The Selection Problem, which involves finding the kth smallest element in a list, is a classic algorithmic challenge with several potential solutions. This blog will guide you through solving similar types of assignments by providing a general approach that is applicable to various problems. We will focus on designing, implementing, and analyzing algorithms to address these challenges effectively. By understanding the different strategies and their complexities, you'll be equipped to tackle various programming assignments with confidence. The process includes learning about different algorithmic techniques, such as sorting and partitioning, and applying them to efficiently solve the problem at hand. This comprehensive approach will not only help you in managing selection problems but also enhance your overall problem-solving skills in programming assignments. Whether you are dealing with basic or advanced algorithmic tasks, this guide will provide the essential tools and knowledge needed to excel in your Algorithms assignments and improve your programming capabilities.
Understanding the Problem
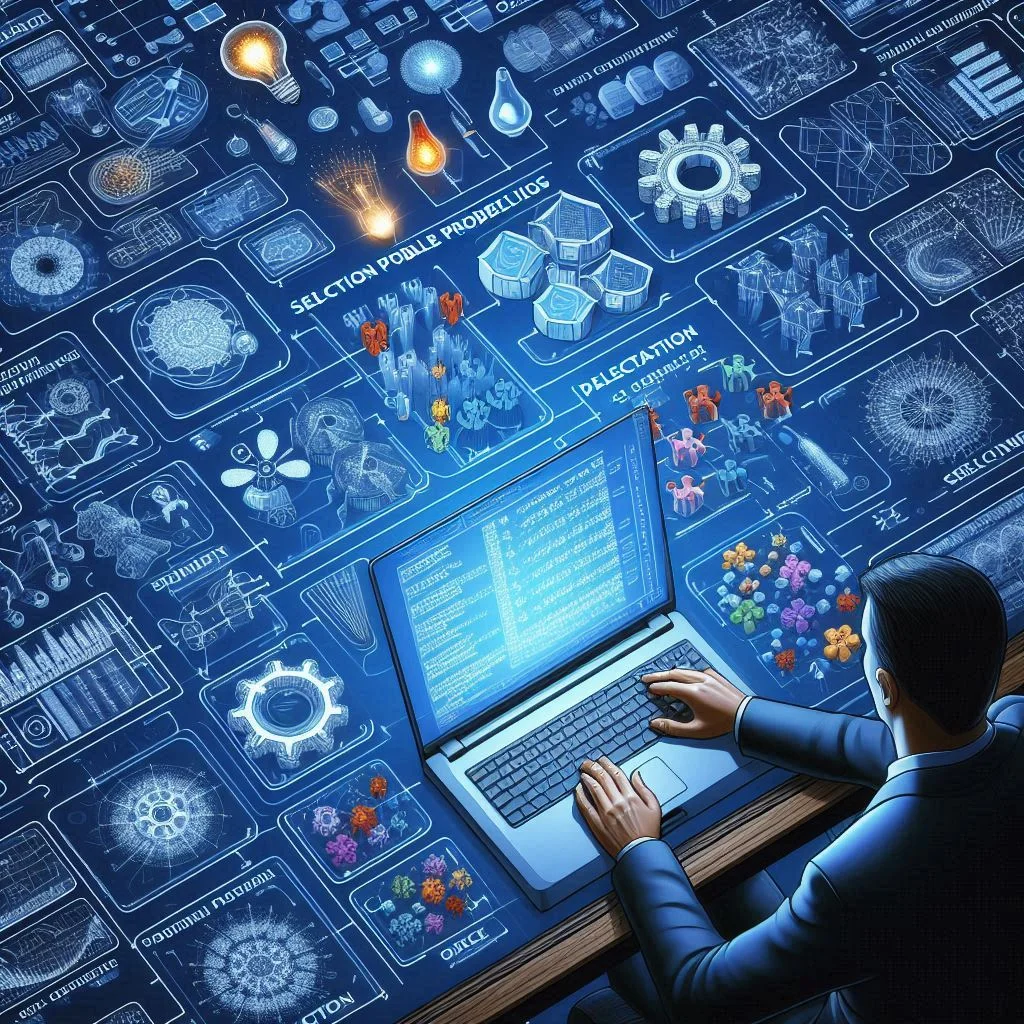
The Selection Problem requires finding the kth smallest element in an unsorted list of n numbers. This problem can be approached using different algorithms, each with its own time complexity and characteristics. Here, we will outline three key algorithms commonly used to solve this problem:
- Sorting-Based Selection (Algorithm 1)
- Partition-Based Selection (Algorithm 2)
- Optimized Partition-Based Selection with Medians of Medians (Algorithm 3)
General Approach to Solving Selection Problems
1. Design and Theoretical Analysis
1. Analyzing Algorithmic Complexity
To approach any selection problem, start by analyzing the theoretical worst-case complexity of each algorithm you plan to use:
- Sorting-Based Selection (Algorithm 1): This algorithm sorts the entire list first and then retrieves the kth smallest element. The time complexity is O(nlogn)O(n \log n)O(nlogn), where n is the number of elements in the list. The worst-case input is typically an unsorted list of size n.
