Programming assignments can often feel overwhelming, especially when they involve complex concepts like two's complement operations, multiplication algorithms, and version control systems like Git. To effectively tackle these assignments, it’s crucial to break them down into manageable parts. If you find yourself struggling, seeking Assembly Language Homework Help can provide valuable insights. Begin by thoroughly reading the assignment prompt to understand the requirements and specific tasks involved. Next, organize your approach by outlining the necessary functions, such as addition, subtraction, and multiplication using two's complement. Pseudo code can be invaluable here, allowing you to visualize the logic before coding. Set up your development environment correctly, utilizing provided tools like Make files for efficient building. As you code, maintain good practices by commenting your work and using consistent naming conventions. Finally, don’t underestimate the importance of testing your code rigorously to ensure its correctness. By following these structured strategies, you’ll transform what seems like a daunting task into a manageable project, setting yourself up for success.
Understanding Two's Complement
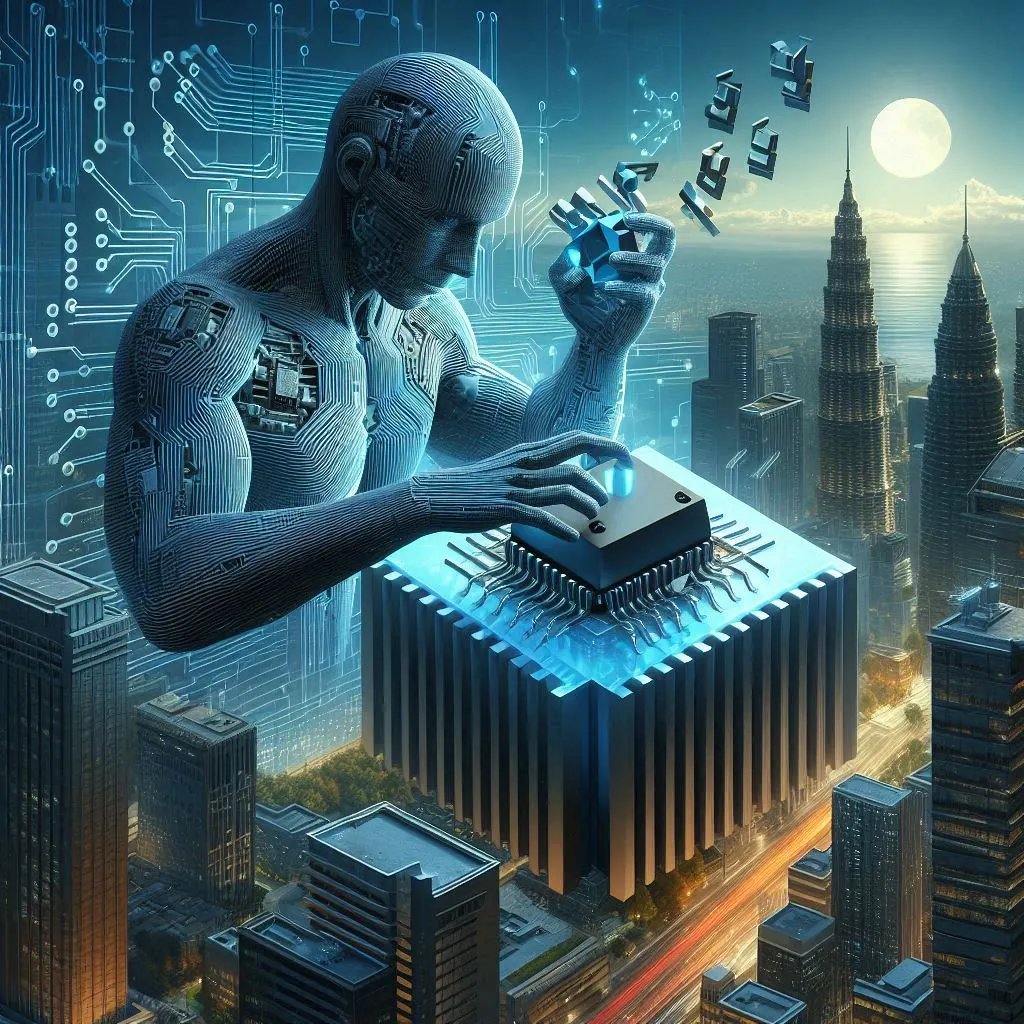
Before diving into specific assignment strategies, it's essential to understand what two's complement is and why it matters. Two's complement is a mathematical representation used in computing for signed integers, which allows for straightforward implementation of addition, subtraction, and other arithmetic operations. Here’s a brief overview of essential operations:
1. Addition
Adding two two's complement numbers is relatively straightforward. The binary addition rules apply, and the result yields the expected value. Importantly, overflow occurs only if the result has a different sign than the operands being added. This characteristic makes two's complement a reliable system for representing signed integers in binary.
