- What It Is: Compilation is the process of converting high-level programming code into assembly code or intermediate code. This step is crucial for languages that need to be translated into a lower-level language that the assembler can process.
- Purpose: The purpose of compilation is to transform human-readable code into a form that can be further processed by an assembler or linker. It checks for syntax errors and translates code into an intermediate format.
b. Assembly
- What It Is: Assembly is the process of converting assembly language code into machine code, which is binary code that the computer’s CPU can execute directly.
- Purpose: The purpose of assembling is to create an executable file from assembly code. This step translates the human-readable assembly instructions into machine-readable code.
c. Linking
- What It Is: Linking is the process of combining various pieces of code and data into a single executable file. This process resolves addresses and references between different code modules and libraries.
- Purpose: Linking ensures that all parts of a program are properly combined and that references between different modules are correctly resolved. It prepares the final executable file that can be run on a computer.
Practical Tips for Success
- Organize Your Work: Keep your code and documentation well-organized. Use meaningful variable names and structure your code logically.
- Follow Guidelines: Adhere to any specific guidelines provided in your assignment. This includes formatting requirements and documentation standards.
- Seek Feedback: If possible, get feedback on your code from peers or instructors. This can help you identify areas for improvement and ensure that you’re on the right track.
- Practice Regularly: Regular practice is key to mastering programming skills. Work on a variety of assignments to build your proficiency and confidence.
Conclusion
Approaching programming assignments with a structured and methodical approach can significantly enhance your problem-solving skills. By breaking down the problem, writing well-commented and organized code, and understanding the compilation, assembly, and linking processes, you’ll be well-prepared to tackle similar assignments effectively. Remember to test your solutions thoroughly and seek feedback to continually improve your programming skills.