- Partition-Based Selection (Algorithm 2): This approach involves partitioning the list using a pivot element (like in Quicksort) and recursively narrowing down the search to the relevant subarray. The average time complexity is O(n)O(n)O(n), but the worst-case complexity is O(n2)O(n^2)O(n2) if the pivot choices are poor. The worst-case input often involves unbalanced partitions.
- Optimized Partition-Based Selection (Algorithm 3): This method improves upon the basic partitioning by using the Medians of Medians technique to choose a better pivot. The time complexity is O(n)O(n)O(n) in the worst case. The worst-case input is similar to Algorithm 2 but with a more robust pivot selection process.
Here’s a table summarizing the theoretical complexities:
2. Designing the Program
For each algorithm, create pseudo code or flowcharts to outline the steps:
Algorithm 1 (Sorting-Based Selection):
- Sort the list using Merge Sort.
- 2Return the element at the kth position.
Pseudocode:
function findKthSmallestSort(arr, k):
sortedArr = mergeSort(arr)
return sortedArr[k-1]
Algorithm 2 (Partition-Based Selection):
1. Choose a pivot and partition the list.
2. Recursively apply the partitioning based on the kth position.
3. Return the element when the pivot position matches k.
Pseudocode:
function partition(arr, low, high):
pivot = arr[high]
i = low - 1
for j = low to high-1:
if arr[j] <= pivot:
i += 1
swap arr[i] and arr[j]
swap arr[i+1] and arr[high]
return i+1
function quickSelect(arr, low, high, k):
if low == high:
return arr[low]
pivotIndex = partition(arr, low, high)
if k == pivotIndex:
return arr[k]
else if k < pivotIndex:
return quickSelect(arr, low, pivotIndex-1, k)
else:
return quickSelect(arr, pivotIndex+1, high, k)
Algorithm 3 (Optimized Partition-Based Selection):
- Use the Medians of Medians technique to select a pivot.
- Apply the partitioning algorithm similar to Algorithm 2.
- Recursively narrow down until the kth position is found.
Pseudocode:
function medianOfMedians(arr, left, right):
numMedians = (right - left + 4) / 5
medians = []
for i = 0 to numMedians - 1:
subLeft = left + i * 5
subRight = min(subLeft + 4, right)
medians.append(median(arr[subLeft:subRight+1]))
return quickSelect(medians, 0, numMedians - 1, numMedians // 2)
function optimizedQuickSelect(arr, left, right, k):
if left == right:
return arr[left]
pivotIndex = partition(arr, left, right, medianOfMedians(arr, left, right))
if k == pivotIndex:
return arr[k]
else if k < pivotIndex:
return optimizedQuickSelect(arr, left, pivotIndex - 1, k)
else:
return optimizedQuickSelect(arr, pivotIndex + 1, right, k)
3. Designing Testing Cases
Develop at least 10 test cases to ensure the correctness of your algorithms. Consider a variety of scenarios, including edge cases and typical cases
4. Designing Testing Strategy
To ensure robust testing:
- Generate Random Inputs: Use a random number generator to create various input lists.
- Run Multiple Trials: Execute each algorithm multiple times (e.g., 10) to account for variability in runtime.
- Compute Average Runtime: Calculate the average runtime excluding the best and worst runs to get a reliable performance measure.
Example Strategy:
- Random Input Generation: Use Python or another language to create random lists of different sizes.
- Multiple Trials: Run each algorithm on the same input multiple times, e.g., 10 trials.
- Average Runtime Calculation:
def average_runtime(times):
times.sort()
return sum(times[1:-1]) / (len(times) - 2)
2. Implementation
1. Coding the Algorithms
Implement the algorithms in your chosen programming language. For Example you can use Java, C++. Ensure that each program is well-documented and adheres to the design specifications from Part 1.
Java Example for Algorithm 1:
import java.util.Arrays;
public class SelectionAlgorithm1 {
public static int findKthSmallest(int[] arr, int k) {
Arrays.sort(arr);
return arr[k - 1];
}
public static void main(String[] args) {
int[] arr = {3, 1, 4, 1, 5};
int k = 3;
System.out.println("The " + k + "th smallest element is " + findKthSmallest(arr, k));
}
}
C++ Example for Algorithm 2:
#include < iostream >
#include < vector >
#include < algorithm >
using namespace std;
int partition(vector& arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] <= pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return i + 1;
}
int quickSelect(vector& arr, int low, int high, int k) {
if (low == high) return arr[low];
int pivotIndex = partition(arr, low, high);
if (k == pivotIndex) return arr[k];
else if (k < pivotIndex) return quickSelect(arr, low, pivotIndex - 1, k);
else return quickSelect(arr, pivotIndex + 1, high, k);
}
int main() {
vector arr = {3, 1, 4, 1, 5};
int k = 3;
cout << "The " << k << "th smallest element is " << quickSelect(arr, 0, arr.size() - 1, k - 1) << endl;
return 0;
}
2. Testing and Debugging
Run your implementations using the designed test cases. Verify correctness by checking if the outputs match the expected results. Debug any issues that arise to ensure all algorithms function as intended.