2. Subtraction
To subtract one two's complement number (the subtrahend) from another (the minuend), you need to negate the subtrahend using its two's complement and then add it to the minuend. This negation simplifies subtraction operations and fits seamlessly into the overall binary addition framework.
3. Multiplication
For multiplication, Booth’s multiplication algorithm is often utilized when dealing with two's complement numbers. This algorithm leverages shifting and adding based on specific conditions, making it effective for multiplying binary numbers without complex circuitry. Understanding how Booth’s algorithm works can significantly aid in implementing multiplication functions in your assignments.
4. Division
When it comes to division, one effective approach is to convert operands into unsigned values and perform the unsigned division operation. Afterward, you can adjust the signs as needed to account for the original signed values. This method is crucial for maintaining the integrity of results in signed division operations.
Steps to Approach the Assignment
1. Read and Understand the Requirements
The first step in any programming assignment is to read the prompt carefully. Understanding what is expected of you is crucial. Identify the operations you need to implement and any specific algorithms mentioned. Look for sections that define input and output requirements, as well as any constraints or specific behaviors that must be adhered to.
2. Break Down the Problem
Once you have a clear understanding of the requirements, divide the assignment into smaller, manageable parts. For instance, if you need to implement functions for addition, subtraction, multiplication, and division, create separate tasks for each of these. This method prevents feeling overwhelmed by the larger project and allows for a more focused approach to coding.
- Implementing Functions: Identify the specific functions you need to create (e.g., add_twos_complement, subtract_twos_complement). Each function should perform a single task clearly and efficiently.
- Directory Structure: Follow the specified directory structure laid out in the assignment. This includes organizing your code into src, include, and bin folders, which helps maintain a clean and manageable project.
3. Plan Your Implementation
Before you start coding, outline your implementation strategy. Planning can save you significant time and prevent potential errors down the line.
- Pseudocode: Write pseudocode for each function to clarify your logic before actual coding. For example, outline the steps for your addition function using two's complement logic. This step helps identify potential pitfalls early in the process.
function add_twos_complement(num1, num2):
result = num1 + num2
if overflow occurs:
handle overflow
return result
- Flowcharts: If applicable, create flowcharts to visualize complex algorithms like Booth’s multiplication. This visual representation can provide insight into the algorithm's workings and assist with understanding how to translate that logic into code.
4. Set Up Your Development Environment
Utilize the provided Make file to manage your build process efficiently. The Make file typically contains all the necessary commands to compile, link, and clean your project. Familiarize yourself with how to run these commands, as they will be integral to the development process.
- Compile and Build: Use commands like make to build your project and make clean to remove any previously built artifacts. This organization helps prevent conflicts and ensures a clean working environment.
5. Coding Best Practices
While coding, adhere to best practices that enhance code quality and maintainability:
- Comment Your Code: Use comments to explain your logic and maintain readability. Include your name and student number in each file’s header comment, which serves as a good practice in academic settings.
// Author: Your Name
// Student Number: 123456
// Description: Implementation of two's complement addition
- Consistent Naming Conventions: Follow consistent naming conventions for functions and variables to enhance clarity. For example, use add_twos_complement instead of something generic like add.
