Programming assignments, especially those involving mathematical computations and data manipulations, often appear daunting at first glance. The complexity of these tasks can lead to uncertainty and hesitation, particularly when students encounter new concepts or methods. However, with a structured approach and systematic breakdown of the problem, tackling such Python assignments becomes not only manageable but also rewarding. This blog aims to provide you with a clear framework for approaching programming assignments in Python, focusing on a practical example. Specifically, we will explore the process of reading a list of numbers, performing calculations to find their sum, mean, and standard deviation, and finally presenting these results effectively. This guide will offer the necessary steps and insights to successfully complete your task.
In the realm of programming, breaking down tasks into smaller, more manageable components is a fundamental strategy for success. Each component serves as a building block that contributes to the overall solution. By understanding the problem statement and identifying the key operations required—such as input handling, computation, and output formatting—you can systematically address the assignment's requirements. This approach not only simplifies the task but also enhances your understanding of how different programming concepts integrate to solve real-world problems.
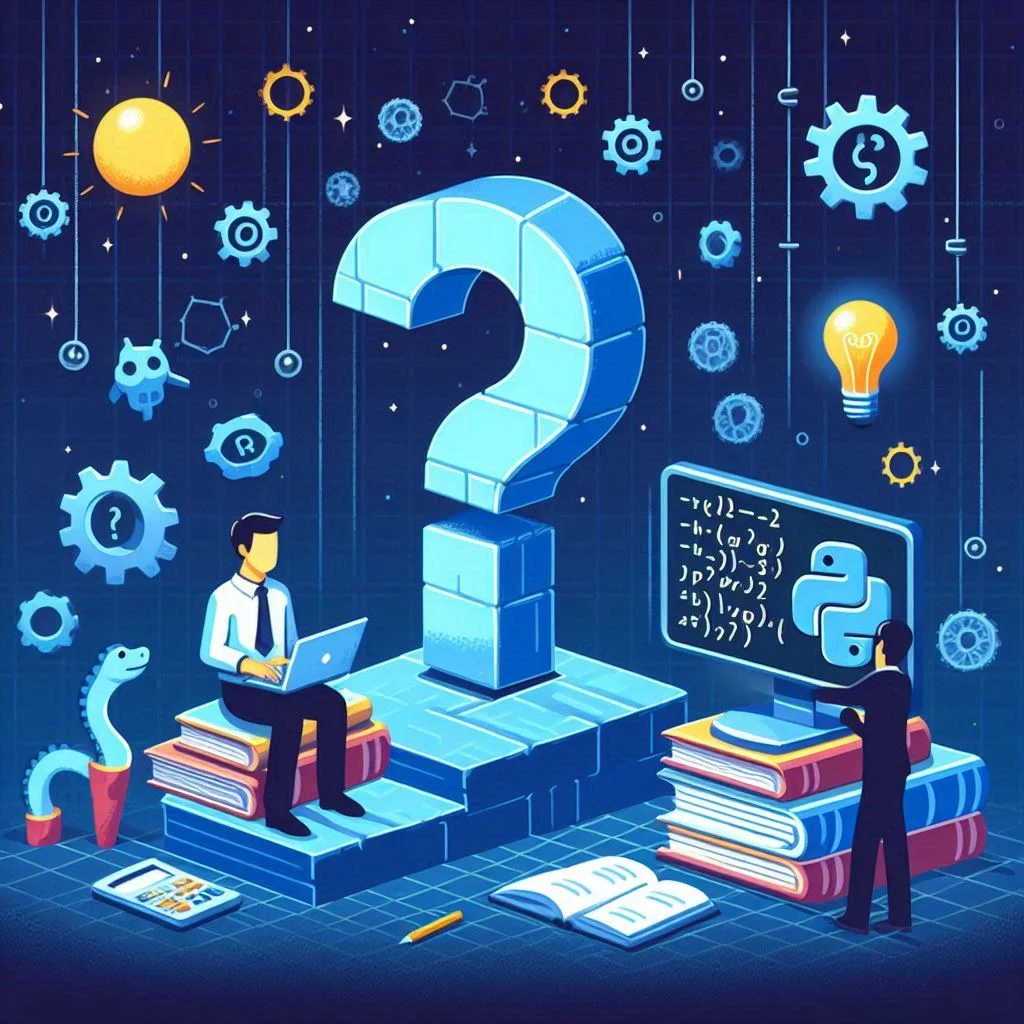
The example assignment provided involves fundamental statistical computations: sum, mean, and standard deviation. These operations are foundational in both programming and data analysis contexts. Learning to execute these calculations programmatically not only sharpens your coding skills but also equips you with valuable tools for future assignments and projects. Python, with its clear syntax and extensive libraries like math for mathematical operations, proves to be an excellent choice for such tasks, enabling concise and efficient implementation of complex algorithms.