Example Test in Java:
public class TestSelectionAlgorithms {
public static void main(String[] args) {
int[][] testCases = {
{3, 1, 4, 1, 5},
{10, 20, 30},
{7, 10, 4, 3, 8},
// add more test cases
};
int[] ks = {3, 2, 4};
for (int i = 0; i < testCases.length; i++) {
System.out.println("Test Case " + (i + 1));
System.out.println("Input: " + Arrays.toString(testCases[i]));
System.out.println("k: " + ks[i]);
System.out.println("Output: " + SelectionAlgorithm1.findKthSmallest(testCases[i], ks[i]));
// Test other algorithms similarly
}
}
}
3. Documentation and Screenshots
Capture screenshots of the execution for each algorithm to demonstrate proper functionality. Include these in your final submission. Annotate the screenshots to highlight the important parts of the output.
Example Documentation:
1. Algorithm 1 (Sorting-Based Selection):
- Screenshot of the program running with test cases.
- Annotations explaining the input, expected output, and actual output.
2. Algorithm 2 (Partition-Based Selection):
- Similar to Algorithm 1, but with its own specific annotations.
3. Algorithm 3 (Optimized Partition-Based Selection):
- Include explanations for Medians of Medians pivot selection.
3. Comparative Analysis
1. Running Experiments
Run each algorithm on the randomly generated inputs. Record the average runtimes for varying input sizes. Use a consistent environment to ensure fairness in the comparison.
Example Experiment Setup:
- Input Sizes: 100, 1000, 10000, 100000
- Random Input Generation:
import random
def generate_random_list(size):
return [random.randint(1, 1000000) for _ in size]
3. Run Each Algorithm:
import time
def measure_runtime(algorithm, input_list, k):
start_time = time.time()
algorithm(input_list, k)
return time.time() - start_time
input_sizes = [100, 1000, 10000, 100000]
algorithms = [algorithm1, algorithm2, algorithm3]
results = {alg: [] for alg in algorithms}
for size in input_sizes:
input_list = generate_random_list(size)
for alg in algorithms:
runtimes = [measure_runtime(alg, input_list, k) for _ in range(10)]
results[alg].append(average_runtime(runtimes))
2. Analyzing Results
- Create Performance Tables: Summarize the average runtimes for each algorithm and input size.
- Plot Graphs: Generate graphs to visually compare the performance of each algorithm. Plot the results on the same graph to facilitate comparison.
- Explain Differences: Discuss why certain algorithms perform better or worse based on their complexity and implementation.
Example Graph:
- Plot runtime on the y-axis and input size on the x-axis.
- Use different colors or markers for each algorithm.
Explanation:
- Algorithm 1 (Sorting-Based Selection) shows higher runtimes due to the O(nlogn)O(n \log n)O(nlogn) complexity.
- Algorithm 2 (Partition-Based Selection) is faster on average but can be slower in worst-case scenarios.
- Algorithm 3 (Optimized Partition-Based Selection) performs best due to the robust pivot selection.
3. Compare Theoretical and Empirical Results
Compare the theoretical complexities with the empirical results from your experiments. Explain any discrepancies and possible reasons for performance differences.
Example Analysis:
- Algorithm 1: The O(nlogn)O(n \log n)O(nlogn) complexity is evident in the empirical results, especially for larger input sizes.
- Algorithm 2: The average-case performance is close to O(n)O(n)O(n), but occasional worst-case scenarios may lead to higher runtimes.
- Algorithm 3: Consistently close to O(n)O(n)O(n) due to the effective pivot selection.
Possible Discrepancies:
- Variations in hardware performance.
- Overhead from programming language runtime environments.
- Differences in implementation details.
4. Specifying Computing Environment
Provide details about your computing environment, including hardware and software specifications, to contextualize your experimental results.
Example Environment Specification:
- Hardware: Intel Core i7-9700K, 16GB RAM, 512GB SSD
- Software: Ubuntu 20.04, Python 3.8, Java 11, GCC 9.3
- Development Tools: VS Code, Jupyter Notebook
5. Concluding the Report
Reflect on the strengths and constraints of your project. Discuss what you would do differently if given another chance, considering aspects like computing environment, programming language, and data set generation.
Conclusion:
This project provided valuable insights into solving the Selection Problem using different algorithms, highlighting each approach's strengths and weaknesses and confirming theoretical complexities with empirical data. Given another opportunity, improvements could include using a larger and more diverse set of test cases, exploring additional optimization techniques, and conducting experiments on multiple platforms for a broader perspective. By following this guide, you can effectively tackle selection problems and similar algorithmic assignments. Understanding the theoretical background, implementing robust algorithms, and conducting thorough testing and analysis are crucial steps in solving such problems. With this structured approach, you’ll be well-prepared to handle a range of algorithmic challenges. Feel free to reach out if you need further assistance with your assignments or have specific questions about implementing these algorithms.