In the realm of computer science, solving problems involving data structures and algorithms forms the bedrock of proficiency for programmers. Among these challenges, the word ladder problem stands out as a fascinating exercise in transformation and traversal. Imagine starting with one word and gradually transforming it into another, changing only one letter at a time, with each intermediate step forming a valid word. This task not only requires ingenuity in algorithm design but also necessitates the use of efficient data structures to manage and query a large set of words effectively.
The word ladder problem is not merely an academic exercise but finds practical applications in fields ranging from natural language processing to game development. By mastering this problem, programmers gain insights into graph theory and traversal algorithms, crucial for optimizing performance in applications involving large datasets. Moreover, the ability to craft efficient solutions underscores the importance of choosing the right data structures, such as hash tables, which can store and retrieve data swiftly, crucial for real-time applications.
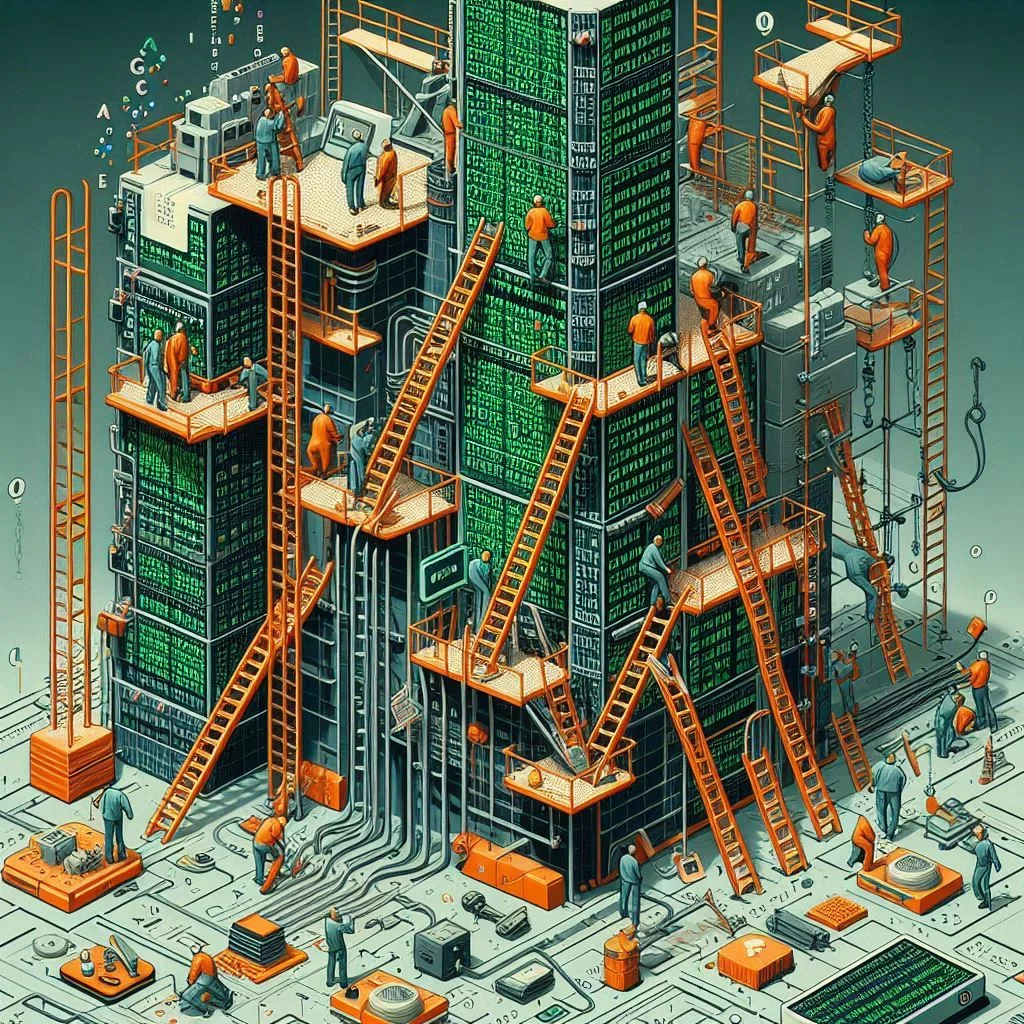
In this blog post, we delve into solving the word ladder problem using a custom WordSet implemented with Cuckoo hashing, a robust technique known for handling collisions in hash tables efficiently. We'll explore how Cuckoo hashing enables us to maintain a dynamic set of words, crucial for validating word transformations during the ladder-solving process. Additionally, we'll leverage the Breadth-First Search (BFS) algorithm, renowned for finding the shortest path in unweighted graphs, to discover the minimal transformation sequence between two words efficiently. This approach not only exemplifies practical problem-solving in programming but also deepens understanding of foundational concepts that underpin computational efficiency.
This blog will be beneficial for university students also who want to take assistance in solving their C++ Assignments.