Throughout this guide, we will delve into each step of the solution, emphasizing clarity and correctness in coding practices. Understanding how to handle user input, compute mathematical formulas, and present results accurately are essential skills for any programmer. By mastering these techniques, you not only enhance your proficiency in Python programming but also build a strong foundation for tackling a wide range of programming challenges.
By the end of this blog, you will have gained practical insights into solving programming assignments, laying a solid groundwork for future learning and application. Whether you're a beginner seeking to understand the basics or an experienced programmer aiming to refine your skills, this guide aims to empower you with the tools and strategies needed to excel in solving similar programming tasks effectively. Let's embark on this journey to demystify programming assignments and empower your problem-solving capabilities in Python.
Understanding the Problem Statement
The first step in tackling any programming assignment is to thoroughly understand the problem statement. Read the instructions carefully and identify the key tasks required. For instance, in the given example, the assignment requires you to:
- Read a list of numbers from the user.
- Compute the sum of these numbers.
- Calculate the mean (average) of the numbers.
- Determine the standard deviation of the numbers.
- Display the computed sum, mean, and standard deviation.
Understanding these requirements helps in planning the solution effectively.
Breaking Down the Task
Once you understand the problem, the next step is to break it down into smaller, manageable tasks. This modular approach not only simplifies the problem but also makes your code more organized and easier to debug. Here, we can divide the assignment into the following functions:
- read_data(): This function will handle user input and store the numbers in a list.
- compute_sum(list_of_numbers): This function will compute the sum of the numbers in the list.
- compute_mean(list_of_numbers): This function will calculate the mean of the numbers.
- compute_sd(list_of_numbers): This function will compute the standard deviation of the numbers.
- display_result(sum, mean, sd): This function will print the sum, mean, and standard deviation.
Writing the Functions
Let’s dive into writing each function step by step.
Reading Data
The read_data() function is responsible for reading numbers from the user until an empty string is entered. These numbers are then stored in a list.
def read_data():
"""
Prompts user for and reads in a set of numbers, one number at a time.
Returns a list of numbers.
"""
list_of_numbers = []
while True:
nextInt = input('Please enter a number (or press enter to finish): ')
if nextInt == '':
break
try:
list_of_numbers.append(int(nextInt))
except ValueError:
print("Invalid input. Please enter a valid number.")
return list_of_numbers
In this function, we use a while loop to continuously prompt the user for input. If the user enters an empty string, the loop breaks, indicating the end of input. We also include error handling to ensure that only valid integers are added to the list.
Computing the Sum
Next, the compute_sum(list_of_numbers) function calculates the sum of the numbers in the list.
python
def compute_sum(list_of_numbers):
"""
Computes the sum of the list of numbers.
Returns the sum.
"""
return sum(list_of_numbers)
Python's built-in sum() function makes this task straightforward and efficient.
Computing the Mean
The compute_mean(list_of_numbers) function calculates the mean by dividing the sum of the numbers by the length of the list.
def compute_mean(list_of_numbers):
"""
Computes the mean of the list of numbers.
Returns the mean.
"""
return compute_sum(list_of_numbers) / len(list_of_numbers)
Here, we reuse the compute_sum() function to get the sum of the numbers, ensuring that our code is modular and reusable.
Computing the Standard Deviation
The compute_sd(list_of_numbers) function calculates the standard deviation, which is a measure of the amount of variation or dispersion in a set of values.
python
import math
def compute_sd(list_of_numbers):
"""
Computes the standard deviation of the list of numbers.
Returns the standard deviation.
"""
mean = compute_mean(list_of_numbers)
variance = sum((x - mean) ** 2 for x in list_of_numbers) / (len(list_of_numbers) - 1)
return math.sqrt(variance)
This function calculates the variance by summing the squared differences between each number and the mean, then dividing by the number of observations minus one. The standard deviation is the square root of the variance.
Displaying the Results
Finally, the display_result(sum, mean, sd) function prints the computed sum, mean, and standard deviation in a formatted manner.
def display_result(sum, mean, sd):
"""
Prints the sum, mean, and standard deviation.