- Error Handling: Implement error handling where necessary to prevent unexpected behavior. For instance, check for overflow conditions in your addition and subtraction functions to ensure the program behaves predictably.
6. Testing and Debugging
After implementing your functions, thorough testing and debugging are essential to ensure correctness:
- Unit Testing: Write tests for each function to verify that they work correctly. Consider using sample inputs that include edge cases, such as positive and negative numbers, to ensure comprehensive coverage.
- Debugging: Utilize debugging tools (like gdb) to step through your code and identify issues. Debugging is crucial for understanding where your implementation might diverge from expected behavior.
7. Version Control with Git
Employ Git effectively to manage your project:
- Frequent Commits: Make regular commits with meaningful messages. This practice helps track progress and changes and allows you to revert to previous versions if necessary. For example, after completing the addition function, you might use:
git add src/add.c
git commit -m "Implemented add_twos_complement function"
- Branching: Consider using branches for significant changes or feature implementations to avoid disrupting the main codebase. This strategy allows you to experiment without affecting the stable version of your project.
8. Finalize and Document
Before submission, ensure you have completed all necessary documentation and code clean-up:
- Complete the README: Your README file should provide an overview of the project, technologies used, how to build and run the project, and a list of contributors. This document is crucial for any future users or evaluators of your code.
- Clean Code: Before submitting, remove any debugging print statements and commented-out code. Clean code enhances readability and professionalism in your submission.
Common Challenges and Solutions
Challenge: Understanding Algorithms
Solution: Spend time studying the underlying algorithms. Look for resources or documentation that clearly explain the steps involved in the algorithm. Online tutorials, videos, and textbooks can be incredibly helpful.
Challenge: Managing Time
Solution: Start early and break the assignment into smaller tasks with deadlines for each part. This approach helps manage time effectively and reduces the risk of last-minute stress.
Challenge: Git Confusion
Solution: Familiarize yourself with basic Git commands. Use resources like Git cheat sheets or tutorials to remind yourself of the necessary commands. Practicing on simple projects can also build your confidence.
Example Workflow
To illustrate these concepts, let’s consider a hypothetical workflow for completing a programming assignment on two's complement arithmetic.
Step 1: Understanding Requirements
You receive an assignment to implement addition, subtraction, multiplication, and division functions for two's complement numbers, alongside using Git for version control.
Step 2: Breaking Down the Problem
You decide to tackle the functions one by one:
- Implement add_twos_complement
- Implement subtract_twos_complement
- Implement Booth's algorithm for multiplication
- Implement division using unsigned division
Step 3: Planning Implementation
You draft pseudocode for addition:
function add_twos_complement(num1, num2):
result = num1 + num2
if overflow occurs:
handle overflow
return result
Step 4: Setting Up Environment
You check the provided Make file and verify that it includes all necessary flags and commands for building the project. You familiarize yourself with running make and make clean.
Step 5: Coding
You start coding, ensuring to comment your functions and follow naming conventions. For example:
// Function to add two two's complement numbers
int add_twos_complement(int a, int b) {
int sum = a + b;
if ((sum < a) != (b < 0)) { // Check for overflow
// Handle overflow
}
return sum;
}
Step 6: Testing
After coding, you write unit tests for your addition function, checking typical cases and edge cases.
Step 7: Version Control
You commit your changes regularly with meaningful messages, creating branches as needed for larger features.
Step 8: Finalization
Before submission, you finalize your README, ensure your code is clean, and remove any unnecessary print statements.
Conclusion
Tackling programming assignments that involve two's complement and related operations can initially seem daunting, but employing a structured approach significantly simplifies the process. Breaking down the problem into smaller, manageable tasks is the first step. Begin by thoroughly understanding the assignment requirements, identifying the specific operations needed, such as addition, subtraction, multiplication, and division using two's complement.
Following best coding practices is crucial throughout the development process. This includes using meaningful variable names, writing clear comments to explain complex sections of code, and ensuring consistency in coding style and formatting. Utilizing version control systems like Git allows for efficient tracking of changes, facilitating collaboration and ensuring project integrity.