Problem Breakdown
The word ladder problem can be summarized as follows: given two words, such as "head" and "tail", transform "head" into "tail" by changing one letter at a time, with each intermediate word being valid and present in a given dictionary.
To solve this problem effectively, we'll employ two main strategies:
1. Implementing a Custom WordSet:
- Instead of relying on standard library data structures, we'll create our own WordSet using Cuckoo hashing. This involves designing a hash table capable of handling collisions efficiently.
2. Using BFS for Pathfinding:
- We'll utilize the Breadth-First Search (BFS) algorithm to find the shortest transformation sequence (word ladder) from the start word to the end word. BFS is particularly suited for this task as it explores all neighbors (words differing by one letter) before moving on to the next level of neighbors.
Implementing the Word Set with Cuckoo Hashing
To begin, let's delve into the implementation details of our custom WordSet using Cuckoo hashing.
Understanding Cuckoo Hashing
Cuckoo hashing is a technique that uses two hash tables to store keys (words, in our case) to handle collisions efficiently. Here’s a step-by-step approach to implementing it:
1. Hash Function Implementation:
- The first step is to design a hash function that maps strings (words) to indices in the hash tables. For our purpose, a polynomial hash function is suitable. This function interprets the string as coefficients of a polynomial and evaluates it at a specified base, using modulus to fit within the table size.
size_t polynomialHashFunction(const std::string& str, unsigned base, unsigned mod) {
size_t hashValue = 0;
unsigned power = 1;
for (char c : str) {
hashValue = (hashValue + (c - 'a' + 1) * power) % mod;
power = (power * base) % mod;
}
return hashValue;
}
2. Cuckoo Hash Table Structure:
- Implement a WordSet class using two dynamically allocated arrays (T1 and T2) to handle collisions. When inserting a new word, if the primary slot (T1) is occupied, evict the existing word into the secondary slot (T2). If collisions persist beyond a threshold (EvictThreshold), resize the hash tables to accommodate more entries.
template
class BaseWordSet {
private:
std::string* T1;
std::string* T2;
size_t capacity;
size_t size;
void resize(size_t newCapacity);
public:
BaseWordSet(size_t initialCapacity);
~BaseWordSet();
void insert(const std::string& word);
bool contains(const std::string& word) const;
size_t size() const noexcept;
size_t capacity() const noexcept;
void erase(const std::string& word);
};
3. Operations on WordSet:
- Implement essential operations like insert, contains, size, capacity, and erase to manage the WordSet efficiently. These methods ensure that operations like insertion and lookup are optimized, adhering to the principles of Cuckoo hashing for collision resolution.
Solving the Word Ladder Problem
Having established our custom WordSet, let’s now focus on solving the word ladder problem using BFS.
BFS Algorithm for Word Ladder
BFS is well-suited for finding the shortest path in an unweighted graph, making it ideal for our word ladder problem where each word transformation represents an edge between vertices (words).
1. Graph Representation:
- Treat words as vertices in a graph, where an edge exists between two vertices (words) if they differ by exactly one letter. This graph representation helps in visualizing the problem and formulating our BFS approach.
2. BFS Implementation:
- Start from the start word and explore all its neighboring words (words that differ by one letter). Use a queue to manage the exploration and a map (previousWord) to track the path from start to end.
template
std::vector
convert(const std::string& start, const std::string& end, const BaseWordSet& words) {
std::queue
queue;
std::unordered_map previousWord;
queue.push(start);
previousWord[start] = "";
while (!queue.empty()) {
std::string current = queue.front();
queue.pop();
// Generate all words that are one letter away from 'current'
for (size_t i = 0; i < current.size(); ++i) {
std::string next = current;
for (char c = 'a'; c <= 'z'; ++c) {
if (c == current[i]) continue;
next[i] = c;
if (words.contains(next) && previousWord.find(next) == previousWord.end()) {
queue.push(next);
previousWord[next] = current;
if (next == end) {
// Reconstruct the path from 'start' to 'end'
std::vector
ladder;
std::string word = end;
while (!word.empty()) {
ladder.push_back(word);
word = previousWord[word];
}
std::reverse(ladder.begin(), ladder.end());
return ladder;
}
}
}
}
}
// If no path found
return std::vector
();
}
3. Path Reconstruction:
- Once BFS reaches the end word, reconstruct the word ladder path using the previousWord map, which stores the parent-child relationships between words encountered during BFS traversal.
Conclusion
In conclusion, solving the word ladder problem with Cuckoo hashing and BFS exemplifies the synergy between data structures and algorithms in problem-solving. By implementing a custom WordSet tailored to handle collisions efficiently using Cuckoo hashing, and employing BFS to find the shortest transformation sequence, we've demonstrated a robust approach to solving complex problems in programming. This methodology not only enhances understanding of foundational concepts but also equips programmers with valuable skills applicable across various domains. Moving forward, exploring different configurations of Cuckoo hashing parameters and adapting algorithms for different problem variations will further deepen insights and proficiency in computational problem-solving