"""
print(f"Sum: {sum:.2f}")
print(f"Mean: {mean:.2f}")
print(f"Standard Deviation: {sd:.2f}")
Using formatted strings (f-strings) ensures that the results are displayed with two decimal places, making them easy to read.
Integrating the Functions
With all the functions defined, the final step is to integrate them into a main program. The main() function coordinates the execution of all the individual functions.
python
def main():
list_of_numbers = read_data()
sum = compute_sum(list_of_numbers)
mean = compute_mean(list_of_numbers)
sd = compute_sd(list_of_numbers)
display_result(sum, mean, sd)
if __name__ == "__main__":
main()
In this main function, we call read_data() to get the list of numbers, then sequentially compute the sum, mean, and standard deviation, and finally display the results.
Debugging and Testing
A crucial part of solving programming assignments is debugging and testing your code. Here are some tips to help you debug and test effectively:
- Test Incrementally: Test each function individually before integrating them. This helps isolate any errors and makes debugging easier.
- Use Print Statements: Add print statements to check the values of variables at different stages of your program. This can help you understand the flow of data and identify where things might be going wrong.
- Edge Cases: Consider edge cases, such as an empty list, a list with a single number, or very large numbers. Ensure your program handles these cases gracefully.
- Code Review: If possible, have someone else review your code. A fresh set of eyes can often spot mistakes that you might have overlooked.
Error Handling and User Input Validation
Robust programs include error handling to manage unexpected inputs or situations. In our read_data() function, we included a try-except block to handle invalid inputs. Here’s a more detailed example:
def read_data():
"""
Prompts user for and reads in a set of numbers, one number at a time.
Returns a list of numbers.
"""
list_of_numbers = []
while True:
nextInt = input('Please enter a number (or press enter to finish): ')
if nextInt == '':
break
try:
list_of_numbers.append(int(nextInt))
except ValueError:
print("Invalid input. Please enter a valid number.")
return list_of_numbers
This approach ensures that the user is prompted to enter valid numbers, enhancing the robustness of the program.
Documentation and Code Comments
Good documentation and code comments are essential for making your code understandable to others and to your future self. Use docstrings to describe the purpose and parameters of each function. Inline comments can explain complex or non-obvious parts of the code.
For example:
def compute_sd(list_of_numbers):
"""
Computes the standard deviation of the list of numbers.
Returns the standard deviation.
"""
mean = compute_mean(list_of_numbers)
# Calculate variance
variance = sum((x - mean) ** 2 for x in list_of_numbers) / (len(list_of_numbers) - 1)
# Standard deviation is the square root of the variance
return math.sqrt(variance)
Enhancing and Extending the Program
Once you have a working solution, consider ways to enhance or extend the program. For example, you might add functionality to:
- Save Results to a File: Allow users to save the results to a text file for future reference.
- Plot Data: Use libraries like Matplotlib to create visualizations of the data and computed statistics.
- Handle More Statistical Measures: Extend the program to compute other statistical measures, such as the median or mode.
- Graphical User Interface (GUI): Create a GUI using Tkinter or another framework to make the program more user-friendly.
Practice and Continuous Learning
Programming is a skill that improves with practice. Continuously challenge yourself with new assignments and projects to build your problem-solving abilities and coding skills. Explore online resources, coding communities, and tutorials to learn new techniques and best practices.
Conclusion
In conclusion, successfully navigating Python programming assignments requires a methodical approach to problem-solving. Breaking down tasks into manageable components, writing clear and modular code, and diligently testing and debugging are essential steps in crafting robust solutions. By adhering to these principles, you can confidently tackle assignments that involve tasks such as data input handling, mathematical computations like sum and mean calculations, and the calculation of standard deviation. It's crucial to prioritize thorough documentation of your code to ensure clarity for both you and others who may review or use your work. Additionally, embracing a mind-set of continuous learning and improvement is key to advancing your skills as a programmer. Regular practice and exposure to diverse programming challenges will sharpen your abilities and pave the way towards becoming a proficient programmer.
In summary, approaching Python programming assignments effectively hinges on a structured methodology and attention to detail. By following the steps outlined in this blog, you will be well-equipped to tackle a variety of programming tasks with confidence and precision. Remember, each assignment is an opportunity to refine your coding skills and deepen your understanding of Python's capabilities. Embrace challenges as learning opportunities, and always strive for clarity, correctness, and efficiency in your code. With persistence and dedication, you'll build a strong foundation for success in your programming journey.